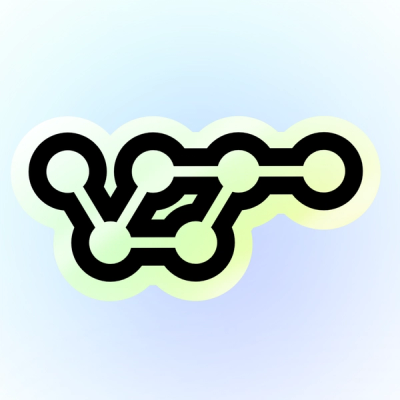
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
@upstash/ratelimit
Advanced tools
[](https://www.npmjs.com/package/@upstash/ratelimit) [](https://github.com/upstash/ratelimit/actions/workf
@upstash/ratelimit is a package designed to help developers implement rate limiting in their applications. It provides a simple and efficient way to control the rate of requests to a server, ensuring that resources are not overwhelmed by too many requests in a short period of time. This is particularly useful for APIs and web services that need to maintain performance and reliability.
Basic Rate Limiting
This code demonstrates how to set up basic rate limiting using @upstash/ratelimit. It limits requests to 10 per minute per IP address. If the limit is exceeded, it responds with a 429 status code.
const { Ratelimit } = require('@upstash/ratelimit');
const ratelimit = new Ratelimit({
redis: { url: 'your-redis-url', token: 'your-redis-token' },
limiter: { points: 10, duration: 60 },
});
async function handleRequest(req, res) {
const rateLimitResult = await ratelimit.limit(req.ip);
if (rateLimitResult.isRateLimited) {
res.status(429).send('Too Many Requests');
} else {
res.send('Request successful');
}
}
Custom Rate Limiting
This example shows how to customize the rate limiting settings. Here, the limit is set to 5 requests per 30 seconds per IP address.
const { Ratelimit } = require('@upstash/ratelimit');
const ratelimit = new Ratelimit({
redis: { url: 'your-redis-url', token: 'your-redis-token' },
limiter: { points: 5, duration: 30 },
});
async function handleRequest(req, res) {
const rateLimitResult = await ratelimit.limit(req.ip);
if (rateLimitResult.isRateLimited) {
res.status(429).send('Too Many Requests');
} else {
res.send('Request successful');
}
}
express-rate-limit is a popular middleware for Express.js applications that provides rate limiting capabilities. It is easy to integrate with Express and offers a variety of configuration options. Compared to @upstash/ratelimit, express-rate-limit is more tightly coupled with Express.js and may not be as flexible for use in non-Express environments.
rate-limiter-flexible is a highly flexible rate limiting library that supports various backends like Redis, MongoDB, and more. It offers advanced features such as cluster support and different rate limiting strategies. Compared to @upstash/ratelimit, rate-limiter-flexible provides more backend options and advanced features, but may require more setup and configuration.
[!NOTE] This project is in GA Stage. The Upstash Professional Support fully covers this project. It receives regular updates, and bug fixes. The Upstash team is committed to maintaining and improving its functionality.
It is the only connectionless (HTTP based) rate limiting library and designed for:
npm install @upstash/ratelimit
import { Ratelimit } from "https://cdn.skypack.dev/@upstash/ratelimit@latest";
Create a new redis database on upstash. See here for documentation on how to create a redis instance.
import { Ratelimit } from "@upstash/ratelimit"; // for deno: see above
import { Redis } from "@upstash/redis"; // see below for cloudflare and fastly adapters
// Create a new ratelimiter, that allows 10 requests per 10 seconds
const ratelimit = new Ratelimit({
redis: Redis.fromEnv(),
limiter: Ratelimit.slidingWindow(10, "10 s"),
analytics: true,
/**
* Optional prefix for the keys used in redis. This is useful if you want to share a redis
* instance with other applications and want to avoid key collisions. The default prefix is
* "@upstash/ratelimit"
*/
prefix: "@upstash/ratelimit",
});
// Use a constant string to limit all requests with a single ratelimit
// Or use a userID, apiKey or ip address for individual limits.
const identifier = "api";
const { success } = await ratelimit.limit(identifier);
if (!success) {
return "Unable to process at this time";
}
doExpensiveCalculation();
return "Here you go!";
For more information on getting started, you can refer to our documentation.
Here's a complete nextjs example
See the documentation for more information details about this package.
Create a new redis database on upstash and copy the url and token.
To run the tests, you will need to set some environment variables. Here is a list of variables to set:
UPSTASH_REDIS_REST_URL
UPSTASH_REDIS_REST_TOKEN
US1_UPSTASH_REDIS_REST_URL
US1_UPSTASH_REDIS_REST_TOKEN
APN_UPSTASH_REDIS_REST_URL
APN_UPSTASH_REDIS_REST_TOKEN
EU2_UPSTASH_REDIS_REST_URL
EU2_UPSTASH_REDIS_REST_TOKEN
You can create a single Upstash Redis and use its URL and token for all four above.
Once you set the environment variables, simply run:
pnpm test
FAQs
[](https://www.npmjs.com/package/@upstash/ratelimit) [](https://github.com/upstash/ratelimit/actions/workf
We found that @upstash/ratelimit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.