Vue File Upload Widget
(With Integrated Cloud Storage)
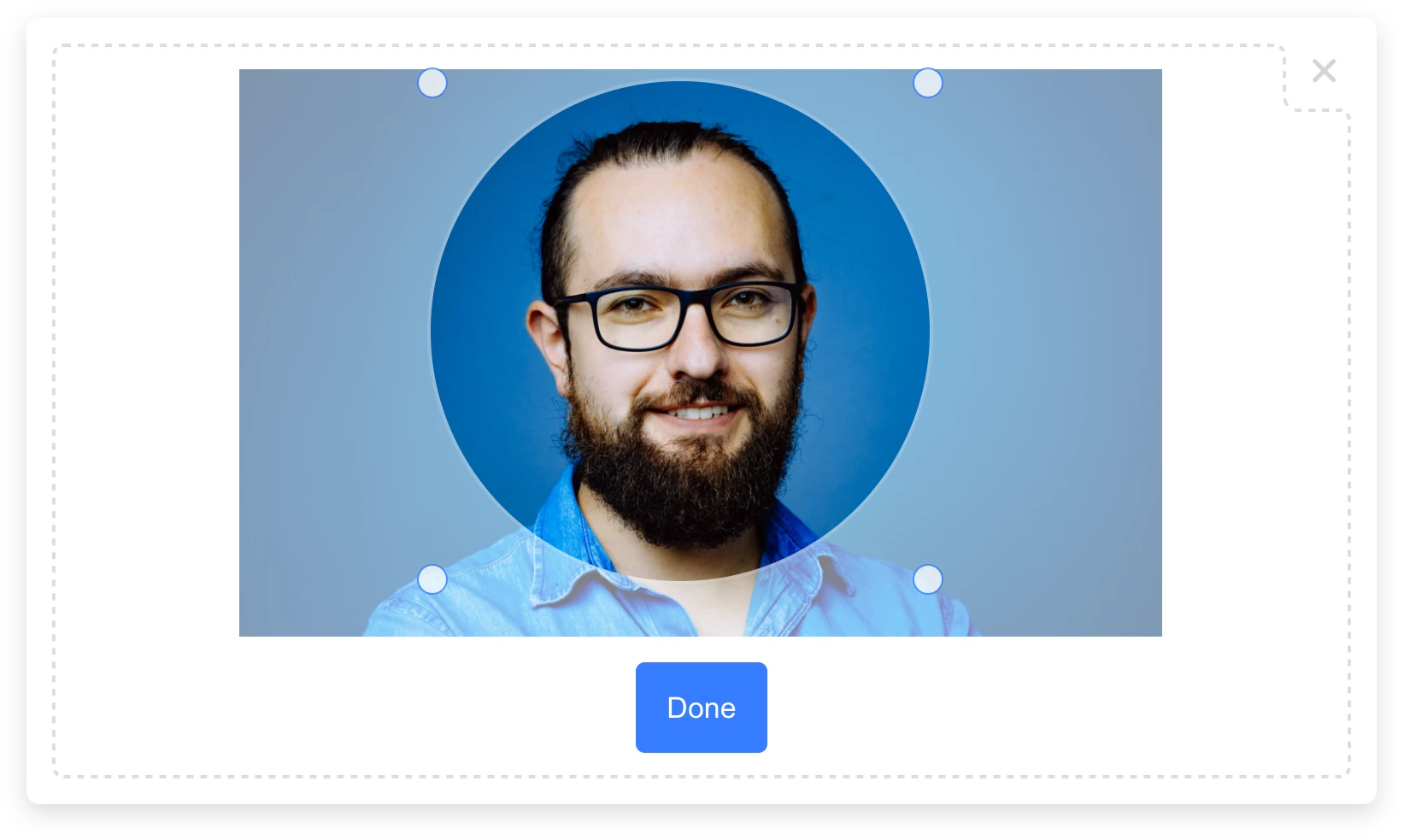
100% Serverless File Upload Widget
Powered by Bytescale
DMCA Compliant โข GDPR Compliant โข 99.9% Uptime SLA
Supports: Rate Limiting, Volume Limiting, File Size & Type Limiting, JWT Auth, and more...
