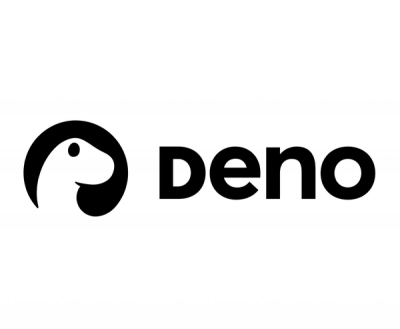
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@types/webpack-dev-server
Advanced tools
Stub TypeScript definitions entry for webpack-dev-server, which provides its own types definitions
@types/webpack-dev-server provides TypeScript type definitions for the webpack-dev-server package, which is a development server that provides live reloading and other features for a better development experience with webpack.
Live Reloading
Enables hot module replacement and live reloading, allowing changes to be applied without a full page reload.
const config: WebpackDevServer.Configuration = {
hot: true,
liveReload: true
};
Proxying API Requests
Proxies API requests to another server, useful for separating frontend and backend development.
const config: WebpackDevServer.Configuration = {
proxy: {
'/api': 'http://localhost:3000'
}
};
Custom Middleware
Allows the addition of custom middleware to the development server, enabling custom request handling.
const config: WebpackDevServer.Configuration = {
before(app) {
app.use((req, res, next) => {
console.log('Request URL:', req.url);
next();
});
}
};
Static File Serving
Serves static files from a specified directory, useful for serving assets like images and fonts.
const config: WebpackDevServer.Configuration = {
static: {
directory: path.join(__dirname, 'public'),
}
};
webpack-serve is another development server for webpack, offering similar features like live reloading and middleware support. However, it is less commonly used and not as actively maintained as webpack-dev-server.
browser-sync is a tool for synchronizing browser testing, offering live reloading, CSS injection, and more. It can be used with webpack but is not specifically designed for it, making it more versatile but potentially more complex to set up.
Parcel is a web application bundler that includes a built-in development server with live reloading and hot module replacement. It is simpler to set up than webpack but may not offer the same level of customization and control.
This is a stub types definition for @types/webpack-dev-server (https://github.com/webpack/webpack-dev-server#readme).
webpack-dev-server provides its own type definitions, so you don't need @types/webpack-dev-server installed!
FAQs
Stub TypeScript definitions entry for webpack-dev-server, which provides its own types definitions
We found that @types/webpack-dev-server demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.