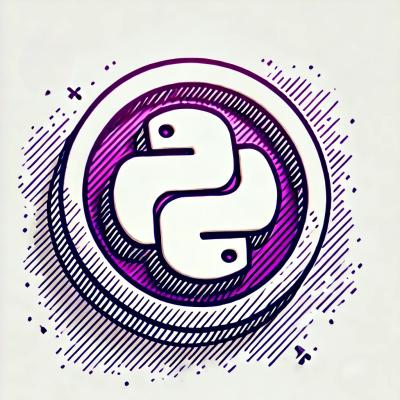
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@vue-storefront/checkout-com
Advanced tools
1. Open your theme directory and run: ``` yarn add @vue-storefront/checkout-com ``` If you are Developing Core of Vue Storefront Next you might need to add `@vue-storefront/checkout-com` to `useRawSource` attribute in one of `buildModules`: ```js ['@vue-s
yarn add @vue-storefront/checkout-com
If you are Developing Core of Vue Storefront Next you might need to add @vue-storefront/checkout-com
to useRawSource
attribute in one of buildModules
:
['@vue-storefront/nuxt', {
coreDevelopment: true,
useRawSource: {
dev: [
'@vue-storefront/commercetools',
'@vue-storefront/core',
'@vue-storefront/checkout-com'
],
prod: [
'@vue-storefront/commercetools',
'@vue-storefront/core',
'@vue-storefront/checkout-com'
]
}
}],
nuxt.config.js
modules
add:['@vue-storefront/checkout-com/nuxt', {
publicKey: 'pk_test_your-public-key',
secretKey: 'sk_test_your-secret-key',
ctApiUrl: 'https://your-commerctools-instance.com'
}],
useCko
:import { useCko } from '@vue-storefront/checkout-com';
useCko
returns:interface {
availableMethods: { name: string, [key: string]: any },
error: Error | null,
selectedPaymentMethod: CkoPaymentType,
savePaymentInstrument: boolean,
storedPaymentInstruments: PaymentInstrument[],
submitDisabled: ComputedRef<boolean>,
loadAvailableMethods: (cartId: string, email?: string): { id, apms },
initForm: (): void,
submitCardForm: (): void,
makePayment: ({ cartId, email, contextDataId }): Promise<Response | void>,
setPaymentInstrument: (token: string): void,
setSavePaymentInstrument: (newSavePaymentInstrument: boolean): void,
loadSavePaymentInstrument: (): boolean,
removePaymentInstrument: (customerId: string, paymentInstrument: string): Promise<void>,
loadStoredPaymentInstruments: (customerId: string): Promise<void>
}
In this step you need:
const { cart } = useCart();
const { setBillingDetails } = useCheckout();
const { isAuthenticated, user } = useUser();
const {
initForm,
loadAvailableMethods,
availableMethods,
submitDisabled,
storedPaymentInstruments,
loadStoredPaymentInstruments,
error
} = useCko();
setBillingDetails
to save billing address. So you will be able to fetch availableMethods
which base on your billing address (server-side)loadStoredPaymentInstruments
for logged in user to load stored payment instruments. They will be loaded to storedPaymentInstruments
array of PaymentInstrument
s. Caution: This interface is being used for storing credit cards currently. It might have different shape for stored different payment methods in the future. Interface:interface PaymentInstrument {
id: string;
type: string;
expiry_month: number;
expiry_year: number;
scheme: string;
last4: string;
fingerprint: string;
bin: string;
card_type: string;
card_category: string;
issuer: string;
issuer_country: string;
product_id: string;
product_type: string;
avs_check: string;
cvv_check: string;
payouts: string;
fast_funds: string;
payment_instrument_id: string;
}
Example of usage:
if (isAuthenticated.value && cart.value && cart.value.customerId) {
await loadStoredPaymentInstruments(cart.value.customerId);
}
loadAvailableMethods
- first argument is cartId (access it via cart.value.id
) - second for authenticated customer is an email (access it via user.value.email
). Then it will return interface { id, apms: Array<any> }
and set apms
inside availableMethods
. E.g:onMounted(async () => {
await loadAvailableMethods(cart.value.id, user.value && user.value.email);
})
initForm
. It mounts different payment handlers depends on arguments (check details below). If you are calling it after load component - use onMounted
