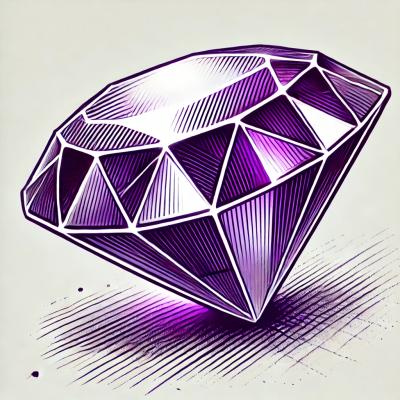
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@types/lodash.get
Advanced tools
TypeScript definitions for lodash.get
@types/lodash.get is a TypeScript type definition package for the lodash.get function, which is part of the Lodash library. Lodash.get is used to safely access deeply nested properties in objects without having to check if each level of the object exists.
Accessing Nested Properties
This feature allows you to access deeply nested properties in an object safely. If the property does not exist, it returns undefined.
const _ = require('lodash.get');
const object = { 'a': [{ 'b': { 'c': 3 } }] };
const value = _.get(object, 'a[0].b.c');
console.log(value); // Output: 3
Default Value
This feature allows you to specify a default value that will be returned if the property does not exist.
const _ = require('lodash.get');
const object = { 'a': [{ 'b': { 'c': 3 } }] };
const value = _.get(object, 'a[0].b.d', 'default');
console.log(value); // Output: 'default'
dot-prop is a package that provides similar functionality to lodash.get. It allows you to get, set, and delete nested properties in an object using a dot path. It is more lightweight compared to lodash.get.
object-path is another package that offers similar capabilities to lodash.get. It provides methods to get, set, and delete nested properties in an object. It also supports arrays and has additional utility methods.
npm install --save @types/lodash.get
This package contains type definitions for lodash.get (https://lodash.com).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/lodash.get.
// Generated from https://github.com/DefinitelyTyped/DefinitelyTyped/blob/master/types/lodash/scripts/generate-modules.ts
import { get } from "lodash";
export = get;
These definitions were written by Brian Zengel, and Ilya Mochalov.
FAQs
TypeScript definitions for lodash.get
The npm package @types/lodash.get receives a total of 130,026 weekly downloads. As such, @types/lodash.get popularity was classified as popular.
We found that @types/lodash.get demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.