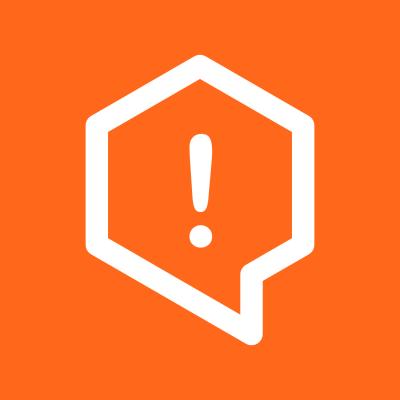
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@types/minimatch
Advanced tools
TypeScript definitions for minimatch
The @types/minimatch package provides TypeScript type definitions for the minimatch library, which is a utility for matching strings against Unix shell style patterns. It enables developers to use minimatch in TypeScript projects with type checking, enhancing development experience by providing autocompletion and error checking based on the expected types of minimatch's functions and options.
Pattern Matching
Match strings against a specified glob pattern. This is useful for filtering file names, paths, or other strings according to a pattern defined in a way similar to Unix shell commands.
import minimatch from 'minimatch';
const match = minimatch('path/to/file.js', '*.js');
console.log(match); // true or false
Using Options
Customize the matching behavior using options. For example, 'nocase' enables case-insensitive matching. This allows for more flexible and powerful string matching based on specific requirements.
import minimatch from 'minimatch';
const options = { nocase: true };
const match = minimatch('path/to/FILE.js', '*.js', options);
console.log(match); // true
Micromatch is a modern and faster alternative to minimatch with a similar feature set. It offers fine-grained control over the matching and globbing behavior, supports multiple patterns, and has a more extensive list of options for customization. Compared to @types/minimatch, micromatch provides its own TypeScript types, eliminating the need for separate type definitions.
Glob is another popular package for matching files using the Unix shell pattern syntax. It not only matches patterns against strings but also implements filesystem operations to find files matching the patterns. While @types/minimatch focuses on providing type definitions for string pattern matching, glob extends the functionality to actual file system querying, which can be more useful in scenarios involving file manipulation and searching.
npm install --save @types/minimatch
This package contains type definitions for minimatch (https://github.com/isaacs/minimatch).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/minimatch.
These definitions were written by vvakame, Shant Marouti, and BendingBender.
FAQs
TypeScript definitions for minimatch
The npm package @types/minimatch receives a total of 15,450,945 weekly downloads. As such, @types/minimatch popularity was classified as popular.
We found that @types/minimatch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.