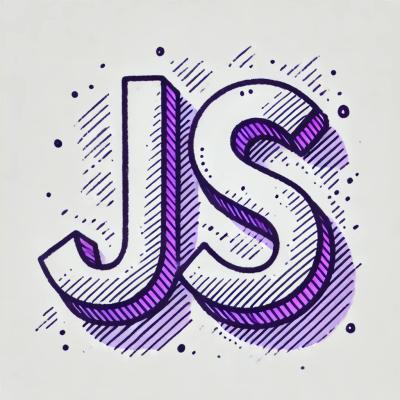
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
@types/googlemaps
Advanced tools
@types/googlemaps provides TypeScript type definitions for the Google Maps JavaScript API, enabling developers to use Google Maps functionalities with type safety and autocompletion in TypeScript projects.
Map Initialization
This feature allows you to initialize a Google Map with a specified center and zoom level.
const map = new google.maps.Map(document.getElementById('map'), { center: { lat: -34.397, lng: 150.644 }, zoom: 8 });
Adding Markers
This feature allows you to add markers to the map at specified locations.
const marker = new google.maps.Marker({ position: { lat: -34.397, lng: 150.644 }, map: map, title: 'Hello World!' });
Geocoding
This feature allows you to convert addresses into geographic coordinates and place markers on the map.
const geocoder = new google.maps.Geocoder(); geocoder.geocode({ 'address': 'Sydney, NSW' }, function(results, status) { if (status === 'OK') { map.setCenter(results[0].geometry.location); const marker = new google.maps.Marker({ map: map, position: results[0].geometry.location }); } else { alert('Geocode was not successful for the following reason: ' + status); } });
Drawing Shapes
This feature allows you to draw shapes like rectangles on the map, with options to make them editable and draggable.
const rectangle = new google.maps.Rectangle({ bounds: { north: 33.685, south: 33.671, east: -116.234, west: -116.251 }, editable: true, draggable: true }); rectangle.setMap(map);
@react-google-maps/api is a library for integrating Google Maps into React applications. It provides a set of React components and hooks for using Google Maps, making it easier to manage map state and lifecycle in React.
Leaflet is an open-source JavaScript library for mobile-friendly interactive maps. It is lightweight and easy to use, with a wide range of plugins available for additional functionalities. Unlike @types/googlemaps, Leaflet does not rely on Google Maps and can use various map tile providers.
Mapbox GL JS is a JavaScript library for interactive, customizable vector maps. It offers high performance and a wide range of features, including 3D terrain and vector tiles. Unlike @types/googlemaps, Mapbox GL JS uses Mapbox's own map data and services.
npm install --save @types/googlemaps
This package contains type definitions for Google Maps JavaScript API 3.20 (https://developers.google.com/maps/).
Files were exported from https://www.github.com/DefinitelyTyped/DefinitelyTyped/tree/types-2.0/googlemaps
Additional Details
These definitions were written by Folia A/S http://www.folia.dk, Chris Wrench https://github.com/cgwrench.
FAQs
TypeScript definitions for Google Maps JavaScript API
The npm package @types/googlemaps receives a total of 366,173 weekly downloads. As such, @types/googlemaps popularity was classified as popular.
We found that @types/googlemaps demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.