@trycourier/courier
Advanced tools
Comparing version 3.5.0 to 3.6.0
@@ -8,2 +8,6 @@ # Change Log | ||
## [v3.6.0] - 2022-02-10 | ||
- adds additional type's for the recipient property (`message.to`) | ||
## [v3.5.0] - 2022-02-10 | ||
@@ -10,0 +14,0 @@ |
@@ -169,6 +169,2 @@ export interface IBrandSnippet { | ||
} | ||
interface ListRecipient { | ||
list_id: string; | ||
pattern?: string; | ||
} | ||
export declare type RuleType = "snooze" | "channel_preferences" | "status"; | ||
@@ -201,4 +197,33 @@ export interface IRule<T extends RuleType> { | ||
} | ||
export interface UserRecipient extends Record<string, any> { | ||
interface InvalidListRecipient { | ||
user_id: string; | ||
list_pattern: string; | ||
} | ||
declare type ListRecipientType = Record<string, unknown> & { | ||
[key in keyof InvalidListRecipient]?: never; | ||
}; | ||
export interface ListRecipient extends ListRecipientType { | ||
list_id?: string; | ||
data?: MessageData; | ||
} | ||
interface InvalidListPatternRecipient { | ||
user_id: string; | ||
list_id: string; | ||
} | ||
declare type ListPatternRecipientType = Record<string, unknown> & { | ||
[key in keyof InvalidListPatternRecipient]?: never; | ||
}; | ||
export interface ListPatternRecipient extends ListPatternRecipientType { | ||
list_pattern?: string; | ||
data?: MessageData; | ||
} | ||
interface InvalidUserRecipient { | ||
list_id: string; | ||
list_pattern: string; | ||
} | ||
declare type UserRecipientType = Record<string, unknown> & { | ||
[key in keyof InvalidUserRecipient]?: never; | ||
}; | ||
export interface UserRecipient extends UserRecipientType { | ||
data?: MessageData; | ||
email?: string; | ||
@@ -210,3 +235,4 @@ locale?: string; | ||
} | ||
export declare type MessageRecipient = ListRecipient | UserRecipient; | ||
export declare type Recipient = ListRecipient | ListPatternRecipient | UserRecipient; | ||
export declare type MessageRecipient = Recipient | Recipient[]; | ||
export interface ElementalContentSugar { | ||
@@ -218,3 +244,3 @@ body?: string; | ||
export interface BaseMessage { | ||
to: MessageRecipient | MessageRecipient[]; | ||
to: MessageRecipient; | ||
data?: MessageData; | ||
@@ -221,0 +247,0 @@ channels?: MessageChannels; |
{ | ||
"name": "@trycourier/courier", | ||
"version": "3.5.0", | ||
"version": "3.6.0", | ||
"description": "A node.js module for communicating with the Courier REST API.", | ||
@@ -5,0 +5,0 @@ "main": "lib/index.js", |
182
README.md
@@ -26,26 +26,139 @@ [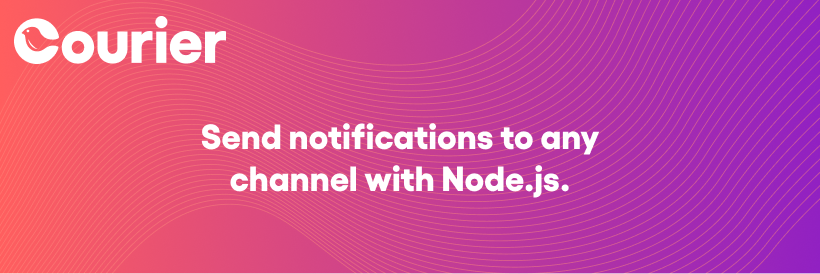](https://courier.com) | ||
// Example: send a basic message to an email recipient | ||
const { requestId } = await courier.send({ | ||
message: { | ||
to: { | ||
data: { | ||
name: "Marty", | ||
}, | ||
email: "marty_mcfly@email.com", | ||
}, | ||
content: { | ||
title: "Back to the Future", | ||
body: "Oh my {{name}}, we need 1.21 Gigawatts!", | ||
}, | ||
routing: { | ||
method: "single", | ||
channels: ["email"], | ||
}, | ||
}, | ||
}); | ||
// Example: send a basic message to an sms recipient | ||
const { requestId } = await courier.send({ | ||
message: { | ||
to: { | ||
data: { | ||
name: "Jenny", | ||
}, | ||
phone_number: "8675309", | ||
}, | ||
content: { | ||
title: "Back to the Future", | ||
body: "Oh my {{name}}, we need 1.21 Gigawatts!", | ||
}, | ||
routing: { | ||
method: "single", | ||
channels: ["sms"], | ||
}, | ||
}, | ||
}); | ||
// Example: send a message to various recipients | ||
const { requestId } = await courier.send({ | ||
message: { | ||
to: [ | ||
{ | ||
user_id: "<USER_ID>", // usually your system's User ID associated to a Courier profile | ||
email: "test@email.com", | ||
data: { | ||
name: "some user's name", | ||
}, | ||
}, | ||
{ | ||
email: "marty@email.com", | ||
data: { | ||
name: "Marty", | ||
}, | ||
}, | ||
{ | ||
email: "doc_brown@email.com", | ||
data: { | ||
name: "Doc", | ||
}, | ||
}, | ||
{ | ||
phone_number: "8675309", | ||
data: { | ||
name: "Jenny", | ||
}, | ||
}, | ||
], | ||
content: { | ||
title: "Back to the Future", | ||
body: "Oh my {{name}}, we need 1.21 Gigawatts!", | ||
}, | ||
routing: { | ||
method: "all", | ||
channels: ["sms", "email"], | ||
}, | ||
}, | ||
}); | ||
