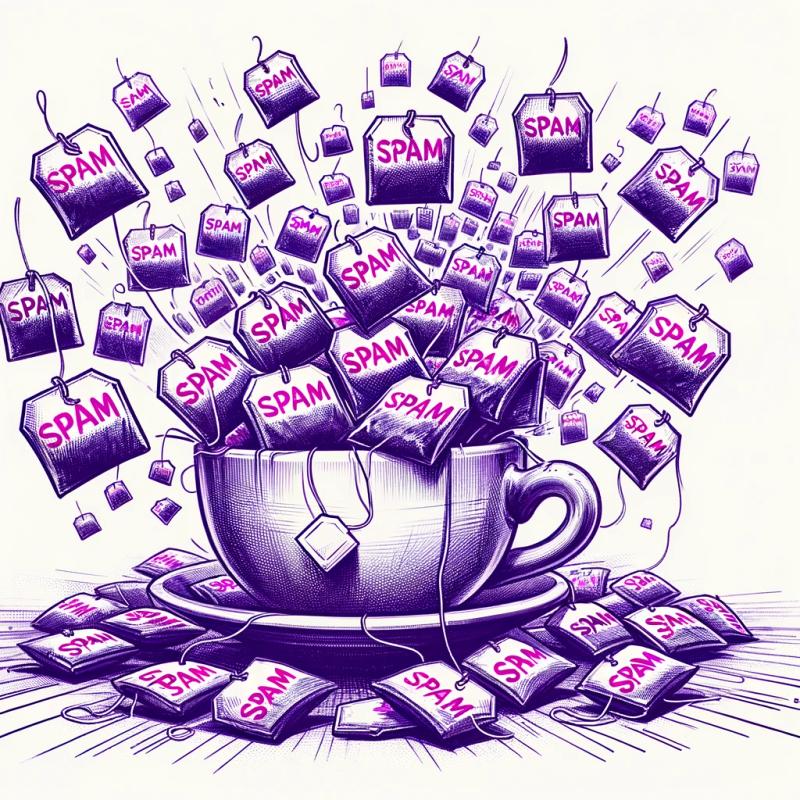
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@unique-nft/api
Advanced tools
Readme
Definitely typed JS API for the Unique Network
Install
yarn add @unique-nft/api
npm install @unique-nft/api
Since this project requires the BigInt support, there may be needed some additional bundler settings.
For example, you may need to add the following to your tsconfig.json
file:
"compilerOptions" {
...
"target": "es2020",
...
}
For Vite, you can do the same. Example of vite.config.ts
:
import { defineConfig } from 'vite'
// https://vitejs.dev/config/
export default defineConfig({
// ...
build: {
target: 'es2020'
}
})
One more thing before you start, please make sure that you delete the ^ symbol in the package.json
file. This is needed to avoid compatibility conflicts. The version of the library must be without this symbol:
"dependencies": {
"@unique-nft/api": "0.0.7",
Feel free to execute the code below to check some library features.
import {init, Substrate, utils} from '@unique-nft/api'
const run = async () => {
await init({})
console.log(utils.address.normalizeSubstrateAddress('yGHGXr2qCKygrxFw16XXEYRLmQwQt8RN8eMN5UuuJ17ZFPosP'))
const chain = new Substrate.Unique()
await chain.connect(`wss://quartz.unique.network`)
console.log(chain.ss58Prefix)
console.log(chain.coin.format(1_500_000_000_000_000_000n))
console.log(await chain.getChainProperties())
await chain.disconnect()
}
run().catch(err => console.error(err))
Initializing with Polkadot extension enabling (works only in browser)
import {init} from '@unique-nft/api'
await init({connectToPolkadotExtensionsAs: 'my app'})
or, another way to do the same:
import {init, Substrate} from '@unique-nft/api'
await init()
await Substrate.extension.connectAs('my app')
An extrinsic may be signed with keyring as well as with an account from the Polkadot extension.
With keyring (available in both browser and Node.js):
import {Substrate, init, WS_RPC} from '@unique-nft/api'
await init()
const chain = new Substrate.Unique()
await chain.connect(WS_RPC.quartz)
const keyring = Substrate.signer.keyringFromSeed('electric suit...')
const result = await chain.transferCoins({toAddress: "5C...", amountInWei: 1_500_000_000_000_000_000n}).signAndSend(keyring)
With the polkadot extension (available in browser only):
import {Substrate, WS_RPC} from '@unique-nft/api'
const quartz = new Substrate.Unique()
const kusama = new Substrate.Common()
await init({connectToPolkadotExtensionsAs: 'my app'})
// we can create instances before init
// but connect must be invoked only after init call
await quartz.connect(WS_RPC.quartz)
await kusama.connect(WS_RPC.kusama)
const accounts = await Substrate.extension.getAllAccounts()
const account = accounts[0]
// or, better option take some specific account
// accounts.find(account => account.address === '5...')
const KSMTransfer = await kusama.transferCoins({...}).signAndSend(account)
const QTZTransfer = await quartz.transferCoins({...}).signAndSend(account)
Note: in case of Substrate.Unique.transferCoins
(but not for Substrate.Common
),
we can pass both substrate address (starting from 5 in normal form)
and an Ethereum address (starting from 0x...).
Unique's transferCoins
(and other functions where it makes sense) can accept any address - substrate or ethereum.
Substrate
class provides methods which take extrinsic parameters and return an Extrinsic
instance.
Example:
const result = await quartz
.transferCoins({toAddress: '5...', amountInWei: 1n})
.signAndSend(keyringOrAccount)
More verbose example:
const quartz = new Substrate.Unique()
const tx = quartz.transferCoins({toAddress: '5...', amountInWei: 1n})
await tx.sign(keyringOrAccount)
console.log(tx.getRawTx().toJSON())
const result = await tx.send()
All params have typings which can be imported this way:
import {SubstrateMethodsParams} from '@unique-nft/api'
const params: SubstrateMethodsParams.TransferCoins = {
toAddress: '5...',
amountInWei: 1n
}
Extrinsic
instance fields and methods:
async sign(signer: KeyringPair | InjectedAccountWithMeta)
- returns it's instanceasync send()
- returns extrinsic resultasync signAndSend(signer: KeyringPair | InjectedAccountWithMeta)
- returns extrinsic resultisSigned
- booleangetRawTx()
- returns SubmittableExtrinsic
objectFAQs
Definitely typed JS API for the Unique Network blockchain
The npm package @unique-nft/api receives a total of 1 weekly downloads. As such, @unique-nft/api popularity was classified as not popular.
We found that @unique-nft/api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.