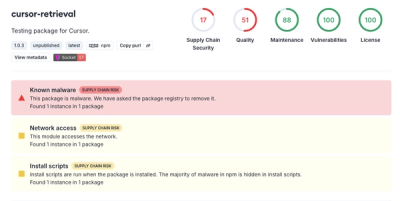
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@types/backbone
Advanced tools
@types/backbone provides TypeScript type definitions for the Backbone.js library, which is a framework that gives structure to web applications by providing models with key-value binding and custom events, collections with a rich API of enumerable functions, views with declarative event handling, and connects it all to your existing API over a RESTful JSON interface.
Models
Backbone models are the heart of any JavaScript application, containing the interactive data as well as a large part of the logic surrounding it. The code sample demonstrates how to define a model with default attributes and how to create an instance of that model.
const MyModel = Backbone.Model.extend({
defaults: {
name: 'Default Name',
age: 0
}
});
const modelInstance = new MyModel();
console.log(modelInstance.get('name')); // Output: Default Name
Collections
Collections are ordered sets of models. The code sample shows how to define a collection that contains a specific model type and how to create an instance of that collection with initial data.
const MyModel = Backbone.Model.extend({});
const MyCollection = Backbone.Collection.extend({
model: MyModel
});
const collectionInstance = new MyCollection([{ id: 1 }, { id: 2 }]);
console.log(collectionInstance.length); // Output: 2
Views
Views are used to reflect the state of a model or collection in the user interface. The code sample demonstrates how to define a view with a render method and how to create an instance of that view and render it.
const MyView = Backbone.View.extend({
render: function() {
this.$el.html('Hello, World!');
return this;
}
});
const viewInstance = new MyView();
viewInstance.render();
console.log(viewInstance.el.innerHTML); // Output: Hello, World!
Routers
Routers provide methods for routing client-side pages and connecting them to actions and events. The code sample shows how to define a router with a route and how to navigate to that route programmatically.
const MyRouter = Backbone.Router.extend({
routes: {
'home': 'homeRoute'
},
homeRoute: function() {
console.log('Home route triggered');
}
});
const routerInstance = new MyRouter();
Backbone.history.start();
routerInstance.navigate('home', { trigger: true }); // Output: Home route triggered
@types/react provides TypeScript type definitions for React, a JavaScript library for building user interfaces. Unlike Backbone, which is a full MVC framework, React focuses solely on the view layer, allowing for more flexibility in choosing other libraries for state management and routing.
@types/angular provides TypeScript type definitions for AngularJS, a structural framework for dynamic web apps. AngularJS is more opinionated and provides a more comprehensive solution compared to Backbone, including dependency injection, two-way data binding, and a more complex templating system.
@types/vue provides TypeScript type definitions for Vue.js, a progressive JavaScript framework for building user interfaces. Vue.js is similar to Backbone in that it can be incrementally adopted, but it offers a more modern and reactive approach to building UIs.
npm install --save @types/backbone
This package contains type definitions for Backbone 1.0.0 (http://backbonejs.org/).
Files were exported from https://www.github.com/DefinitelyTyped/DefinitelyTyped/tree/types-2.0/backbone
Additional Details
These definitions were written by Boris Yankov https://github.com/borisyankov/, Natan Vivo https://github.com/nvivo/.
FAQs
TypeScript definitions for backbone
We found that @types/backbone demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.