// Example: send a message supporting email & SMS | ||
const { messageId } = await courier.send({ | ||
eventId: "<EVENT_ID>", // get from the Courier UI | ||
recipientId: "<RECIPIENT_ID>", // usually your system's User ID | ||
profile: { | ||
email: "example@example.com", | ||
phone_number: "555-228-3890", | ||
const { requestId } = await courier.send({ | ||
message: { | ||
template: "<TEMPLATE_OR_EVENT_ID>", // get from the Courier UI | ||
to: { | ||
user_Id: "<USER_ID>", // usually your system's User ID | ||
email: "example@example.com", | ||
phone_number: "555-228-3890", | ||
}, | ||
data: {}, // optional variables for merging into templates | ||
}, | ||
data: {}, // optional variables for merging into templates | ||
}); | ||
// Example: send a message to a list | ||
const { messageId } = await courier.lists.send({ | ||
event: "<EVENT_ID>", // get from the Courier UI | ||
list: "<LIST_ID>", // e.g. example.list.id | ||
data: {}, // optional variables for merging into templates | ||
const { requestId } = await courier.send({ | ||
message: { | ||
template: "<TEMPLATE_OR_EVENT_ID>", // get from the Courier UI | ||
to: { | ||
list_id: "<LIST_ID>", // e.g. your Courier List Id | ||
}, | ||
data: {}, // optional variables for merging into templates | ||
}, | ||
}); | ||
// Example: send a message to a pattern | ||
const { messageId } = await courier.lists.send({ | ||
event: "<EVENT_ID>", // get from the Courier UI | ||
pattern: "<PATTERN>", // e.g. example.list.* | ||
data: {}, // optional variables for merging into templates | ||
const { requestId } = await courier.send({ | ||
message: { | ||
template: "<TEMPLATE_OR_EVENT_ID>", // get from the Courier UI | ||
to: { | ||
list_pattern: "<PATTERN>", // e.g. example.list.* | ||
}, | ||
data: {}, // optional variables for merging into templates | ||
}, | ||
}); | ||
// Example: send a message to a list, pattern and user | ||
const { requestId } = await courier.send({ | ||
message: { | ||
to: [ | ||
{ | ||
list_pattern: "<PATTERN>", // e.g. example.list.* | ||
}, | ||
{ | ||
list_id: "<LIST_ID>", // e.g. your Courier List Id | ||
}, | ||
{ | ||
email: "test@email.com" | ||
} | ||
] | ||
}, | ||
routing: { | ||
method: "single", | ||
channels: ["email"], | ||
}, | ||
}, | ||
}); | ||
``` | ||
@@ -69,23 +182,28 @@ | ||
// Example: send a message | ||
const { messageId } = await courier.send({ | ||
eventId: "<EVENT_ID>", | ||
recipientId: "<RECIPIENT_ID>", | ||
profile: {}, // optional | ||
data: {}, // optional | ||
brand: "<BRAND_ID>", //optional | ||
preferences: {}, // optional | ||
override: {}, // optional | ||
const { requestId } = await courier.send({ | ||
message: { | ||
template: "<TEMPLATE_OR_EVENT_ID>", | ||
to: { | ||
// optional | ||
user_id: "<RECIPIENT_ID>", | ||
}, | ||
data: {}, // optional | ||
brand_id: "<BRAND_ID>", //optional | ||
routing: {}, | ||
channels: {}, // optional | ||
providers: {}, // optional | ||
}, | ||
}); | ||
console.log(messageId); | ||
console.log(requestId); | ||
// Example: get a message status | ||
const messageStatus = await courier.getMessage(messageId); | ||
const messageStatus = await courier.getMessage(requestId); | ||
console.log(messageStatus); | ||
// Example: get a message history | ||
const { results } = await courier.getMessageHistory(messageId); | ||
const { results } = await courier.getMessageHistory(requestId); | ||
console.log(results); | ||
// Example: get a message output | ||
const { results } = await courier.getMessageOutput(messageId); | ||
const { results } = await courier.getMessageOutput(requestId); | ||
console.log(results); | ||
@@ -438,7 +556,7 @@ | ||
async function run() { | ||
const { messageId } = await courier.send( | ||
const { requestId } = await courier.send( | ||
{ | ||
eventId: "<EVENT_ID>", | ||
recipientId: "<RECIPIENT_ID>", | ||
profile: { | ||
template: "<TEMPLATE_OR_EVENT_ID>", | ||
to: { | ||
user_id: "<USER_ID>", | ||
email: "example@example.com", | ||
@@ -455,3 +573,3 @@ phone_number: "555-867-5309", | ||
); | ||
console.log(messageId); | ||
console.log(requestId); | ||
} | ||
@@ -458,0 +576,0 @@ |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
120881
2294
583