Installation
Install via NPM:
npm install @upload-io/vue-uploader
Or via YARN:
yarn add @upload-io/vue-uploader
Or via a <script>
tag:
<script src="https://js.bytescale.com/vue-uploader/v3"></script>
Usage
Vue Uploader provides two options:
Option 1) File Upload Button โ Try on CodePen
Create a file upload button using the openUploadModal
helper:
<template>
<button @click="uploadFile">Upload a file...</button>
</template>
<script lang="ts">
import { Uploader } from "uploader";
import { openUploadModal } from "@upload-io/vue-uploader";
import type { UploadWidgetConfig, UploadWidgetResult } from "uploader";
import type { PreventableEvent } from "@upload-io/vue-uploader";
const uploader = Uploader({
apiKey: "free"
});
const options: UploadWidgetConfig = {
multi: true
};
export default {
name: "App",
methods: {
uploadFile(event: PreventableEvent) {
openUploadModal({
event,
uploader,
options,
onComplete: (files: UploadWidgetResult[]) => {
if (files.length === 0) {
alert("No files selected.");
} else {
alert(files.map(f => f.fileUrl).join("\n"));
}
}
})
}
}
};
</script>
Create a file upload dropzone using the UploadDropzone
component:
<template>
<UploadDropzone :uploader="uploader"
:options="options"
:on-update="onFileUploaded"
width="600px"
height="375px" />
</template>
<script lang="ts">
import { Uploader } from "uploader";
import { UploadDropzone } from "@upload-io/vue-uploader";
import type { UploadWidgetConfig, UploadWidgetResult } from "uploader";
const uploader = Uploader({ apiKey: "free" });
const options: UploadWidgetConfig = {
multi: true
};
export default {
name: "App",
components: {
UploadDropzone
},
data() {
return {
uploader,
options
};
},
methods: {
onFileUploaded(files: UploadWidgetResult[]) {
if (files.length === 0) {
alert("No files selected.");
} else {
alert(files.map(f => f.fileUrl).join("\n"));
}
}
}
};
</script>
The Result
The callbacks receive a Array<UploadWidgetResult>
:
{
fileUrl: "https://upcdn.io/FW25...",
filePath: "/uploads/example.jpg",
editedFile: undefined,
originalFile: {
fileUrl: "https://upcdn.io/FW25...",
filePath: "/uploads/example.jpg",
accountId: "FW251aX",
originalFileName: "example.jpg",
file: { ... },
size: 12345,
lastModified: 1663410542397,
mime: "image/jpeg",
metadata: {
...
},
tags: [
"tag1",
"tag2",
...
]
}
}
๐ API Support
๐ File Management API
Bytescale provides an Upload API, which supports the following:
- File uploading.
- File listing.
- File deleting.
- And more...
Uploading a "Hello World"
text file is as simple as:
curl --data "Hello World" \
-u apikey:free \
-X POST "https://api.bytescale.com/v1/files/basic"
Note: Remember to set -H "Content-Type: mime/type"
when uploading other file types!
Read the Upload API docs ยป
๐ Image Processing API (Resize, Crop, etc.)
Bytescale also provides an Image Processing API, which supports the following:
Read the Image Processing API docs ยป
Original Image
Here's an example using a photo of Chicago:
https://upcdn.io/W142hJk/raw/example/city-landscape.jpg
Processed Image
Using the Image Processing API, you can produce this image:
https://upcdn.io/W142hJk/image/example/city-landscape.jpg
?w=900
&h=600
&fit=crop
&f=webp
&q=80
&blur=4
&text=WATERMARK
&layer-opacity=80
&blend=overlay
&layer-rotate=315
&font-size=100
&padding=10
&font-weight=900
&color=ffffff
&repeat=true
&text=Chicago
&gravity=bottom
&padding-x=50
&padding-bottom=20
&font=/example/fonts/Lobster.ttf
&color=ffe400
Full Documentation
Uploader Documentation ยป
Need a Headless (no UI) File Upload Library?
Try Upload.js ยป
Can I use my own storage?
Yes: Bytescale supports AWS S3, Cloudflare R2, Google Storage, and DigitalOcean Spaces.
To configure a custom storage backend, please see:
https://www.bytescale.com/docs/storage/sources
๐ Create your Bytescale Account
Vue Uploader is the Vue Upload Widget for Bytescale: the best way to serve images, videos, and audio for web apps.
Create a Bytescale account ยป
Building From Source
BUILD.md
License
MIT