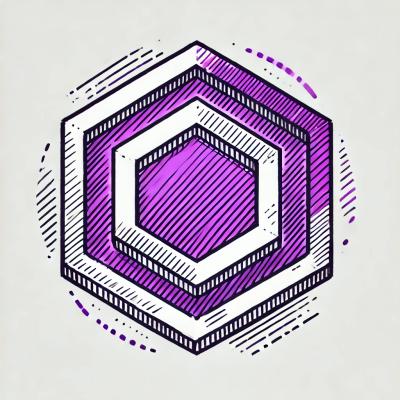
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
@trpc/next
Advanced tools
@trpc/next is a package that allows you to create end-to-end typesafe APIs using tRPC in a Next.js application. It simplifies the process of building and consuming APIs by leveraging TypeScript for type safety and Next.js for server-side rendering and API routes.
Creating a tRPC Router
This feature allows you to create a tRPC router with various procedures. In this example, a simple 'hello' query is defined that returns 'Hello world'.
import { initTRPC } from '@trpc/server';
const t = initTRPC.create();
const appRouter = t.router({
hello: t.procedure.query(() => 'Hello world'),
});
export type AppRouter = typeof appRouter;
Integrating tRPC with Next.js API Routes
This feature demonstrates how to integrate a tRPC router with Next.js API routes. The `createNextApiHandler` function is used to create an API handler that can be used in a Next.js API route.
import { createNextApiHandler } from '@trpc/server/adapters/next';
import { appRouter } from 'path/to/your/router';
export default createNextApiHandler({
router: appRouter,
createContext: () => null,
});
Using tRPC in Next.js Pages
This feature shows how to use tRPC in a Next.js page. The `trpc.hello.useQuery` hook is used to fetch data from the 'hello' query defined in the tRPC router.
import { trpc } from 'path/to/trpc';
const HomePage = () => {
const { data, error, isLoading } = trpc.hello.useQuery();
if (isLoading) return <div>Loading...</div>;
if (error) return <div>Error: {error.message}</div>;
return <div>{data}</div>;
};
export default HomePage;
next-api-decorators is a package that provides a set of decorators to create type-safe API routes in Next.js. It uses TypeScript decorators to define API routes and handlers, offering a similar level of type safety as @trpc/next but with a different approach.
Blitz is a full-stack React framework built on top of Next.js. It includes a built-in data layer that provides a type-safe API similar to tRPC. Blitz aims to be a 'batteries-included' framework, offering more out-of-the-box features compared to @trpc/next.
GraphQL is a query language for APIs and a runtime for executing those queries. While it is not specific to Next.js, it can be integrated with Next.js to create type-safe APIs. GraphQL offers more flexibility in querying data compared to tRPC but requires more setup and boilerplate.
End-to-end typesafe APIs made easy
@trpc/next
Connect a tRPC router to Next.js.
Full documentation for @trpc/next
can be found here
# npm
npm install @trpc/next @trpc/react-query @tanstack/react-query@4
# Yarn
yarn add @trpc/next @trpc/react-query @tanstack/react-query@4
# pnpm
pnpm add @trpc/next @trpc/react-query @tanstack/react-query@4
# Bun
bun add @trpc/next @trpc/react-query @tanstack/react-query@4
Setup tRPC in utils/trpc.ts
.
import { createTRPCNext, httpBatchLink } from '@trpc/next';
// Import the router type from your server file
import type { AppRouter } from '../pages/api/[trpc].ts';
export const trpc = createTRPCNext<AppRouter>({
config() {
return {
links: [
httpBatchLink({
url: 'http://localhost:3000/trpc',
}),
],
};
},
ssr: true,
});
Hook up tRPC inside _app.tsx
.
import { trpc } from '~/utils/trpc';
const App = ({ Component, pageProps }) => {
return <Component {...pageProps} />;
};
export default trpc.withTRPC(App);
Now you can query your API in any component.
import { trpc } from '~/utils/trpc';
export function Hello() {
const { data, error, status } = trpc.greeting.useQuery({
name: 'tRPC',
});
if (error) {
return <p>{error.message}</p>;
}
if (status !== 'success') {
return <p>Loading...</p>;
}
return <div>{data && <p>{data.greeting}</p>}</div>;
}
FAQs
The tRPC Next.js library
The npm package @trpc/next receives a total of 222,051 weekly downloads. As such, @trpc/next popularity was classified as popular.
We found that @trpc/next demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.