to make sure DOM Element where it should be mounted already exists. Card's Frames will be mounted in DOM element with class card-frame
.interface PaymentMethods {
card?: boolean;
klarna?: boolean;
paypal?: boolean;
}
interface PaymentMethodsConfig {
card?: Omit<Configuration, 'publicKey'>;
klarna?: any;
paypal?: any;
}
const initForm = (initMethods: PaymentMethods = null, config: PaymentMethodsConfig = {}): void
initMethods
- if it is null
- method will try to mount handler for each supported payment method
{}
- nothing will be mounted{ card: true }
but it will still check whether it is supported or notconfig
allows to specify configuration for some payment handler, e.g. for card Frames we could use:
{
card: {
localization: 'es-ES'
}
}
This configuration will have bigger priority than one from nuxt.config.js
. The thing is you cannot overwrite is publicKey
there. Signature for Frames looks like that:
(params?: Omit<Configuration, 'publicKey'>): void
Unfortunately, Checkout.com is not sharing any component for Saved Cards. After using loadStoredPaymentInstruments
you can access an array of them via storedPaymentInstruments
. Show them to user in a way you want. To choose certain Stored Instrument call setPaymentInstrument(item.id)
where item
is single element of storePaymentInstruments
array.
When submitDisabled
changes to false - it means provided Card's data is proper and you could allow your user go forward. Card's token will be stored in localStorage for a moment.
Call submitCardForm
function on card form submit (only for Credit Card method - not necessary for Stored Payment Method). It requires mounted Frames
instance as it uses Frames.submitCard()
under the hood.
Then you need to make Payment
error
- contains error message from the response if you do not use 3ds or we have some server related issues. If the user just removed stored token from localStorage it will have There is no payment token
inside.
makePayment
- it proceeds with the payment and removes card token afterward. Returns Promise if succeed, or Promise if failed.
You should call makePayment
at first (remember to check if everything went ok):
// If it is guest
const payment = await makePayment({ cartId: cart.value.id });
// If it is customer
const payment = await makePayment({ cartId: cart.value.id, email: user.value && user.value.email });
// If you've already loaded available payment methods with same useCko composable instance
const payment = await makePayment();
if (!payment) return;
If there is any error, you can access it via error.value
. Otherwise, it will be nullish
If no errors, place an order:
const order = await placeOrder();
payment.data.redirect_url
contains 3DS Auth redirect url if it is required by bank. You have to support it:if (payment.data.redirect_url) {
window.location.href = payment.data.redirect_url;
return;
}
makePayment
method:success_url: `${window.location.origin}/cko/payment-success`,
failure_url: `${window.location.origin}/cko/payment-error`
It is important to set proper CKO's Payment Method in useCko
instance so it will be able to figure out proper payload to send in makePayment
. To do that:
import { useCko, CkoPaymentType } from '@vue-storefront/checkout-com'
// ...
const {
initForm,
loadAvailableMethods,
submitCardForm,
makePayment,
selectPaymentMethod, // Here
setPaymentInstrument,
setSavePaymentInstrument,
loadSavePaymentInstrument,
selectedPaymentMethod,
loadStoredPaymentInstruments,
removePaymentInstrument,
storedPaymentInstruments,
submitDisabled,
error
} = useCko();
Currently, these are available payment methods:
enum CkoPaymentType {
NOT_SELECTED = 0,
CREDIT_CARD = 1,
SAVED_CARD,
KLARNA, // Not supported yet
PAYPAL // Not supported yet
}
By default, selectPaymentMethod
equals CkoPaymentType.NOT_SELECTED
.
