What is @ui5/builder?
@ui5/builder is a Node.js-based tool for building UI5 projects. It provides a set of functionalities to build, optimize, and bundle UI5 applications and libraries. The tool is part of the UI5 Tooling ecosystem, which aims to provide a modular and extensible tooling infrastructure for UI5 development.
What are @ui5/builder's main functionalities?
Build UI5 Projects
This feature allows you to build UI5 projects by specifying the project configuration and destination path. The build process compiles and optimizes the project for deployment.
const { build } = require('@ui5/builder');
const project = { /* project configuration */ };
build({
project,
destPath: 'dist'
}).then(() => {
console.log('Build successful');
}).catch((err) => {
console.error('Build failed', err);
});
Custom Tasks and Extensions
You can define custom tasks and extensions to extend the build process. This feature allows you to add custom logic to the build pipeline.
const { taskRepository } = require('@ui5/builder');
const customTask = {
name: 'customTask',
task: async function({ workspace, dependencies, taskUtil }) {
// Custom task logic
}
};
taskRepository.addTask(customTask);
Middleware Integration
This feature allows you to integrate custom middleware into the build process. Middleware can be used to handle HTTP requests during the build process.
const { middlewareRepository } = require('@ui5/builder');
const customMiddleware = {
name: 'customMiddleware',
middleware: function({ resources, options }) {
return function(req, res, next) {
// Custom middleware logic
next();
};
}
};
middlewareRepository.addMiddleware(customMiddleware);
Other packages similar to @ui5/builder
webpack
Webpack is a popular module bundler for JavaScript applications. It offers a wide range of plugins and loaders to handle different types of assets and optimize the build process. Compared to @ui5/builder, Webpack is more general-purpose and can be used for various types of projects, not just UI5.
gulp
Gulp is a toolkit for automating tasks in the development workflow. It uses a code-over-configuration approach and allows you to define tasks using JavaScript. Gulp is highly extensible and can be used for a variety of build tasks, similar to @ui5/builder, but it is not specifically tailored for UI5 projects.
grunt
Grunt is a JavaScript task runner that automates repetitive tasks like minification, compilation, and unit testing. It uses a configuration-over-code approach and has a large ecosystem of plugins. While Grunt can be used for building UI5 projects, it is more general-purpose compared to @ui5/builder.
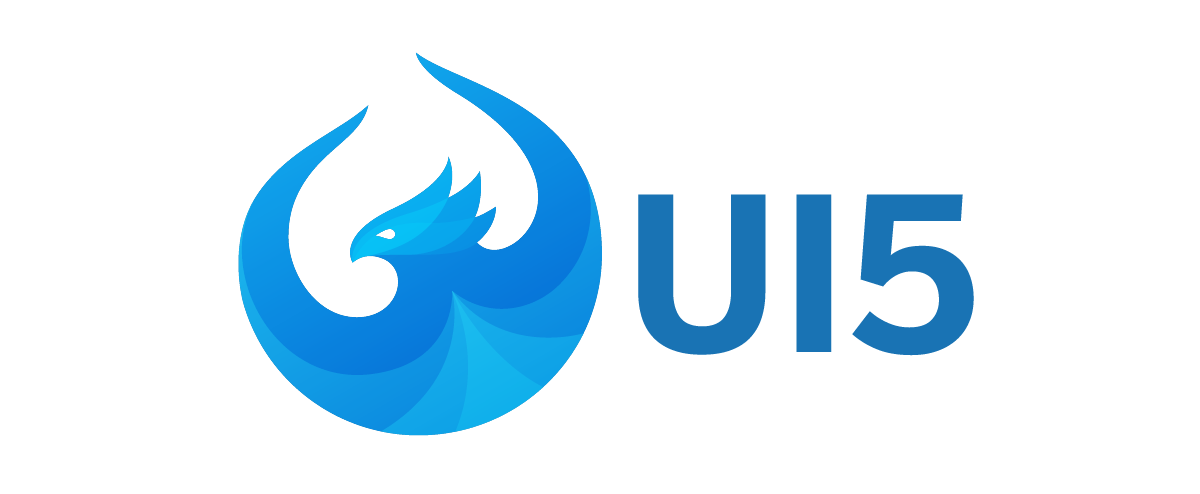
ui5-builder
Modules for building UI5 projects
Part of the UI5 Build and Development Tooling

This is an alpha release!
The UI5 Build and Development Tooling described here is not intended for productive use yet. Breaking changes are to be expected.
Builder
Types
Types define how a project can be configured and how it is being built. A type orchestrates a set of tasks and defines the order in which they get applied during build phase. Furthermore, it takes care of formatting and validating the project specific configuration.
Currently, the following types are defined:
The project type can be defined as part of a projects root folder.
Tasks
Tasks are specific build steps to be executed during build phase.
They are responsible for collecting resources which can be modified by a processor. A task configures one or more processors and supplies them with the collected resources. After the respective processor processed the resources, the task is able to continue with its workflow.
Available tasks are listed here.
Processors
Processors work with provided resources. They contain the actual build step logic to apply specific modifications to supplied resources or to make use of the resources' content to create new resources out of that.
Processors can be implemented generically. The string replacer is an example for that.
Since string replacement is a common build step, it can be useful in different contexts, e.g. code, version, date and copyright replacement. A concrete replacement operation could be achieved by passing a custom configuration to the processor. This way multiple tasks can make use of the same processor to achive their build step.
Available processors are listed here.
Legacy Bundle Tooling (lbt)
JavaScript port of the "legacy" Maven/Java based bundle tooling.
Contributing
Please check our Contribution Guidelines.
Support
Please follow our Contribution Guidelines on how to report an issue.
Release History
See CHANGELOG.md.
License
This project is licensed under the Apache Software License, Version 2.0 except as noted otherwise in the LICENSE file.