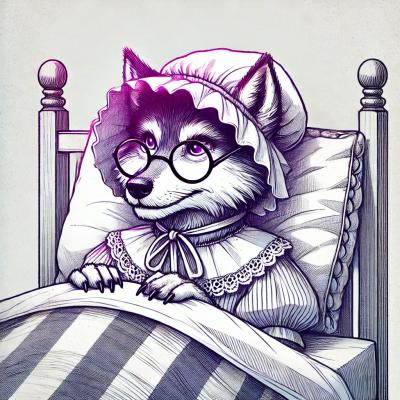
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@types/tedious
Advanced tools
@types/tedious provides TypeScript type definitions for the 'tedious' package, which is a TDS (Tabular Data Stream) protocol implementation for Node.js. It allows you to connect to and interact with Microsoft SQL Server databases.
Connecting to a SQL Server
This code demonstrates how to establish a connection to a SQL Server using the 'tedious' package. The configuration object includes server details, authentication options, and database name.
const { Connection } = require('tedious');
const config = {
server: 'your_server_name',
authentication: {
type: 'default',
options: {
userName: 'your_username',
password: 'your_password'
}
},
options: {
database: 'your_database_name'
}
};
const connection = new Connection(config);
connection.on('connect', err => {
if (err) {
console.error('Connection failed', err);
} else {
console.log('Connected');
}
});
Executing a SQL Query
This code demonstrates how to execute a SQL query using the 'tedious' package. It creates a new Request object with the SQL query and a callback function to handle the results.
const { Request } = require('tedious');
const request = new Request('SELECT * FROM your_table_name', (err, rowCount, rows) => {
if (err) {
console.error('Query failed', err);
} else {
console.log(`${rowCount} rows returned`);
rows.forEach(row => {
console.log(row);
});
}
});
connection.execSql(request);
Handling SQL Server Events
This code demonstrates how to handle various events emitted by the SQL Server connection, such as errors and connection closure.
connection.on('error', err => {
console.error('Connection error', err);
});
connection.on('end', () => {
console.log('Connection closed');
});
The 'mssql' package is a Microsoft SQL Server client for Node.js. It supports both Promises and async/await syntax, making it easier to work with modern JavaScript. Compared to 'tedious', 'mssql' provides a higher-level API and additional features like connection pooling.
The 'node-mssql' package is another SQL Server client for Node.js, built on top of 'tedious'. It offers a more user-friendly API and additional functionalities such as connection pooling and simplified query execution. It is often preferred for its ease of use and better integration with modern JavaScript features.
npm install --save @types/tedious
This package contains type definitions for tedious 1.8.0 (https://pekim.github.io/tedious).
Files were exported from https://www.github.com/DefinitelyTyped/DefinitelyTyped/tree/types-2.0/tedious
Additional Details
These definitions were written by Rogier Schouten https://github.com/rogierschouten.
FAQs
TypeScript definitions for tedious
The npm package @types/tedious receives a total of 696,444 weekly downloads. As such, @types/tedious popularity was classified as popular.
We found that @types/tedious demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.