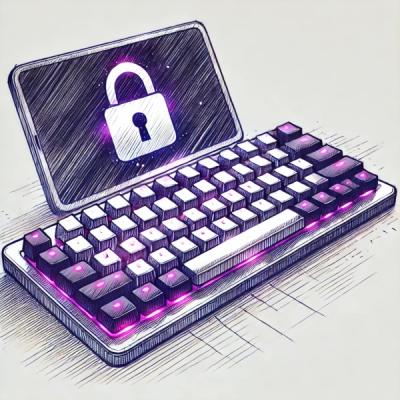
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
@types/amqplib
Advanced tools
Package description
@types/amqplib provides TypeScript definitions for the amqplib library, which is a client for RabbitMQ, a popular message broker. This package allows TypeScript developers to use amqplib with type safety, ensuring that the code adheres to the expected types and interfaces.
Connecting to RabbitMQ
This feature demonstrates how to establish a connection to a RabbitMQ server using amqplib. The code connects to a RabbitMQ server running on localhost and then closes the connection.
const amqp = require('amqplib');
async function connect() {
try {
const connection = await amqp.connect('amqp://localhost');
console.log('Connected to RabbitMQ');
await connection.close();
} catch (error) {
console.error('Failed to connect to RabbitMQ', error);
}
}
connect();
Creating a Channel
This feature demonstrates how to create a channel after establishing a connection to RabbitMQ. Channels are used to perform most of the operations in RabbitMQ.
const amqp = require('amqplib');
async function createChannel() {
try {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
console.log('Channel created');
await channel.close();
await connection.close();
} catch (error) {
console.error('Failed to create channel', error);
}
}
createChannel();
Sending a Message
This feature demonstrates how to send a message to a queue in RabbitMQ. The code creates a connection and a channel, asserts a queue, and sends a message to that queue.
const amqp = require('amqplib');
async function sendMessage() {
try {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'test_queue';
const message = 'Hello, RabbitMQ!';
await channel.assertQueue(queue);
channel.sendToQueue(queue, Buffer.from(message));
console.log(`Message sent: ${message}`);
await channel.close();
await connection.close();
} catch (error) {
console.error('Failed to send message', error);
}
}
sendMessage();
Receiving a Message
This feature demonstrates how to receive messages from a queue in RabbitMQ. The code creates a connection and a channel, asserts a queue, and consumes messages from that queue.
const amqp = require('amqplib');
async function receiveMessage() {
try {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'test_queue';
await channel.assertQueue(queue);
console.log(`Waiting for messages in ${queue}`);
channel.consume(queue, (msg) => {
if (msg !== null) {
console.log(`Received message: ${msg.content.toString()}`);
channel.ack(msg);
}
});
} catch (error) {
console.error('Failed to receive message', error);
}
}
receiveMessage();
Rhea is a high-level AMQP 1.0 client for Node.js. It provides a more modern and flexible API compared to amqplib, which is based on AMQP 0.9.1. Rhea supports advanced features like message routing and filtering.
amqp-connection-manager is a wrapper around amqplib that provides automatic reconnection and connection pooling. It simplifies the management of RabbitMQ connections and channels, making it easier to build robust applications.
Rascal is a configuration-driven library for working with RabbitMQ. It builds on top of amqplib and provides features like message retry, dead-letter exchanges, and more. Rascal is designed to simplify the setup and management of RabbitMQ resources.
Readme
npm install --save @types/amqplib
This package contains type definitions for amqplib (https://github.com/squaremo/amqp.node).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/amqplib
Additional Details
These definitions were written by Michael Nahkies https://github.com/mnahkies, Ab Reitsma https://github.com/abreits, Nicolás Fantone https://github.com/nfantone, and Nick Zelei https://github.com/zelein.
FAQs
Unknown package
The npm package @types/amqplib receives a total of 288,501 weekly downloads. As such, @types/amqplib popularity was classified as popular.
We found that @types/amqplib demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.