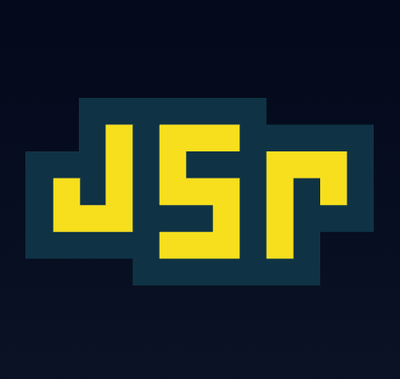
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@trivago/prettier-plugin-sort-imports
Advanced tools
A prettier plugins to sort imports in provided RegEx order
@trivago/prettier-plugin-sort-imports is a Prettier plugin that automatically sorts your import statements in a consistent and customizable manner. It helps maintain a clean and organized codebase by ensuring that import statements are ordered according to specified rules.
Sort imports alphabetically
This feature sorts the import statements alphabetically by module name.
import React from 'react';
import { useState } from 'react';
import { render } from 'react-dom';
Group imports by type
This feature groups import statements by their type, such as external libraries, internal modules, and styles.
import React from 'react';
import { useState } from 'react';
import { render } from 'react-dom';
import './styles.css';
import utils from './utils';
Customizable sorting order
This feature allows you to define a custom sorting order for your import statements, such as placing React imports at the top, followed by other external libraries, and then internal modules.
import React from 'react';
import { useState } from 'react';
import { render } from 'react-dom';
import utils from './utils';
import './styles.css';
eslint-plugin-import is an ESLint plugin that helps validate proper imports and enforce import order. It provides a wide range of rules for managing import statements, including sorting them. Unlike @trivago/prettier-plugin-sort-imports, which is a Prettier plugin, eslint-plugin-import is used with ESLint.
import-sort is a tool and library for sorting ES6 imports. It can be used as a standalone tool or integrated with various editors and build tools. It offers customizable sorting configurations similar to @trivago/prettier-plugin-sort-imports but is not tied to Prettier.
prettier-plugin-organize-imports is a Prettier plugin that uses the TypeScript language service to organize imports. It automatically removes unused imports and sorts the remaining ones. It is similar to @trivago/prettier-plugin-sort-imports but focuses on TypeScript projects.
A prettier plugin to sort import declarations by provided Regular Expression order.
Note: If you are migrating from v2.x.x to v3.x.x, Please Read Migration Guidelines
import React, {
FC,
useEffect,
useRef,
ChangeEvent,
KeyboardEvent,
} from 'react';
import { logger } from '@core/logger';
import { reduce, debounce } from 'lodash';
import { Message } from '../Message';
import { createServer } from '@server/node';
import { Alert } from '@ui/Alert';
import { repeat, filter, add } from '../utils';
import { initializeApp } from '@core/app';
import { Popup } from '@ui/Popup';
import { createConnection } from '@server/database';
import { debounce, reduce } from 'lodash';
import React, {
ChangeEvent,
FC,
KeyboardEvent,
useEffect,
useRef,
} from 'react';
import { createConnection } from '@server/database';
import { createServer } from '@server/node';
import { initializeApp } from '@core/app';
import { logger } from '@core/logger';
import { Alert } from '@ui/Alert';
import { Popup } from '@ui/Popup';
import { Message } from '../Message';
import { add, filter, repeat } from '../utils';
npm
npm install --save-dev @trivago/prettier-plugin-sort-imports
or, using yarn
yarn add --dev @trivago/prettier-plugin-sort-imports
Note: If you are migrating from v2.x.x to v3.x.x, Please Read Migration Guidelines
Note: If formatting .vue
sfc files please install @vue/compiler-sfc
if not in your dependency tree - this normally is within Vue projects.
Add an order in prettier config file.
module.exports = {
"printWidth": 80,
"tabWidth": 4,
"trailingComma": "all",
"singleQuote": true,
"semi": true,
"importOrder": ["^@core/(.*)$", "^@server/(.*)$", "^@ui/(.*)$", "^[./]"],
"importOrderSeparation": true,
"importOrderSortSpecifiers": true
}
importOrder
type: Array<string>
A collection of Regular expressions in string format.
