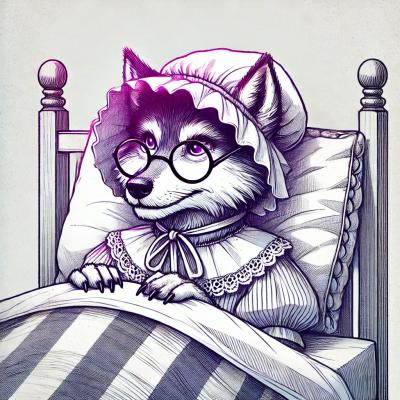
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@walletconnect/browser
Advanced tools
Browser Client for WalletConnect
For more details, read the documentation
yarn add @walletconnect/browser @walletconnect/qrcode-modal
# OR
npm install --save @walletconnect/browser @walletconnect/qrcode-modal
import WalletConnect from "@walletconnect/browser";
import WalletConnectQRCodeModal from "@walletconnect/qrcode-modal";
// Create a connector
const connector = new WalletConnect({
bridge: "https://bridge.walletconnect.org" // Required
});
// Check if connection is already established
if (!connector.connected) {
// create new session
connector.createSession().then(() => {
// get uri for QR Code modal
const uri = connector.uri;
// display QR Code modal
WalletConnectQRCodeModal.open(uri, () => {
console.log("QR Code Modal closed");
});
});
}
// Subscribe to connection events
connector.on("connect", (error, payload) => {
if (error) {
throw error;
}
// Close QR Code Modal
WalletConnectQRCodeModal.close();
// Get provided accounts and chainId
const { accounts, chainId } = payload.params[0];
});
connector.on("session_update", (error, payload) => {
if (error) {
throw error;
}
// Get updated accounts and chainId
const { accounts, chainId } = payload.params[0];
});
connector.on("disconnect", (error, payload) => {
if (error) {
throw error;
}
// Delete connector
});
// Draft transaction
const tx = {
from: "0xbc28Ea04101F03aA7a94C1379bc3AB32E65e62d3", // Required
to: "0x89D24A7b4cCB1b6fAA2625Fe562bDd9A23260359", // Required (for non contract deployments)
data: "0x", // Required
gasPrice: "0x02540be400", // Optional
gasLimit: "0x9c40", // Optional
value: "0x00", // Optional
nonce: "0x0114" // Optional
};
// Send transaction
connector
.sendTransaction(tx)
.then(result => {
// Returns transaction id (hash)
console.log(result);
})
.catch(error => {
// Error returned when rejected
console.error(error);
});
// Draft transaction
const tx = {
from: "0xbc28Ea04101F03aA7a94C1379bc3AB32E65e62d3", // Required
to: "0x89D24A7b4cCB1b6fAA2625Fe562bDd9A23260359", // Required (for non contract deployments)
data: "0x", // Required
gasPrice: "0x02540be400", // Optional
gasLimit: "0x9c40", // Optional
value: "0x00", // Optional
nonce: "0x0114" // Optional
};
// Sign transaction
connector
.signTransaction(tx)
.then(result => {
// Returns signed transaction
console.log(result);
})
.catch(error => {
// Error returned when rejected
console.error(error);
});
// Draft Message Parameters
const message = "My email is john@doe.com - 1537836206101"
const msgParams = [
convertUtf8ToHex(message) // Required
"0xbc28ea04101f03ea7a94c1379bc3ab32e65e62d3", // Required
];
// Sign personal message
connector
.signPersonalMessage(msgParams)
.then((result) => {
// Returns signature.
console.log(result)
})
.catch(error => {
// Error returned when rejected
console.error(error);
})
// Draft Message Parameters
const message = "My email is john@doe.com - 1537836206101";
const msgParams = [
"0xbc28ea04101f03ea7a94c1379bc3ab32e65e62d3", // Required
keccak256("\x19Ethereum Signed Message:\n" + len(message) + message)) // Required
];
// Sign message
connector
.signMessage(msgParams)
.then((result) => {
// Returns signature.
console.log(result)
})
.catch(error => {
// Error returned when rejected
console.error(error);
})
// Draft Message Parameters
const typedData = {
types: {
EIP712Domain: [
{ name: "name", type: "string" },
{ name: "version", type: "string" },
{ name: "chainId", type: "uint256" },
{ name: "verifyingContract", type: "address" }
],
Person: [
{ name: "name", type: "string" },
{ name: "account", type: "address" }
],
Mail: [
{ name: "from", type: "Person" },
{ name: "to", type: "Person" },
{ name: "contents", type: "string" }
]
},
primaryType: "Mail",
domain: {
name: "Example Dapp",
version: "1.0.0-beta",
chainId: 1,
verifyingContract: "0x0000000000000000000000000000000000000000"
},
message: {
from: {
name: "Alice",
account: "0xaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa"
},
to: {
name: "Bob",
account: "0xbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbb"
},
contents: "Hey, Bob!"
}
};
const msgParams = [
"0xbc28ea04101f03ea7a94c1379bc3ab32e65e62d3", // Required
typedData // Required
];
// Sign Typed Data
connector
.signTypedData(msgParams)
.then(result => {
// Returns signature.
console.log(result);
})
.catch(error => {
// Error returned when rejected
console.error(error);
});
// Draft Custom Request
const customRequest = {
id: 1337,
jsonrpc: "2.0",
method: "eth_signTransaction",
params: [
{
from: "0xbc28Ea04101F03aA7a94C1379bc3AB32E65e62d3",
to: "0x89D24A7b4cCB1b6fAA2625Fe562bDd9A23260359",
data: "0x",
gasPrice: "0x02540be400",
gasLimit: "0x9c40",
value: "0x00",
nonce: "0x0114"
}
]
};
// Send Custom Request
connector
.sendCustomRequest(customRequest)
.then(result => {
// Returns request result
console.log(result);
})
.catch(error => {
// Error returned when rejected
console.error(error);
});
import WalletConnect from "@walletconnect/browser";
import WalletConnectQRCodeModal from "@walletconnect/qrcode-modal";
// Create a connector
const connector = new WalletConnect({
bridge: "https://bridge.walletconnect.org" // Required
});
// Draft Instant Request
const instantRequest = {
id: 1,
jsonrpc: "2.0",
method: "eth_signTransaction",
params: [
{
from: "0xbc28ea04101f03ea7a94c1379bc3ab32e65e62d3",
to: "0x0000000000000000000000000000000000000000",
nonce: 1,
gas: 100000,
value: 0,
data: "0x0"
}
]
};
// Subscribe to Instant Request URI
connector.on("display_uri", (error, payload) => {
if (error) {
throw error;
}
const uri = payload.params[0].uri;
// Display QR Code Modal
WalletConnectQRCodeModal.open(uri, () => {
console.log("QR Code Modal closed");
});
});
// Create Instant Request
connector
.createInstantRequest(instantRequest)
.then(result => {
// Get Instant Request Result
console.log(result);
// Close QR Code Modal
WalletConnectQRCodeModal.close();
})
.catch(error => {
// Handle Error or Rejection
console.error(error);
});
FAQs
Browser Client for WalletConnect
The npm package @walletconnect/browser receives a total of 93 weekly downloads. As such, @walletconnect/browser popularity was classified as not popular.
We found that @walletconnect/browser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.