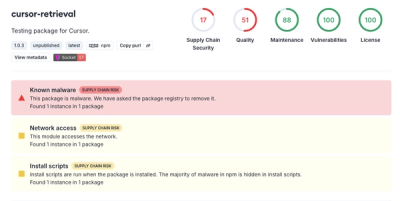
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@types/bonjour
Advanced tools
TypeScript definitions for bonjour
@types/bonjour provides TypeScript definitions for the bonjour package, which is used for zero-configuration networking using multicast DNS (mDNS) service discovery. It allows you to publish and discover services on a local network without any configuration.
Publishing a Service
This feature allows you to publish a service on the local network. In this example, a web server service is published on port 3000.
const bonjour = require('bonjour')();
const service = bonjour.publish({ name: 'My Web Server', type: 'http', port: 3000 });
service.start();
Browsing for Services
This feature allows you to browse for services of a specific type on the local network. In this example, it searches for HTTP servers and logs the details of any found services.
const bonjour = require('bonjour')();
bonjour.find({ type: 'http' }, function (service) {
console.log('Found an HTTP server:', service);
});
Stopping a Service
This feature allows you to stop a published service. In this example, the previously published web server service is stopped.
const bonjour = require('bonjour')();
const service = bonjour.publish({ name: 'My Web Server', type: 'http', port: 3000 });
service.start();
// Later, stop the service
service.stop();
Unpublishing All Services
This feature allows you to unpublish all services that have been published by the bonjour instance. In this example, two services are published and then all services are unpublished.
const bonjour = require('bonjour')();
// Publish some services
const service1 = bonjour.publish({ name: 'Service1', type: 'http', port: 3000 });
const service2 = bonjour.publish({ name: 'Service2', type: 'http', port: 3001 });
service1.start();
service2.start();
// Unpublish all services
bonjour.unpublishAll();
The mdns package provides multicast DNS service discovery, similar to bonjour. It allows you to advertise and browse services on the local network. Compared to bonjour, mdns offers more low-level control over the mDNS protocol but may require more configuration.
The multicast-dns package is a low-level implementation of multicast DNS in JavaScript. It provides the basic building blocks for mDNS service discovery but does not include the higher-level abstractions found in bonjour. It is suitable for developers who need fine-grained control over mDNS operations.
The dnssd package is another option for DNS-SD (DNS Service Discovery) and mDNS. It provides a high-level API similar to bonjour but with additional features and optimizations. It is a good alternative for developers looking for a more feature-rich solution.
npm install --save @types/bonjour
This package contains type definitions for bonjour (https://github.com/watson/bonjour).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/bonjour.
These definitions were written by Quentin Lampin.
FAQs
TypeScript definitions for bonjour
The npm package @types/bonjour receives a total of 7,470,407 weekly downloads. As such, @types/bonjour popularity was classified as popular.
We found that @types/bonjour demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.