"importOrder": ["^@core/(.*)$", "^@server/(.*)$", "^@ui/(.*)$", "^[./]"],
Default behavior: The plugin moves the third party imports to the top which are not part of the importOrder
list.
To move the third party imports at desired place, you can use <THIRD_PARTY_MODULES>
to assign third party imports to the appropriate position:
"importOrder": ["^@core/(.*)$", "<THIRD_PARTY_MODULES>", "^@server/(.*)$", "^@ui/(.*)$", "^[./]"],
importOrderSeparation
type: boolean
default value: false
A boolean value to enable or disable the new line separation
between sorted import declarations group. The separation takes place according to the importOrder
.
"importOrderSeparation": true,
importOrderSortSpecifiers
type: boolean
default value: false
A boolean value to enable or disable sorting of the specifiers in an import declarations.
importOrderGroupNamespaceSpecifiers
type: boolean
default value: false
A boolean value to enable or disable sorting the namespace specifiers to the top of the import group.
importOrderCaseInsensitive
type: boolean
default value: false
A boolean value to enable case-insensitivity in the sorting algorithm used to order imports within each match group.
For example, when false (or not specified):
import ExampleView from './ExampleView';
import ExamplesList from './ExamplesList';
compared with "importOrderCaseInsensitive": true
:
import ExamplesList from './ExamplesList';
import ExampleView from './ExampleView';
importOrderParserPlugins
type: Array<string>
default value: ["typescript", "jsx"]
Previously known as experimentalBabelParserPluginsList
.
A collection of plugins for babel parser. The plugin passes this list to babel parser, so it can understand the syntaxes used in the file being formatted. The plugin uses prettier itself to figure out the parser it needs to use but if that fails, you can use this field to enforce the usage of the plugins' babel parser needs.
To pass the plugins to babel parser:
"importOrderParserPlugins" : ["classProperties", "decorators-legacy"]
To pass the options to the babel parser plugins: Since prettier options are limited to string, you can pass plugins
with options as a JSON string of the plugin array:
"[\"plugin-name\", { \"pluginOption\": true }]"
.
"importOrderParserPlugins" : ["classProperties", "[\"decorators\", { \"decoratorsBeforeExport\": true }]"]
To disable default plugins for babel parser, pass an empty array:
importOrderParserPlugins: []
The plugin extracts the imports which are defined in importOrder
. These imports are considered as local imports.
The imports which are not part of the importOrder
is considered as third party imports.
After, the plugin sorts the local imports and third party imports using natural sort algorithm.
In the end, the plugin returns final imports with third party imports on top and local imports at the end.
The third party imports position (it's top by default) can be overridden using the <THIRD_PARTY_MODULES>
special word in the importOrder
.
Having some trouble or an issue ? You can check FAQ / Troubleshooting section.
Framework | Supported | Note |
---|---|---|
JS with ES Modules | ✅ Everything | - |
NodeJS with ES Modules | ✅ Everything | - |
React | ✅ Everything | - |
Angular | ✅ Everything | Supported through importOrderParserPlugins API |
Vue | ✅ Everything | @vue/compiler-sfc is required |
Svelte | ⚠️ Soon to be supported. | Any contribution is welcome. |
Want to highlight your project or company ? Adding your project / company name will help plugin to gain attraction and contribution. Feel free to make a Pull Request to add your project / company name.
For more information regarding contribution, please check the Contributing Guidelines. If you are trying to debug some code in the plugin, check Debugging Guidelines
Ayush Sharma | Behrang Yarahmadi |
---|---|
@ayusharma_ | @behrang_y |
This plugin modifies the AST which is against the rules of prettier.
v4.1.0
FAQs
A prettier plugins to sort imports in provided RegEx order
The npm package @trivago/prettier-plugin-sort-imports receives a total of 684,651 weekly downloads. As such, @trivago/prettier-plugin-sort-imports popularity was classified as popular.
We found that @trivago/prettier-plugin-sort-imports demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.