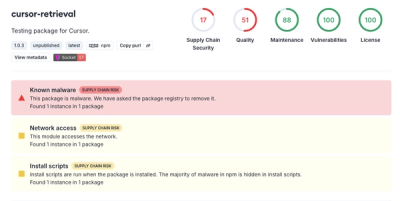
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@types/superagent
Advanced tools
TypeScript definitions for superagent
The @types/superagent package provides TypeScript type definitions for the superagent library, which is a lightweight, progressive AJAX API crafted for flexibility, readability, and a low learning curve. This package doesn't add any new functionality to superagent itself but allows TypeScript developers to use superagent in their projects with type checking, autocompletion, and other TypeScript benefits.
Making GET requests
This feature allows you to make GET requests to retrieve data from a specified resource. The code sample demonstrates how to make a simple GET request to '/api/pets' and log the response body.
import request from 'superagent';
request.get('/api/pets').then(res => {
console.log(res.body);
});
Posting data
This feature enables you to send data to a server using POST requests. The code sample shows how to post data to '/api/pets', sending an object with the name and species of a pet, and then logging the response body.
import request from 'superagent';
request.post('/api/pets')
.send({ name: 'Milo', species: 'cat' })
.then(res => {
console.log(res.body);
});
Setting headers
This feature allows you to set custom headers for your requests. In the code sample, a GET request is made to '/api/pets' with a custom 'API-Key' header.
import request from 'superagent';
request.get('/api/pets')
.set('API-Key', 'foobar')
.then(res => {
console.log(res.body);
});
Axios is a promise-based HTTP client for the browser and Node.js. It provides a simple API for making XMLHttpRequests and http requests. Compared to @types/superagent, Axios supports request and response interceptors, automatic transforms for JSON data, and client-side protection against XSRF.
node-fetch is a lightweight module that brings the Fetch API to Node.js. It aims to provide a consistent API with the browser's fetch API. Unlike @types/superagent, which is designed for use with superagent, node-fetch is more focused on mimicking the fetch API in Node.js environments.
Got is a human-friendly and powerful HTTP request library for Node.js. It supports retries, streams, and pagination out-of-the-box. Compared to @types/superagent, Got offers more advanced features like request retries, more comprehensive stream support, and built-in caching mechanisms.
npm install --save @types/superagent
This package contains type definitions for superagent (https://github.com/visionmedia/superagent).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/superagent.
These definitions were written by Nico Zelaya, Michael Ledin, Shrey Jain, Alec Zopf, Adam Haglund, Lukas Elmer, Jesse Rogers, Chris Arnesen, Anders Kindberg, LuckyWind_sck, and David Tanner.
FAQs
TypeScript definitions for superagent
We found that @types/superagent demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.