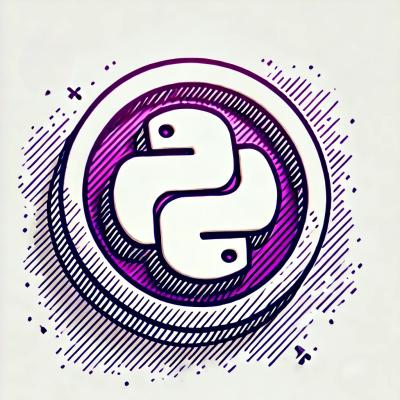
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@trpc/client
Advanced tools
@trpc/client is a TypeScript-first framework for building end-to-end typesafe APIs. It allows you to create and consume APIs with full type safety, ensuring that your client and server are always in sync.
Creating a TRPC Client
This code demonstrates how to create a TRPC client that connects to a TRPC server running at 'http://localhost:4000/trpc'.
const trpc = require('@trpc/client');
const { createTRPCClient } = trpc;
const client = createTRPCClient({
url: 'http://localhost:4000/trpc',
});
Making a Query
This code demonstrates how to make a query to the TRPC server to fetch a user with a specific ID.
const result = await client.query('getUser', { id: 1 });
console.log(result);
Making a Mutation
This code demonstrates how to make a mutation to the TRPC server to create a new user.
const newUser = await client.mutation('createUser', { name: 'John Doe' });
console.log(newUser);
Subscribing to a Subscription
This code demonstrates how to subscribe to a subscription on the TRPC server to listen for updates to a specific user.
const subscription = client.subscription('onUserUpdate', { userId: 1 }, {
onData: (data) => console.log('User updated:', data),
onError: (err) => console.error('Subscription error:', err),
});
Apollo Client is a comprehensive state management library for JavaScript that enables you to manage both local and remote data with GraphQL. Unlike @trpc/client, which is TypeScript-first and focuses on end-to-end type safety, Apollo Client is centered around GraphQL and offers a rich ecosystem for managing data fetching, caching, and state management.
Axios is a promise-based HTTP client for the browser and Node.js. While it does not offer the same level of type safety and end-to-end integration as @trpc/client, it is a versatile and widely-used library for making HTTP requests.
React Query is a data-fetching library for React applications that simplifies fetching, caching, and synchronizing server data. It can be used with any data-fetching library, including @trpc/client, to manage server state in a React application. Unlike @trpc/client, React Query is not focused on type safety but rather on providing a powerful and flexible data-fetching solution.
End-to-end typesafe APIs made easy
@trpc/client
Communicate with a tRPC server on the client side.
Full documentation for @trpc/client
can be found here
# npm
npm install @trpc/client@next
# Yarn
yarn add @trpc/client@next
# pnpm
pnpm add @trpc/client@next
# Bun
bun add @trpc/client@next
import { createTRPCClient, httpBatchLink } from '@trpc/client';
// Importing the router type from the server file
import type { AppRouter } from './server';
// Initializing the tRPC client
const trpc = createTRPCClient<AppRouter>({
links: [
httpBatchLink({
url: 'http://localhost:3000/trpc',
}),
],
});
async function main() {
// Querying the greeting
const helloResponse = await trpc.greeting.query({
name: 'world',
});
console.log('helloResponse', helloResponse); // Hello world
}
main();
FAQs
The tRPC client library
The npm package @trpc/client receives a total of 425,508 weekly downloads. As such, @trpc/client popularity was classified as popular.
We found that @trpc/client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.