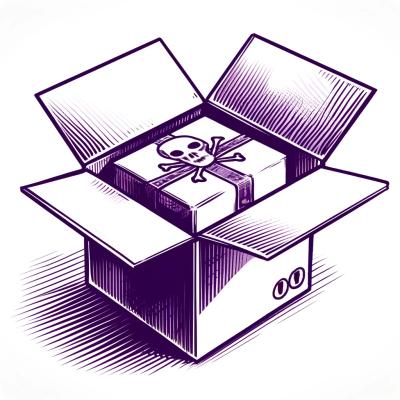
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@types/react-signature-canvas
Advanced tools
TypeScript definitions for react-signature-canvas
@types/react-signature-canvas provides TypeScript definitions for the react-signature-canvas package, which is a React wrapper for the signature_pad library. This package allows you to easily integrate a signature canvas into your React applications, enabling users to draw signatures or other freehand drawings.
Basic Signature Canvas
This code demonstrates how to set up a basic signature canvas using the react-signature-canvas package. The canvas allows users to draw signatures with a black pen.
import React from 'react';
import SignatureCanvas from 'react-signature-canvas';
const SignaturePad = () => {
let sigPad = React.useRef(null);
return (
<div>
<SignatureCanvas ref={(ref) => { sigPad = ref; }} penColor='black' canvasProps={{width: 500, height: 200, className: 'sigCanvas'}} />
</div>
);
};
export default SignaturePad;
Clear Signature Canvas
This code adds a button to clear the signature canvas. When the button is clicked, the clear function is called, which clears the canvas.
import React from 'react';
import SignatureCanvas from 'react-signature-canvas';
const SignaturePad = () => {
let sigPad = React.useRef(null);
const clear = () => {
sigPad.clear();
};
return (
<div>
<SignatureCanvas ref={(ref) => { sigPad = ref; }} penColor='black' canvasProps={{width: 500, height: 200, className: 'sigCanvas'}} />
<button onClick={clear}>Clear</button>
</div>
);
};
export default SignaturePad;
Save Signature as Image
This code adds a button to save the signature as an image. When the button is clicked, the save function is called, which logs the data URL of the signature image to the console.
import React from 'react';
import SignatureCanvas from 'react-signature-canvas';
const SignaturePad = () => {
let sigPad = React.useRef(null);
const save = () => {
const dataURL = sigPad.toDataURL();
console.log(dataURL);
};
return (
<div>
<SignatureCanvas ref={(ref) => { sigPad = ref; }} penColor='black' canvasProps={{width: 500, height: 200, className: 'sigCanvas'}} />
<button onClick={save}>Save</button>
</div>
);
};
export default SignaturePad;
react-signature-pad-wrapper is another React wrapper for the signature_pad library. It provides similar functionality to react-signature-canvas, allowing users to draw signatures on a canvas. However, it may have different APIs and additional features.
react-signature-pad is a React component for signature_pad. It offers similar capabilities for capturing signatures in a React application. The main difference is in the implementation and API, which might be more suitable for certain use cases.
npm install --save @types/react-signature-canvas
This package contains type definitions for react-signature-canvas (https://github.com/agilgur5/react-signature-canvas).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/react-signature-canvas.
import * as React from "react";
import SignaturePad = require("signature_pad");
declare namespace ReactSignatureCanvas {
interface ReactSignatureCanvasProps extends SignaturePad.SignaturePadOptions {
/** directly passed to the underlying <canvas /> element */
canvasProps?: React.CanvasHTMLAttributes<HTMLCanvasElement> | undefined;
/**
* whether or not the canvas should be cleared when the window resizes
* @default true
*/
clearOnResize?: boolean | undefined;
}
}
declare class ReactSignatureCanvas extends React.Component<ReactSignatureCanvas.ReactSignatureCanvasProps> {
/** rebinds all event handlers */
on: SignaturePad["on"];
/** unbinds all event handlers */
off: SignaturePad["off"];
/** clears the canvas using the {@link ReactSignatureCanvas.ReactSignatureCanvasProps.backgroundColor|backgroundColor} prop */
clear: SignaturePad["clear"];
isEmpty: SignaturePad["isEmpty"];
/** writes a base64 image to canvas */
fromDataURL: SignaturePad["fromDataURL"];
/** returns the signature image as a data URL */
toDataURL: SignaturePad["toDataURL"];
/** draws signature image from an array of point groups */
fromData: SignaturePad["fromData"];
/** returns signature image as an array of point groups */
toData: SignaturePad["toData"];
/** returns the underlying canvas ref. Allows you to modify the canvas however you want or call methods such as {@link ReactSignatureCanvas.toDataURL|toDataURL()} */
getCanvas(): HTMLCanvasElement;
/** creates a copy of the canvas and returns a {@link https://github.com/agilgur5/trim-canvas|trimmed version} of it, with all whitespace removed. */
getTrimmedCanvas(): HTMLCanvasElement;
/** returns the underlying SignaturePad reference */
getSignaturePad(): SignaturePad;
}
export = ReactSignatureCanvas;
These definitions were written by Kamil Socha.
FAQs
TypeScript definitions for react-signature-canvas
The npm package @types/react-signature-canvas receives a total of 87,820 weekly downloads. As such, @types/react-signature-canvas popularity was classified as popular.
We found that @types/react-signature-canvas demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.