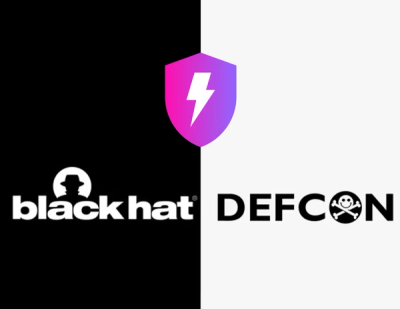
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
@typescript-eslint/typescript-estree
Advanced tools
A parser that converts TypeScript source code into an ESTree compatible form
The @typescript-eslint/typescript-estree package is a parser that converts TypeScript source code into an ESTree-compatible form. It is primarily used in the context of ESLint to enable linting of TypeScript code by converting TypeScript syntax into a format that ESLint can understand and work with.
Parsing TypeScript code to ESTree
This feature allows you to parse TypeScript code and get an Abstract Syntax Tree (AST) that is compatible with ESTree. This is useful for tools that need to analyze or manipulate the syntax of TypeScript code.
const tsEStree = require('@typescript-eslint/typescript-estree');
const code = 'let x: number = 1;';
const ast = tsEStree.parse(code, { jsx: false });
console.log(ast);
Parsing TypeScript code with JSX
This feature is similar to the previous one but includes support for JSX syntax, which is commonly used in React applications. It allows the parser to correctly interpret JSX elements within TypeScript code.
const tsEStree = require('@typescript-eslint/typescript-estree');
const code = '<div>Hello, TypeScript!</div>';
const ast = tsEStree.parse(code, { jsx: true });
console.log(ast);
Parsing a file
This feature allows you to parse the contents of a TypeScript file by reading the file and then parsing the code. It is useful when you want to analyze or lint a file directly.
const tsEStree = require('@typescript-eslint/typescript-estree');
const fs = require('fs');
const filePath = './example.ts';
const code = fs.readFileSync(filePath, 'utf8');
const ast = tsEStree.parse(code, { filePath });
console.log(ast);
This package was the predecessor to @typescript-eslint/typescript-estree and has been deprecated in favor of the newer package. It provided similar functionality in terms of parsing TypeScript code for ESLint.
babel-eslint is a parser that allows ESLint to run on source code that is transpiled with Babel. While it is not TypeScript-specific, it can be used with Babel's TypeScript preset to parse TypeScript code.
Espree is the default parser for ESLint and is built on top of Acorn. It is designed to parse ECMAScript (JavaScript) code. While it does not natively support TypeScript, it serves a similar purpose for JavaScript code as @typescript-eslint/typescript-estree does for TypeScript.
@typescript-eslint/typescript-estree
A parser that produces an ESTree-compatible AST for TypeScript code.
š See https://typescript-eslint.io/packages/typescript-estree for documentation on this package.
See https://typescript-eslint.io for general documentation on typescript-eslint, the tooling that allows you to run ESLint and Prettier on TypeScript code.
8.0.1 (2024-08-05)
You can read about our versioning strategy and releases on our website.
š See Announcing typescript-eslint v8 for an upgrade guide and the full list of changes.
reportUnusedIgnorePattern
option (#9324)ignoreClassWithStaticInitBlock
(#9325)projectService
in disabled-type-checked
shared config (#9460)RuleModuleWithMetaDocs
(#9465)You can read about our versioning strategy and releases on our website.
FAQs
A parser that converts TypeScript source code into an ESTree compatible form
The npm package @typescript-eslint/typescript-estree receives a total of 71,648,265 weekly downloads. As such, @typescript-eslint/typescript-estree popularity was classified as popular.
We found that @typescript-eslint/typescript-estree demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Ā It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600Ć faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.