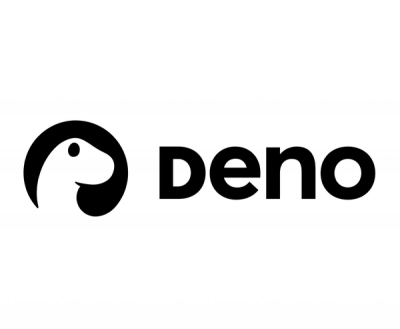
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@valu/lazy-script
Advanced tools
The smart way.
npm install @valu/lazy-script
Learn by example:
import { LazyScript } from "@valu/lazy-script";
const SCRIPT = new LazyScript({
// URL to the script to be loaded
src: "https://finmun.boost.ai/chatPanel/chatPanel.js",
// Initialization code to be executed only once when the script was loaded
initialize: () => {
if (typeof window.boostChatPanel !== "function") {
throw new Error("window.boostChatPanel not configured properly");
}
const chatPanel = window.boostChatPanel({
apiUrlBase: "https://finmun.boost.ai/api",
});
return chatPanel;
},
});
Lazily bind event handlers:
SCRIPT.lazy((chatPanel) => {
chatPanel.addEventListener("chatPanelClosed", () => {
alert("Chat closed");
});
});
Load the code immediately:
document.querySelector("button").addEventListener("click", () => {
SCRIPT.now((chatPanel) => {
chatPanel.show();
});
});
Things to note:
.now()
triggers all previous and future .lazy()
bindings.lazy()
alone does not do anything.now()
multiple times is ok. The script will be loaded only once and the initialization script is executed only once tooinitialize
functionListen to script load events with
const unbind = SCRIPT.onStateChange(() => {
console.log(SCRIPT.state); // "pending" | "loading" | "ready"
});
This can be used to implement loading indicators.
There's a React hook for easy access to the script state from render:
import { useLazyScript } from "@valu/lazy-script/react";
function Component() {
const state = useLazyScript(SCRIPT);
}
FAQs
Lazy load 3rd party scripts
The npm package @valu/lazy-script receives a total of 3 weekly downloads. As such, @valu/lazy-script popularity was classified as not popular.
We found that @valu/lazy-script demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.