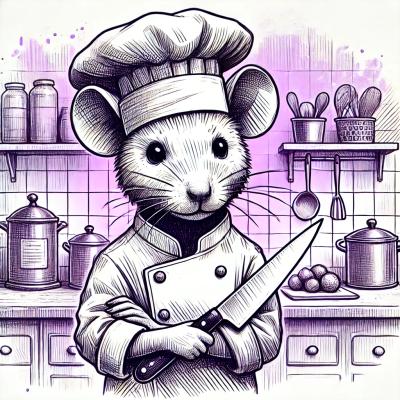
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@turf/flip
Advanced tools
@turf/flip is a module in the Turf.js library that allows you to flip the coordinates of GeoJSON objects. This is useful when you need to switch the order of latitude and longitude coordinates.
Flip Coordinates of a Point
This feature allows you to flip the coordinates of a GeoJSON Point object. The code sample demonstrates how to flip the coordinates of a point from [120.0, 30.0] to [30.0, 120.0].
const turf = require('@turf/turf');
const point = turf.point([120.0, 30.0]);
const flippedPoint = turf.flip(point);
console.log(flippedPoint);
Flip Coordinates of a LineString
This feature allows you to flip the coordinates of a GeoJSON LineString object. The code sample demonstrates how to flip the coordinates of a line from [[120.0, 30.0], [121.0, 31.0]] to [[30.0, 120.0], [31.0, 121.0]].
const turf = require('@turf/turf');
const line = turf.lineString([[120.0, 30.0], [121.0, 31.0]]);
const flippedLine = turf.flip(line);
console.log(flippedLine);
Flip Coordinates of a Polygon
This feature allows you to flip the coordinates of a GeoJSON Polygon object. The code sample demonstrates how to flip the coordinates of a polygon from [[[120.0, 30.0], [121.0, 31.0], [122.0, 32.0], [120.0, 30.0]]] to [[[30.0, 120.0], [31.0, 121.0], [32.0, 122.0], [30.0, 120.0]]].
const turf = require('@turf/turf');
const polygon = turf.polygon([[[120.0, 30.0], [121.0, 31.0], [122.0, 32.0], [120.0, 30.0]]]);
const flippedPolygon = turf.flip(polygon);
console.log(flippedPolygon);
The geojson-rewind package is used to enforce the winding order of GeoJSON polygons. While it doesn't flip coordinates, it ensures that the coordinates follow the right-hand rule. This is different from @turf/flip, which specifically flips the order of latitude and longitude.
The geojson-normalize package is used to normalize various GeoJSON objects into a consistent format. While it doesn't flip coordinates, it ensures that the GeoJSON objects are in a standard format. This is different from @turf/flip, which specifically flips the order of latitude and longitude.
Takes input features and flips all of their coordinates from [x, y]
to [y, x]
.
geojson
GeoJSON input features
options
Object Optional parameters (optional, default {}
)
options.mutate
boolean allows GeoJSON input to be mutated (significant performance increase if true) (optional, default false
)var serbia = turf.point([20.566406, 43.421008]);
var saudiArabia = turf.flip(serbia);
//addToMap
var addToMap = [serbia, saudiArabia];
Returns GeoJSON a feature or set of features of the same type as input
with flipped coordinates
This module is part of the Turfjs project, an open source module collection dedicated to geographic algorithms. It is maintained in the Turfjs/turf repository, where you can create PRs and issues.
Install this single module individually:
$ npm install @turf/flip
Or install the all-encompassing @turf/turf module that includes all modules as functions:
$ npm install @turf/turf
FAQs
turf flip module
We found that @turf/flip demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.