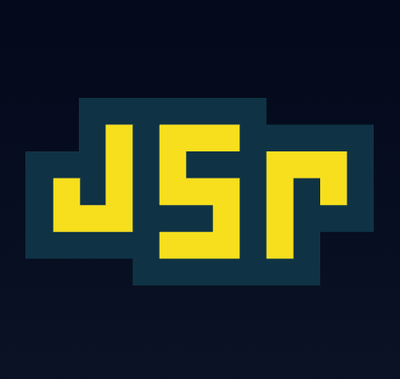
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@typegoose/typegoose
Advanced tools
(These badges are from typegoose:master)
Define Mongoose models using TypeScript classes
Migration Guides:
(Date format: dd-mm-yyyy
)
25-11-2023
)27-03-2023
)12-12-2022
)22-09-2021
)28-07-2021
)01-04-2020
)30-09-2019
)import { prop, getModelForClass } from '@typegoose/typegoose';
import * as mongoose from 'mongoose';
class User {
@prop()
public name?: string;
@prop({ type: () => [String] })
public jobs?: string[];
}
const UserModel = getModelForClass(User); // UserModel is a regular Mongoose Model with correct types
(async () => {
await mongoose.connect('mongodb://localhost:27017/', { dbName: 'test' });
const { _id: id } = await UserModel.create({ name: 'JohnDoe', jobs: ['Cleaner'] });
const user = await UserModel.findById(id).exec();
console.log(user); // prints { _id: 59218f686409d670a97e53e0, name: 'JohnDoe', __v: 0 }
})();
A common problem when using Mongoose with TypeScript is that you have to define both the Mongoose model and the TypeScript interface. If the model changes, you also have to keep the TypeScript interface file in sync or the TypeScript interface would not represent the real data structure of the model.
Typegoose aims to solve this problem by defining only a TypeScript interface (class), which needs to be enhanced with special Typegoose decorators (like @prop
).
Under the hood it uses the Reflect & reflect-metadata API to retrieve the types of the properties, so redundancy can be significantly reduced.
Instead of writing this:
// This is a representation of how typegoose's compile output would look like
interface Car {
model?: string;
}
interface Job {
title?: string;
position?: string;
}
interface User {
name?: string;
age!: number;
preferences?: string[];
mainJob?: Job;
jobs?: Job[];
mainCar?: Car | string;
cars?: (Car | string)[];
}
const JobSchema = new mongoose.Schema({
title: String;
position: String;
});
const CarModel = mongoose.model('Car', {
model: string,
});
const UserModel = mongoose.model('User', {
name: { type: String },
age: { type: Number, required: true },
preferences: [{ type: String }],
mainJob: { type: JobSchema },
jobs: [{ type: JobSchema }],
mainCar: { type: Schema.Types.ObjectId, ref: 'Car' },
cars: [{ type: Schema.Types.ObjectId, ref: 'Car' }],
});
You can just write this:
class Job {
@prop()
public title?: string;
@prop()
public position?: string;
}
class Car {
@prop()
public model?: string;
}
class User {
@prop()
public name?: string;
@prop({ required: true })
public age!: number; // This is a single Primitive
@prop({ type: () => [String] })
public preferences?: string[]; // This is a Primitive Array
@prop()
public mainJob?: Job; // This is a single SubDocument
@prop({ type: () => Job })
public jobs?: Job[]; // This is a SubDocument Array
@prop({ ref: () => Car })
public mainCar?: Ref<Car>; // This is a single Reference
@prop({ ref: () => Car })
public cars?: Ref<Car>[]; // This is a Reference Array
}
yarn install
yarn run test
This Project should comply with Semver. It uses the Major.Minor.Fix
standard (or in NPM terms, Major.Minor.Patch
).
To ask questions or just talk with us, join our Discord Server.
+1
or similar comments to issues. Use the reactions instead.FAQs
Define Mongoose models using TypeScript classes
The npm package @typegoose/typegoose receives a total of 68,544 weekly downloads. As such, @typegoose/typegoose popularity was classified as popular.
We found that @typegoose/typegoose demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.