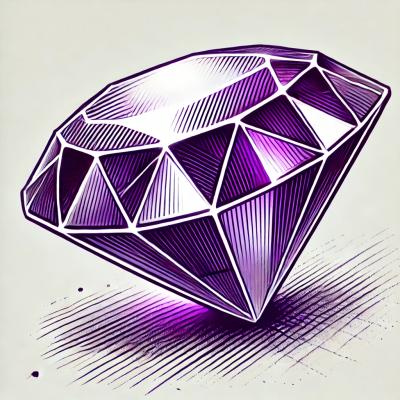
Security News
Highlights from the 2024 Rails Community Survey
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
@types/d3-hierarchy
Advanced tools
TypeScript definitions for d3-hierarchy
@types/d3-hierarchy provides TypeScript type definitions for the d3-hierarchy module, which is part of the D3.js library. This module is used for creating and manipulating hierarchical data structures such as trees, clusters, partitions, and treemaps.
Tree Layout
The tree layout organizes data into a tree structure. The `d3.hierarchy` function is used to create a root node from the data, and `d3.tree` generates the tree layout.
const root = d3.hierarchy(data);
const treeLayout = d3.tree();
treeLayout(root);
Cluster Layout
The cluster layout arranges nodes in a dendrogram. Similar to the tree layout, `d3.hierarchy` creates the root node, and `d3.cluster` generates the cluster layout.
const root = d3.hierarchy(data);
const clusterLayout = d3.cluster();
clusterLayout(root);
Treemap Layout
The treemap layout displays hierarchical data as a set of nested rectangles. The `d3.hierarchy` function creates the root node, and `d3.treemap` generates the treemap layout.
const root = d3.hierarchy(data).sum(d => d.value);
const treemapLayout = d3.treemap();
treemapLayout(root);
Partition Layout
The partition layout is used to create a space-filling visualization of hierarchical data. The `d3.hierarchy` function creates the root node, and `d3.partition` generates the partition layout.
const root = d3.hierarchy(data).sum(d => d.value);
const partitionLayout = d3.partition();
partitionLayout(root);
Pack Layout
The pack layout arranges nodes in a circle-packing layout. The `d3.hierarchy` function creates the root node, and `d3.pack` generates the pack layout.
const root = d3.hierarchy(data).sum(d => d.value);
const packLayout = d3.pack();
packLayout(root);
The d3 package is the core library of D3.js, which includes a wide range of data visualization tools. While @types/d3-hierarchy focuses on hierarchical layouts, the d3 package provides a comprehensive set of tools for creating various types of visualizations.
The d3-hierarchy package is the JavaScript implementation of the hierarchical layouts provided by D3.js. It is the core library that @types/d3-hierarchy provides type definitions for, and it includes the actual implementation of the hierarchical data structures and layouts.
The d3-tree package is a specialized library for creating tree visualizations using D3.js. It offers more focused functionality for tree layouts compared to the broader hierarchical layouts provided by @types/d3-hierarchy.
npm install --save @types/d3-hierarchy
This package contains type definitions for d3-hierarchy (https://github.com/d3/d3-hierarchy/).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/d3-hierarchy.
These definitions were written by Tom Wanzek, Alex Ford, Boris Yankov, denisname, Nathan Bierema, and Fil.
FAQs
TypeScript definitions for d3-hierarchy
The npm package @types/d3-hierarchy receives a total of 1,577,914 weekly downloads. As such, @types/d3-hierarchy popularity was classified as popular.
We found that @types/d3-hierarchy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.