Transifex Native is a full end-to-end, cloud-based localization stack for moderns apps.
Transifex Native SDK: JavaScript i18n
A general purpose Javascript library for localizing web apps using Transifex Native.
Requires a Transifex Native Project Token.
Supported Node.js versions >= 14.x.x
Related packages:
Learn more about Transifex Native in the Transifex Developer Hub.
How it works
Step1: Create a Transifex Native project in Transifex.
Step2: Grab credentials.
Step3: Internationalize the code using the SDK.
Step4: Push source phrases using the @transifex/cli
tool.
Step5: Translate the app using over-the-air updates.
No translation files required.
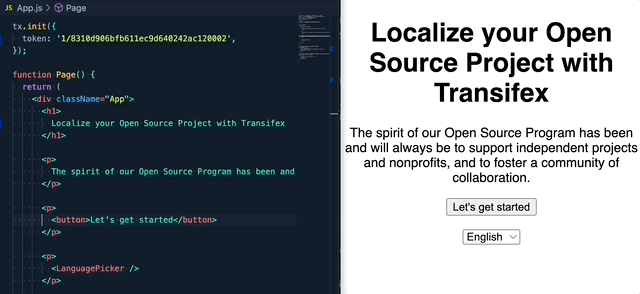
Upgrade to v2
If you are upgrading from the 1.x.x
version, please read this migration guide, as there are breaking changes in place.
Quick starting guide
Install the library using:
npm install @transifex/native --save
Webpack
import { tx, t } from '@transifex/native';
tx.init({
token: '<PUBLIC PROJECT TOKEN>',
});
async function main() {
await tx.setCurrentLocale('el');
const message = t('Welcome {user}', {user: 'Joe'});
console.log(message);
const languages = await tx.getLanguages();
console.log(languages);
}
main();
Node.js
const { tx, t } = require('@transifex/native');
tx.init({
token: '<PUBLIC PROJECT TOKEN>',
});
...
Browser
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/@transifex/native/dist/browser.native.min.js"></script>
<script type="text/javascript">
const tx = Transifex.tx;
const t = Transifex.t;
tx.init({
token: '<PUBLIC PROJECT TOKEN>',
});
tx.setCurrentLocale('fr').then(function() {
const message = t('Welcome {user}', {user: 'Joe'});
console.log(message);
});
</script>
API
Initialize library
tx.init({
token: String,
cdsHost: String,
filterTags: String,
filterStatus: String,
missingPolicy: Function,
errorPolicy: Function,
stringRenderer: Function,
cache: Function,
fetchTimeout: Number,
fetchInterval: Number,
})
Languages
Fetches list of project languages from CDS, useful for creating a language picker.
tx.getLanguages(): Promise([
{
name: String,
code: String,
localized_name: String,
rtl: Boolean
},
...
])
tx.getLanguages().
then(languages => console.log(languages)).
catch(err => console.log(err))
Get a list of available locales based on CDS.
tx.getLocales(): Promise(['code', 'code',...])
Set current translation language
Fetches translations from the CDS and stores them in cache. When the
promise returns, all content will be translated to that language.
tx.setCurrentLocale(localeCode): Promise
tx.setCurrentLocale('el').
then(() => console.log('content loaded')).
catch(err => console.log(err))
Get current translation language
Returns the currently selected language code.
tx.getCurrentLocale(): String(localeCode)
console.log(tx.getCurrentLocale())
Content translation
Returns the translation of the passed source string based on the
currenly selected language. If the translation is not found, the returned
string is handled by the configured missing policy. If an error occurs in the
ICU parsing of the string, the error is handled based on the configured error policy.
The translation is returned unescaped and it is NOT safe to be used inside the HTML document unless escaped
t(sourceString, params): String(localizedString)
sourceString: String(ICU syntax string)
params: Object({
_context: String,
_comment: String,
_charlimit: Number,
_tags: String,
_key: String,
_escapeVars: Boolean,
...icu variables...
})
console.log(
t('Hello <b>{username}</b>', { username: 'Joe' })
)
Escaping translations
Using the translation as is from the t
function inside HTML is dangerous for
XSS attacks. The translation must be escaped based on two scenarios.
Escaping text translations
import { t, escape } from '@transifex/native';
const translation = escape(t('Hello {username}', { username }));
Escaping HTML source
HTML source content cannot be globally escaped. In that case, we can just escape
the ICU variables using the _escapeVars
parameter.
import { t } from '@transifex/native';
const html = t('<b>Hello {username}</b>', {
username: username,
_escapeVars: true,
});
Push source content
For server side integrations you can also push source content programmatically
without using the CLI.
tx.pushSource(payload, params): Promise
payload: Object({
key: {
string: String,
meta: {
context: String,
developer_comment: String,
character_limit: Number,
tags: Array(String),
occurrences: Array(String),
},
},
key: { .. }
})
params: Object({
purge: Boolean,
overrideTags: Boolean,
overrideOccurrences: Boolean,
noWait: Boolean,
})
For example:
const { createNativeInstance } = require('@transifex/native');
const tx = createNativeInstance({
token: 'token',
secret: 'secret',
});
await tx.pushSource({
'mykey': {
string: 'My string',
meta: {
context: 'content',
developer_comment: 'developer comment',
character_limit: 10,
tags: ['tag1', 'tag2'],
occurrences: ['file.jsx', 'file2.js'],
},
}
});
Invalidate CDS cache
Server side integrations can also invalidate the CDS cache programmatically.
tx.invalidateCDS({
purge: Boolean,
}): Promise
For example:
const { createNativeInstance } = require('@transifex/native');
const tx = createNativeInstance({
token: 'token',
secret: 'secret',
});
await tx.invalidateCDS();
Events
Library for listening to various async events.
onEvent(type, function)
type:
FETCHING_TRANSLATIONS
TRANSLATIONS_FETCHED
TRANSLATIONS_FETCH_FAILED
LOCALE_CHANGED
FETCHING_LOCALES
LOCALES_FETCHED
LOCALES_FETCH_FAILED
offEvent(type, function)
sendEvent(type, payload, caller)
Using more than one TX Native instances
const { tx, t, createNativeInstance } = require('@transifex/native');
const txOtherInstance = createNativeInstance();
txOtherInstance.init({
token: '<PUBLIC PROJECT TOKEN 2>',
})
tx.init({
token: '<PUBLIC PROJECT TOKEN>',
});
tx.controllerOf(txOtherInstance);
tx.setCurrentLocale('fr').then(function() {
const message = t('Welcome {user}', {user: 'Joe'});
console.log(message);
const message2 = txOtherInstance.t('Welcome {user}', {user: 'Joe'});
console.log(message2);
});
License
Licensed under Apache License 2.0, see LICENSE file.