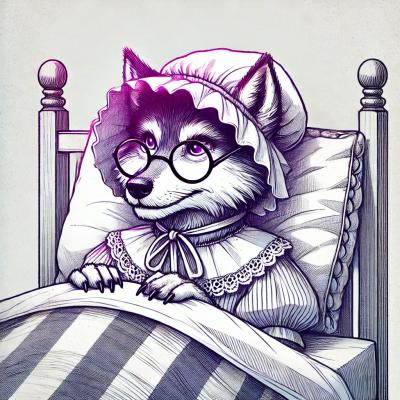
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@types/hast
Advanced tools
The @types/hast package provides TypeScript type definitions for HAST (Hypertext Abstract Syntax Tree) format, which is a virtual DOM format for HTML and XML. This allows developers to work with HAST trees in TypeScript projects with type safety, enabling better development experience, auto-completion, and error checking.
Creating a HAST node
This code sample demonstrates how to create a simple HAST node representing a paragraph element containing the text 'Hello, world!'. The node is typed, ensuring that only valid properties and child nodes are used.
{"type": "element", "tagName": "p", "properties": {}, "children": [{"type": "text", "value": "Hello, world!"}]}
Type-checking a HAST tree
This code sample shows how to type-check a HAST tree to safely access properties. It specifically checks if a node is an anchor ('a') element and logs its 'href' property if available.
import {Element} from 'hast';
function processNode(node: Element): void {
if (node.tagName === 'a' && node.properties) {
console.log(node.properties.href);
}
}
Rehype is a processor powered by plugins to work with HTML. It uses HAST under the hood, allowing manipulation of HTML documents. Compared to @types/hast, rehype offers higher-level operations like parsing, serializing, and transforming HTML content, whereas @types/hast focuses on providing type definitions.
Unified is an interface for processing text using syntax trees, including HAST for HTML. It's more general-purpose than @types/hast, offering a framework to parse, transform, and serialize text through a unified ecosystem of plugins, whereas @types/hast specifically provides TypeScript types for HAST nodes.
Remark is part of the unified ecosystem, focusing on Markdown processing using MDAST (Markdown Abstract Syntax Tree). While it serves a different syntax (Markdown vs. HTML/XML in HAST), it offers a similar concept of providing a structured tree format for content. Remark and @types/hast both enable developers to work with content trees, but they target different types of content.
npm install --save @types/hast
This package contains type definitions for Hast (https://github.com/syntax-tree/hast).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/hast.
// Type definitions for non-npm package Hast 2.3
// Project: https://github.com/syntax-tree/hast
// Definitions by: lukeggchapman <https://github.com/lukeggchapman>
// Junyoung Choi <https://github.com/rokt33r>
// Christian Murphy <https://github.com/ChristianMurphy>
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
// TypeScript Version: 3.0
import { Parent as UnistParent, Literal as UnistLiteral, Node as UnistNode } from 'unist';
export { UnistNode as Node };
/**
* Node in hast containing other nodes.
*/
export interface Parent extends UnistParent {
/**
* List representing the children of a node.
*/
children: Array<Element | DocType | Comment | Text>;
}
/**
* Nodes in hast containing a value.
*/
export interface Literal extends UnistLiteral {
value: string;
}
/**
* Root represents a document.
* Can be used as the rood of a tree, or as a value of the
* content field on a 'template' Element, never as a child.
*/
export interface Root extends Parent {
/**
* Represents this variant of a Node.
*/
type: 'root';
}
/**
* Element represents an HTML Element.
*/
export interface Element extends Parent {
/**
* Represents this variant of a Node.
*/
type: 'element';
/**
* Represents the element’s local name.
*/
tagName: string;
/**
* Represents information associated with the element.
*/
properties?: Properties | undefined;
/**
* If the tagName field is 'template', a content field can be present.
*/
content?: Root | undefined;
/**
* List representing the children of a node.
*/
children: Array<Element | Comment | Text>;
}
/**
* Represents information associated with an element.
*/
export interface Properties {
[PropertyName: string]: boolean | number | string | null | undefined | Array<string | number>;
}
/**
* Represents an HTML DocumentType.
*/
export interface DocType extends UnistNode {
/**
* Represents this variant of a Node.
*/
type: 'doctype';
name: string;
}
/**
* Represents an HTML Comment.
*/
export interface Comment extends Literal {
/**
* Represents this variant of a Literal.
*/
type: 'comment';
}
/**
* Represents an HTML Text.
*/
export interface Text extends Literal {
/**
* Represents this variant of a Literal.
*/
type: 'text';
}
These definitions were written by lukeggchapman, Junyoung Choi, and Christian Murphy.
FAQs
TypeScript definitions for hast
The npm package @types/hast receives a total of 7,054,476 weekly downloads. As such, @types/hast popularity was classified as popular.
We found that @types/hast demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.