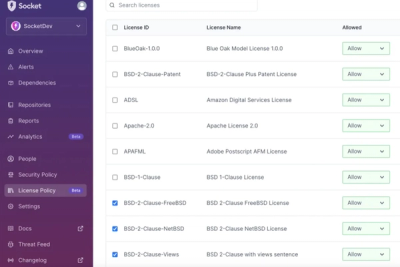
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
@types/recharts
Advanced tools
TypeScript definitions for recharts
@types/recharts provides TypeScript definitions for the Recharts library, which is a composable charting library built on React components. It allows developers to create a variety of charts and visualizations with ease, ensuring type safety and better development experience in TypeScript projects.
Line Chart
This code demonstrates how to create a simple Line Chart using Recharts. It includes axes, grid, tooltips, and legends.
import { LineChart, Line, XAxis, YAxis, CartesianGrid, Tooltip, Legend } from 'recharts';
const data = [
{ name: 'Page A', uv: 4000, pv: 2400, amt: 2400 },
{ name: 'Page B', uv: 3000, pv: 1398, amt: 2210 },
{ name: 'Page C', uv: 2000, pv: 9800, amt: 2290 },
{ name: 'Page D', uv: 2780, pv: 3908, amt: 2000 },
{ name: 'Page E', uv: 1890, pv: 4800, amt: 2181 },
{ name: 'Page F', uv: 2390, pv: 3800, amt: 2500 },
{ name: 'Page G', uv: 3490, pv: 4300, amt: 2100 },
];
const MyLineChart = () => (
<LineChart width={600} height={300} data={data}>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="name" />
<YAxis />
<Tooltip />
<Legend />
<Line type="monotone" dataKey="pv" stroke="#8884d8" />
<Line type="monotone" dataKey="uv" stroke="#82ca9d" />
</LineChart>
);
Bar Chart
This code demonstrates how to create a Bar Chart using Recharts. It includes axes, grid, tooltips, and legends.
import { BarChart, Bar, XAxis, YAxis, CartesianGrid, Tooltip, Legend } from 'recharts';
const data = [
{ name: 'Page A', uv: 4000, pv: 2400, amt: 2400 },
{ name: 'Page B', uv: 3000, pv: 1398, amt: 2210 },
{ name: 'Page C', uv: 2000, pv: 9800, amt: 2290 },
{ name: 'Page D', uv: 2780, pv: 3908, amt: 2000 },
{ name: 'Page E', uv: 1890, pv: 4800, amt: 2181 },
{ name: 'Page F', uv: 2390, pv: 3800, amt: 2500 },
{ name: 'Page G', uv: 3490, pv: 4300, amt: 2100 },
];
const MyBarChart = () => (
<BarChart width={600} height={300} data={data}>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="name" />
<YAxis />
<Tooltip />
<Legend />
<Bar dataKey="pv" fill="#8884d8" />
<Bar dataKey="uv" fill="#82ca9d" />
</BarChart>
);
Pie Chart
This code demonstrates how to create a Pie Chart using Recharts. It includes tooltips and legends.
import { PieChart, Pie, Tooltip, Legend } from 'recharts';
const data = [
{ name: 'Group A', value: 400 },
{ name: 'Group B', value: 300 },
{ name: 'Group C', value: 300 },
{ name: 'Group D', value: 200 },
];
const MyPieChart = () => (
<PieChart width={400} height={400}>
<Pie data={data} dataKey="value" nameKey="name" cx="50%" cy="50%" outerRadius={60} fill="#8884d8" />
<Pie data={data} dataKey="value" nameKey="name" cx="50%" cy="50%" innerRadius={70} outerRadius={90} fill="#82ca9d" label />
<Tooltip />
<Legend />
</PieChart>
);
Victory is another React-based charting library that provides a wide range of chart types and is highly customizable. It offers a similar level of functionality to Recharts but with a different API and styling approach.
Nivo provides a rich set of dataviz components, built on top of D3 and React. It offers a variety of chart types and is known for its beautiful and highly customizable charts. Nivo also supports server-side rendering.
React wrapper for Chart.js, a popular JavaScript charting library. It provides a simple and flexible way to create charts in React applications. While it may not be as composable as Recharts, it is widely used and has a large community.
npm install --save @types/recharts
This package contains type definitions for recharts (http://recharts.org/).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/recharts.
These definitions were written by Dmitriy Serdtsev.
FAQs
TypeScript definitions for recharts
The npm package @types/recharts receives a total of 195,083 weekly downloads. As such, @types/recharts popularity was classified as popular.
We found that @types/recharts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.