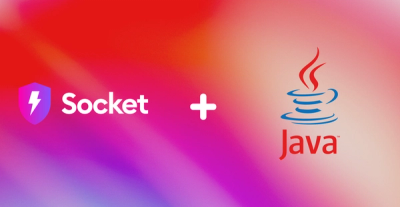
Product
Introducing Java Support in Socket
We're excited to announce that Socket now supports the Java programming language.
@vue/cli-plugin-typescript
Advanced tools
@vue/cli-plugin-typescript is a Vue CLI plugin that adds TypeScript support to Vue.js projects. It allows developers to write Vue components and other code in TypeScript, providing type safety and other benefits of TypeScript.
TypeScript Support
This feature allows you to add TypeScript support to your Vue.js project. By including the plugin in your Vue CLI configuration, you can start writing your Vue components and other code in TypeScript.
module.exports = {
plugins: [
'@vue/cli-plugin-typescript'
]
};
TypeScript Configuration
This feature provides a default TypeScript configuration file (tsconfig.json) that is optimized for Vue.js projects. It includes settings for module resolution, strict type checking, and paths for resolving modules.
{
"compilerOptions": {
"target": "esnext",
"module": "esnext",
"strict": true,
"jsx": "preserve",
"importHelpers": true,
"moduleResolution": "node",
"experimentalDecorators": true,
"esModuleInterop": true,
"allowSyntheticDefaultImports": true,
"sourceMap": true,
"baseUrl": ".",
"types": [
"webpack-env"
],
"paths": {
"@/*": [
"src/*"
]
},
"lib": [
"esnext",
"dom",
"dom.iterable",
"scripthost"
]
},
"include": [
"src/**/*.ts",
"src/**/*.tsx",
"src/**/*.vue"
],
"exclude": [
"node_modules"
]
}
TypeScript in Vue Components
This feature allows you to write Vue components using TypeScript. By setting the script tag's lang attribute to 'ts', you can leverage TypeScript's type checking and other features within your Vue components.
<template>
<div class="hello">
<h1>{{ msg }}</h1>
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
name: 'HelloWorld',
props: {
msg: String
}
});
</script>
<style scoped>
h1 {
color: #42b983;
}
</style>
TypeScript is a language for application-scale JavaScript development. It is a strict syntactical superset of JavaScript and adds optional static typing to the language. While @vue/cli-plugin-typescript integrates TypeScript into Vue projects, TypeScript itself is the core language that provides the type safety and other features.
vue-class-component is a library that allows you to define Vue components using TypeScript decorators. It provides a more class-based approach to defining components, which can be more familiar to developers coming from other object-oriented languages. It complements @vue/cli-plugin-typescript by providing a different syntax for writing components.
vue-property-decorator is a library that works with vue-class-component to provide decorators for Vue properties, such as @Prop, @Watch, and @Emit. It enhances the TypeScript experience in Vue projects by providing a more declarative way to define component properties and methods. It can be used alongside @vue/cli-plugin-typescript to provide additional functionality.
typescript plugin for vue-cli
Uses TypeScript + ts-loader
+ fork-ts-checker-webpack-plugin for faster off-thread type checking.
TypeScript can be configured via tsconfig.json
.
Since 3.0.0-rc.6
, typescript
is now a peer dependency of this package, so you can use a specific version of TypeScript by updating your project's package.json
.
This plugin can be used alongside @vue/cli-plugin-babel
. When used with Babel, this plugin will output ES2015 and delegate the rest to Babel for auto polyfill based on browser targets.
cache-loader is enabled by default and cache is stored in <projectRoot>/node_modules/.cache/ts-loader
.
thread-loader is enabled by default when the machine has more than 1 CPU cores. This can be turned off by setting parallel: false
in vue.config.js
.
parallel
should be set to false
when using Typescript in combination with non-serializable loader options, such as regexes, dates and functions. These options would not be passed correctly to ts-loader
which may lead to unexpected errors.
vue add typescript
config.rule('ts')
config.rule('ts').use('ts-loader')
config.rule('ts').use('babel-loader')
(when used alongside @vue/cli-plugin-babel
)config.rule('ts').use('cache-loader')
config.plugin('fork-ts-checker')
5.0.0-beta.1 (2021-05-14)
@vue/cli-service
@vue/cli-plugin-unit-mocha
@vue/cli-service
@vue/cli-plugin-typescript
@vue/cli-ui
@vue/cli-plugin-unit-jest
FAQs
typescript plugin for vue-cli
The npm package @vue/cli-plugin-typescript receives a total of 202,099 weekly downloads. As such, @vue/cli-plugin-typescript popularity was classified as popular.
We found that @vue/cli-plugin-typescript demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We're excited to announce that Socket now supports the Java programming language.
Security News
Socket detected a malicious Python package impersonating a popular browser cookie library to steal passwords, screenshots, webcam images, and Discord tokens.
Security News
Deno 2.0 is now available with enhanced package management, full Node.js and npm compatibility, improved performance, and support for major JavaScript frameworks.