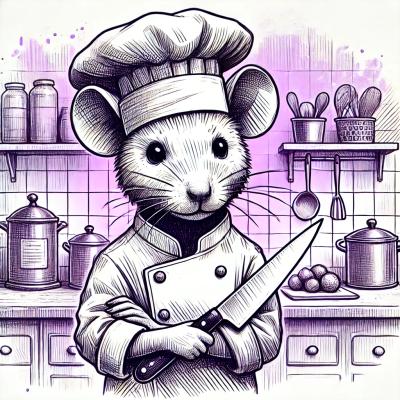
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@vertexvis/api-client-node
Advanced tools
If you're ready to integrate Vertex into your application, this is the place! For more background on the Vertex platform, start with our Developer Portal.
The Vertex REST API client for Node.js is generated using openapi-generator
, so it's always up-to-date. On top of the generated code, we've added a higher-level client and helpers in the ./client
directory.
If you're not an existing Vertex customer, sign up for a free account.
Install the client and export your credentials.
# Install client
npm install --save @vertexvis/api-client-node
# Export your Vertex REST API client ID and secret
export VERTEX_CLIENT_ID=[YOUR_CLIENT_ID]
export VERTEX_CLIENT_SECRET=[YOUR_CLIENT_SECRET]
Then, create a client and start using the Vertex API.
import { logError, prettyJson, VertexClient } from '@vertexvis/api-client-node';
const main = async () => {
try {
// Shown with default values
const client = await VertexClient.build({
basePath: 'https://platform.vertexvis.com',
client: {
id: process.env.VERTEX_CLIENT_ID,
secret: process.env.VERTEX_CLIENT_SECRET,
},
});
const getFilesRes = await client.files.getFiles({ pageSize: 1 });
console.log(prettyJson(getFilesRes.data));
} catch (error) {
logError(error, console.error);
}
};
main();
# Install dependencies
yarn
# Transpile TypeScript to JavaScript
yarn build
# Format code
yarn format
# Generate latest
yarn generate
# Generate using latest OpenAPI spec, version, and open GitHub PR
yarn push:version [patch|minor|major (default: patch)]
FAQs
The Vertex REST API client for Node.js.
The npm package @vertexvis/api-client-node receives a total of 121 weekly downloads. As such, @vertexvis/api-client-node popularity was classified as not popular.
We found that @vertexvis/api-client-node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.