If user uses stored payment call setSavePaymentInstrument
and it will set selectPaymentMethod.value = CkoPaymentType.SAVED_CARD
setPaymentInstrument(item.id);
If user uses credit card use:
selectPaymentMethod.value = CkoPaymentType.CREDIT_CARD
useCko
composable shares savePaymentInstrument
ref and setSavePaymentInstrument
method for that purpose. It is also being stored in the localStorage and autoloaded in onMounted
hook. Remember to always use setSavePaymentInstrument
after savePaymentInstrument
update state in localStorage. E.g:
const {
initForm,
loadAvailableMethods,
submitCardForm,
makePayment,
selectPaymentMethod,
setPaymentInstrument,
setSavePaymentInstrument, // Save
loadSavePaymentInstrument, // Load
selectedPaymentMethod,
loadStoredPaymentInstruments,
removePaymentInstrument,
storedPaymentInstruments,
submitDisabled,
error
} = useCko();
const savePaymentInstrument = ref(loadSavePaymentInstrument());
<SfCheckbox
@change="setSavePaymentInstrument(savePaymentInstrument)"
v-model="savePaymentInstrument"
label="Save payment instrument"
name="savePaymentInstrument"
class="form__element"
/>
Checkout.com supports 3 payment methods - Credit Card, Klarna & Paypal. By default, module fetches SDK only for Credit Card (Frames). You can customize it with module's config paymentMethods
attribute. E.g:
['@vue-storefront/checkout-com/nuxt', {
// ...
paymentMethods: {
cc: true,
paypal: false,
klarna: true
}
}]
In nuxt.config.js
module's config you can use each attribute from this page, e.g:
['@vue-storefront/checkout-com/nuxt', {
// ...
card: {
localization: 'KO-KR',
styles: {
'card-number': {
color: 'red'
},
base: {
color: '#72757e',
fontSize: '19px',
minWidth: '60px'
},
invalid: {
color: 'red'
},
placeholder: {
base: {
color: 'cyan',
fontSize: '50px'
}
}
}
}
}]
localization
attribute must be a string or fulfill CustomLocalization
interface:
interface CustomLocalization {
cardNumberPlaceholder: string;
expiryMonthPlaceholder: string;
expiryYearPlaceholder: string;
cvvPlaceholder: string;
}
At first, you have to save billing address in your backend to do that. You can do it just after setBillingDetails
call from Creadit card component
step. Then you can easily use loadAvailableMethods
method. It requires reference as the first argument - which is cartId. E.g:
// Somewhere inside Vue3's setup method
const { cart } = useCart();
const { user } = useUser();
const { loadAvailableMethods, availableMethods } = useCko();
const { setBillingDetails } = useCheckout();
const handleFormSubmit = async () => {
await setBillingDetails(billingDetails.value, { save: true });
// If it is Guest
const response = await loadAvailableMethods(cart.value.id);
// If it is Customer
const response = await loadAvailableMethods(cart.value.id, user.value.email);
console.log('Server respond with ', response)
console.log('Array of available payment methods ', availableMethods)
};
Response might look like:
{
"id": "cid_XXX",
"apms": [
{
"name": "paypal",
"schema": "https://somewebsite.com/apms/paypal.json",
"show": true
}
]
}
availableMethods
might look like:
[
{
"name": "card"
},
{
"name": "klarna",
"some_key": "123"
},
{
"name": "paypal",
"some_key": "456"
}
]
useCko
composable shares removePaymentInstrument
method for that purpose. Keep in mind you use storedPaymentMethods[i].id
for payment but storedPaymentMethods[i].payment_instrument
for removing it from the vault. Use it like:
const {
removePaymentInstrument,
// ...
} = useCko();
const { isAuthenticated } = useUser();
const { cart } = useCart();
const removeMinePaymentInstrument = async (paymentInstrument: string): Promise<void> => {
if (isAuthenticated.value && cart.value && cart.value.customerId) {
await removePaymentInstrument(cart.value.customerId, paymentInstrument);
}
}
FAQs
### Stay connected
The npm package @vue-storefront/checkout-com receives a total of 2 weekly downloads. As such, @vue-storefront/checkout-com popularity was classified as not popular.
We found that @vue-storefront/checkout-com demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.