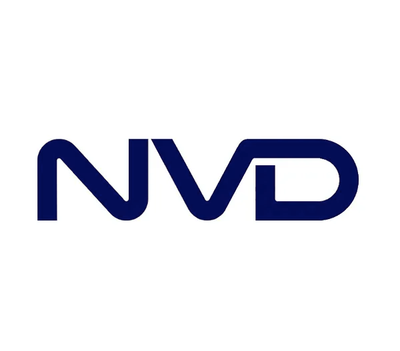
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
@typegoose/typegoose
Advanced tools
(These badges are from typegoose:master)
Define Mongoose models using TypeScript classes
Migration Guides:
(Date format: dd-mm-yyyy
)
22-09-2021
)28-07-2021
)01-04-2020
)30-09-2019
)import { prop, getModelForClass } from '@typegoose/typegoose';
import * as mongoose from 'mongoose';
class User {
@prop()
public name?: string;
@prop({ type: () => [String] })
public jobs?: string[];
}
const UserModel = getModelForClass(User); // UserModel is a regular Mongoose Model with correct types
(async () => {
await mongoose.connect('mongodb://localhost:27017/', { useNewUrlParser: true, useUnifiedTopology: true, dbName: 'test' });
const { _id: id } = await UserModel.create({ name: 'JohnDoe', jobs: ['Cleaner'] } as User); // an "as" assertion, to have types for all properties
const user = await UserModel.findById(id).exec();
console.log(user); // prints { _id: 59218f686409d670a97e53e0, name: 'JohnDoe', __v: 0 }
})();
A common problem when using Mongoose with TypeScript is that you have to define both the Mongoose model and the TypeScript interface. If the model changes, you also have to keep the TypeScript interface file in sync or the TypeScript interface would not represent the real data structure of the model.
Typegoose aims to solve this problem by defining only a TypeScript interface (class), which needs to be enhanced with special Typegoose decorators (like @prop
).
Under the hood it uses the Reflect & reflect-metadata API to retrieve the types of the properties, so redundancy can be significantly reduced.
Instead of writing this:
// This is a representation of how typegoose's compile output would look like
interface Car {
model?: string;
}
interface Job {
title?: string;
position?: string;
}
interface User {
name?: string;
age!: number;
preferences?: string[];
mainJob?: Job;
jobs?: Job[];
mainCar?: Car | string;
cars?: (Car | string)[];
}
const JobSchema = new mongoose.Schema({
title: String;
position: String;
});
const CarModel = mongoose.model('Car', {
model: string,
});
const UserModel = mongoose.model('User', {
name: { type: String },
age: { type: Number, required: true },
preferences: [{ type: String }],
mainJob: { type: JobSchema },
jobs: [{ type: JobSchema }],
mainCar: { type: Schema.Types.ObjectId, ref: 'Car' },
cars: [{ type: Schema.Types.ObjectId, ref: 'Car' }],
});
You can just write this:
class Job {
@prop()
public title?: string;
@prop()
public position?: string;
}
class Car {
@prop()
public model?: string;
}
class User {
@prop()
public name?: string;
@prop({ required: true })
public age!: number; // This is a single Primitive
@prop({ type: () => [String] })
public preferences?: string[]; // This is a Primitive Array
@prop()
public mainJob?: Job; // This is a single SubDocument
@prop({ type: () => Job })
public jobs?: Job[]; // This is a SubDocument Array
@prop({ ref: () => Car })
public mainCar?: Ref<Car>; // This is a single Reference
@prop({ ref: () => Car })
public cars?: Ref<Car>[]; // This is a Reference Array
}
yarn install
yarn run test
This Project should comply with Semver. It uses the Major.Minor.Fix
standard (or in NPM terms, Major.Minor.Patch
).
To ask questions or just talk with us, join our Discord Server.
+1
or similar comments to issues. Use the reactions instead.10.0.0-beta.1 (2022-12-01)
hooks: dont define empty hooks options if not provided (89b9416)
hooks: use mongoose's array looping over methods over typegoose's (ca2a03a), closes typegoose/typegoose#587
utils::initProperty: simplify paths (37ca83e)
update minimal NodeJS version to 14.0.0 (fcffbd8)
FAQs
Define Mongoose models using TypeScript classes
The npm package @typegoose/typegoose receives a total of 85,010 weekly downloads. As such, @typegoose/typegoose popularity was classified as popular.
We found that @typegoose/typegoose demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.