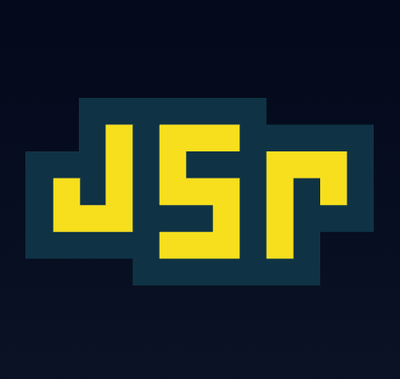
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@types/multer
Advanced tools
The @types/multer package provides TypeScript type definitions for Multer, a node.js middleware for handling multipart/form-data, primarily used for uploading files. It allows developers to use Multer in TypeScript projects with type checking, enabling better development experience and error handling.
File Upload
This feature allows for the uploading of files. The code sample demonstrates how to set up a simple file upload endpoint using Express and Multer, where files are saved to the 'uploads/' directory.
import express from 'express';
import multer from 'multer';
const upload = multer({ dest: 'uploads/' });
const app = express();
app.post('/upload', upload.single('file'), function (req, res) {
console.log(req.file);
res.send('File uploaded successfully.');
});
Multiple Files Upload
This feature supports the uploading of multiple files in a single request. The code sample shows how to configure an endpoint to accept a maximum of 5 files using the `upload.array` method.
import express from 'express';
import multer from 'multer';
const upload = multer({ dest: 'uploads/' });
const app = express();
app.post('/upload', upload.array('files', 5), function (req, res) {
console.log(req.files);
res.send('Files uploaded successfully.');
});
File Filtering
This feature allows for filtering files based on specific criteria, such as file type. The code sample demonstrates how to accept only JPEG files using a custom file filter.
import express from 'express';
import multer from 'multer';
const fileFilter = (req, file, cb) => {
if (file.mimetype === 'image/jpeg') {
cb(null, true);
} else {
cb(new Error('Only JPEG files are allowed!'), false);
}
};
const upload = multer({ dest: 'uploads/', fileFilter });
const app = express();
app.post('/upload', upload.single('file'), function (req, res) {
res.send('File uploaded successfully.');
});
Formidable is a Node.js module for parsing form data, especially file uploads. It differs from Multer in that it's lower level, providing a more flexible API for parsing incoming form data, including file uploads, but requires more setup compared to Multer.
Busboy is a fast, streaming parser for HTML form data for Node.js, focusing on performance. It's similar to Multer in handling file uploads but is more stream-oriented, making it suitable for handling large files or high volumes of data efficiently.
npm install --save @types/multer
This package contains type definitions for multer (https://github.com/expressjs/multer).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/multer.
These definitions were written by jt000 (https://github.com/jt000), vilicvane (https://github.com/vilic), David Broder-Rodgers (https://github.com/DavidBR-SW), Michael Ledin (https://github.com/mxl), HyunSeob Lee (https://github.com/hyunseob), Pierre Tchuente (https://github.com/PierreTchuente), and Oliver Emery (https://github.com/thrymgjol).
FAQs
TypeScript definitions for multer
The npm package @types/multer receives a total of 1,572,624 weekly downloads. As such, @types/multer popularity was classified as popular.
We found that @types/multer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.