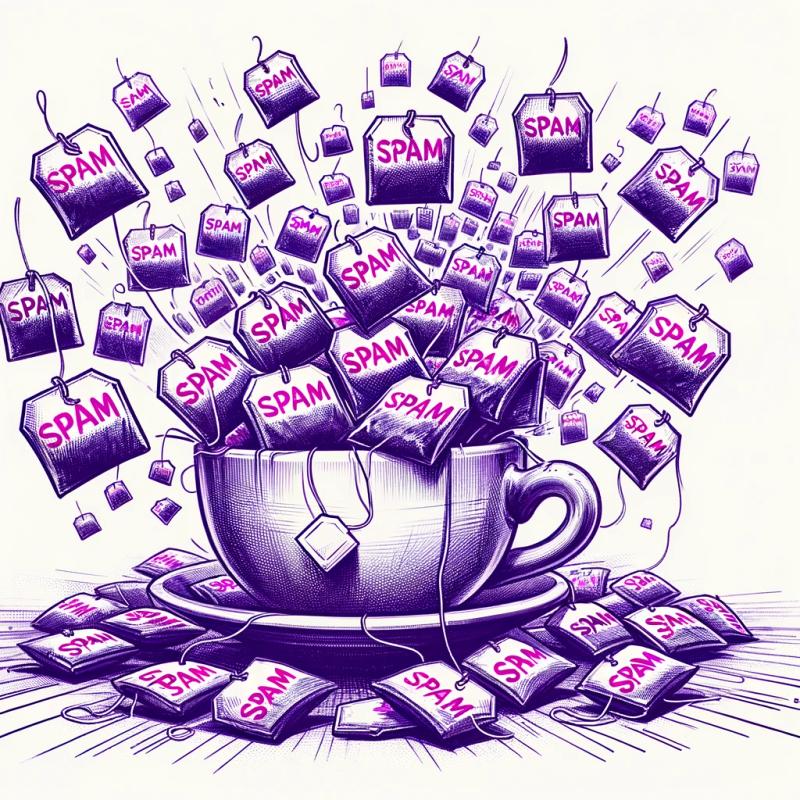
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@types/http-proxy
Advanced tools
Package description
The @types/http-proxy package provides TypeScript type definitions for the http-proxy library, which is a full-featured HTTP proxy for node.js. This package allows TypeScript developers to use http-proxy in their projects with the benefits of type checking and IntelliSense in their code editors. It does not provide proxy functionality by itself but adds type definitions for easier development with TypeScript.
Creating an HTTP Proxy Server
This code sample demonstrates how to create a basic HTTP proxy server that forwards incoming requests to 'http://example.com'. It showcases the use of the 'createProxyServer' method to create a proxy instance and the 'web' method to proxy HTTP requests.
import * as http from 'http';
import * as httpProxy from 'http-proxy';
const proxy = httpProxy.createProxyServer({});
const server = http.createServer(function(req, res) {
proxy.web(req, res, { target: 'http://example.com' });
});
server.listen(8000);
Listening for Proxy Events
This code sample shows how to listen for errors on the proxy server. When an error occurs, it sends a 500 response to the client with a message indicating that something went wrong. This is useful for handling and logging errors.
proxy.on('error', function (err, req, res) {
res.writeHead(500, {
'Content-Type': 'text/plain'
});
res.end('Something went wrong.');
});
http-proxy-middleware is a package that provides an express middleware to create a proxy server. It is built on top of http-proxy and offers a convenient way to integrate proxy functionality into Express applications. Compared to @types/http-proxy, http-proxy-middleware is more focused on integration with Express and other similar frameworks.
node-http-proxy is the underlying library that @types/http-proxy provides types for. It is a full-featured HTTP proxy library for Node.js, supporting websockets and other advanced features. While node-http-proxy provides the actual functionality, @types/http-proxy adds TypeScript support.
Readme
npm install --save @types/http-proxy
This package contains type definitions for http-proxy (https://github.com/nodejitsu/node-http-proxy).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/http-proxy.
These definitions were written by Maxime LUCE, Florian Oellerich, Daniel Schmidt, Jordan Abreu, and Samuel Bodin.
FAQs
TypeScript definitions for http-proxy
The npm package @types/http-proxy receives a total of 8,957,149 weekly downloads. As such, @types/http-proxy popularity was classified as popular.
We found that @types/http-proxy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.