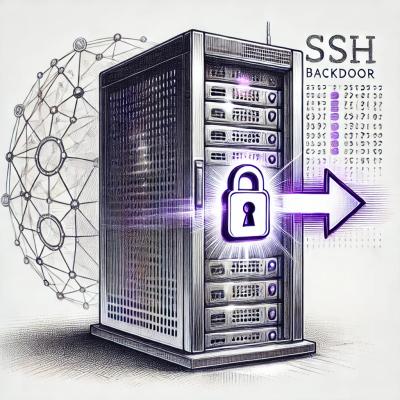
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@ueberfuhr/ngx-gitlab-client
Advanced tools
This Gitlab Client provides Angular-based services to call the [Gitlab REST API](https://docs.gitlab.com/ee/api/index.html).
This Gitlab Client provides Angular-based services to call the Gitlab REST API.
We have to import the GitlabClientModule
to make the services injectable.
For that, we need to define how to access Gitlab, i.e. which
host and security token to use.
We can easily configure the Gitlab connection with the following import:
@NgModule({
imports: [
GitlabClientModule.forRoot({
host: 'https://mygitlabhost/',
token: 'mygitlabtoken'
})
]
})
export class MyModule {
}
If the Gitlab connection is calculated during runtime and provided by a custom service,
we can import the GitlabClientModule
directly and then have to provide a GITLAB_CONNECTION_PROVIDER
:
@Injectable({providedIn: 'root'})
export class MyGitlabConfigService {
readConfiguration(): GitlabConfig {
// ...
}
}
@NgModule({
imports: [
GitlabClientModule
],
providers: [
{
provide: GITLAB_CONFIG_PROVIDER,
useFactory: (service: MyGitlabConfigService) => service.readConfiguration,
deps: [MyGitlabConfigService],
}
]
})
export class MyModule {
}
GitlabService
is a common service without any relation to a special REST resource.
We can use it, when we
Calls to Gitlab are made using the Angular HttpClient
. To invoke a simple get request
(using the configured Gitlab connection configuration), we can use
gitlab
.call<MyCommitType>('projects/5/repository/commits')
.subscribe(commit => {
// ...
})
We can also provide the HTTP method and some other options like additional headers:
gitlab
.call<MyCommitResult>('projects/5/repository/commits', 'post', {
body: myNewCommit,
headers: {
myHeaderName: myHeaderValue
}
})
.subscribe(result => {
// ...
})
For big data, Gitlab uses pagination. GitlabService
is able to handle it
and provides lazy loading, i.e. it only calls the API when we need to read the data.
We can simply use
gitlab
.callPaginated<MyType>('projects/5/repository/commits')
.pipe(take(10)) // only read the first 10 entries, then skip
.subscribe(dataset => {
let myObj = dataset.payload;
let index = dataset.index;
let total = dataset.total;
// ...
})
We have to be aware that the default page size is 20, so those 20 entries are read out with a single request. If we already know the count of entries we want to read, we could also specify a different page size:
gitlab
.callPaginated<MyType>('projects/5/repository/commits', null, 10)
We can get notified when a call to Gitlab is made or when an error occurred. E.g., this allows to provide a kind of component to display the current connection status:
export class GitlabConnectionStatusComponent implements OnInit, OnDestroy {
private accesses?: Subscription;
private errors?: Subscription;
constructor(private readonly gitlab: GitlabService) {
}
ngOnInit(): void {
this.accesses = this.gitlab.accesses.subscribe(access => {
// ... (access is type of GitlabAccess)
});
this.errors = this.gitlab.errors.subscribe(err => {
// ... (err is type of GitlabAccessError)
});
}
ngOnDestroy(): void {
this.accesses?.unsubscribe();
this.errors?.unsubscribe()
}
}
FAQs
This Gitlab Client provides Angular-based services to call the [Gitlab REST API](https://docs.gitlab.com/ee/api/index.html).
The npm package @ueberfuhr/ngx-gitlab-client receives a total of 0 weekly downloads. As such, @ueberfuhr/ngx-gitlab-client popularity was classified as not popular.
We found that @ueberfuhr/ngx-gitlab-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.