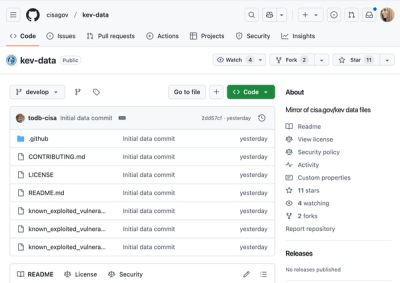
Security News
CISA Brings KEV Data to GitHub
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
@vue/babel-helper-vue-jsx-merge-props
Advanced tools
@vue/babel-helper-vue-jsx-merge-props is a utility package designed to help with merging props in Vue.js JSX. It simplifies the process of combining multiple sets of properties into a single set, which is particularly useful when dealing with JSX in Vue components.
Merging Props
This feature allows you to merge multiple sets of properties into one. In the example, `props1` and `props2` are merged, resulting in a single object that combines the classes and other properties.
const mergeProps = require('@vue/babel-helper-vue-jsx-merge-props');
const props1 = { class: 'btn', type: 'button' };
const props2 = { class: 'btn-primary', disabled: true };
const mergedProps = mergeProps([props1, props2]);
console.log(mergedProps); // { class: 'btn btn-primary', type: 'button', disabled: true }
lodash.merge is a function from the Lodash library that recursively merges own and inherited enumerable string keyed properties of source objects into the destination object. It is more general-purpose compared to @vue/babel-helper-vue-jsx-merge-props, which is specifically tailored for Vue.js JSX props.
deepmerge is a library that allows you to merge JavaScript objects without mutating them. It is similar to @vue/babel-helper-vue-jsx-merge-props in that it can combine multiple objects, but it is not specifically designed for Vue.js or JSX.
A package used internally by vue jsx transformer to merge props spread. It is required to merge some prop trees like this:
import mergeProps from '@vue/babel-helper-vue-jsx-merge-props'
const MyComponent = {
render(h) {
// original: <button onClick={$event => console.log($event)} {...{ on: { click: $event => doSomething($event) } }} />
return h(
'button',
mergeProps([
{
on: {
click: $event => console.log($event),
},
},
{
on: {
click: $event => doSomething($event),
},
},
]),
)
},
}
This tool is used internally and there is no reason for you to ever use it.
FAQs
Babel helper for Vue JSX spread
The npm package @vue/babel-helper-vue-jsx-merge-props receives a total of 612,682 weekly downloads. As such, @vue/babel-helper-vue-jsx-merge-props popularity was classified as popular.
We found that @vue/babel-helper-vue-jsx-merge-props demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.