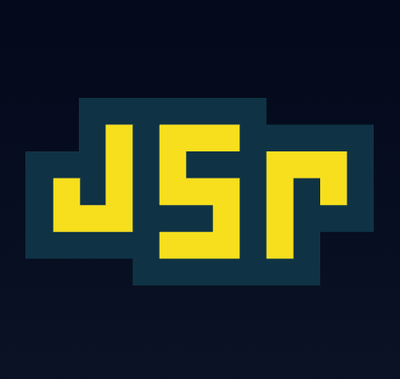
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@walletconnect/universal-provider
Advanced tools
@walletconnect/universal-provider is a JavaScript library that allows developers to integrate WalletConnect functionality into their applications. WalletConnect is an open protocol for connecting decentralized applications (dApps) to mobile wallets with QR code scanning or deep linking. This package provides a universal provider that supports multiple blockchain networks and wallet types, making it easier to manage wallet connections and interactions.
Initialize WalletConnect Provider
This code initializes the WalletConnect Universal Provider with the necessary configuration, including project ID, relay URL, and metadata for the dApp.
const { UniversalProvider } = require('@walletconnect/universal-provider');
const provider = new UniversalProvider({
projectId: 'your_project_id',
relayUrl: 'wss://relay.walletconnect.org',
metadata: {
name: 'My DApp',
description: 'My decentralized application',
url: 'https://mydapp.com',
icons: ['https://mydapp.com/icon.png']
}
});
Connect to Wallet
This code demonstrates how to connect to a wallet using the Universal Provider. It specifies the required namespaces, methods, chains, and events, and then logs the connected session.
async function connectWallet() {
const session = await provider.connect({
requiredNamespaces: {
eip155: {
methods: ['eth_sendTransaction', 'personal_sign'],
chains: ['eip155:1'],
events: ['chainChanged', 'accountsChanged']
}
}
});
console.log('Connected session:', session);
}
connectWallet();
Send Transaction
This code demonstrates how to send a transaction using the Universal Provider. It constructs a transaction object and sends it using the 'eth_sendTransaction' method.
async function sendTransaction() {
const tx = {
from: '0xYourAddress',
to: '0xRecipientAddress',
value: '0xAmountInHex',
gas: '0xGasLimitInHex'
};
const result = await provider.request({
method: 'eth_sendTransaction',
params: [tx]
});
console.log('Transaction result:', result);
}
sendTransaction();
Web3Modal is a library that allows developers to easily integrate multiple wallet providers into their dApps. It provides a modal for users to select their preferred wallet and supports various wallet providers like MetaMask, WalletConnect, and more. Compared to @walletconnect/universal-provider, Web3Modal offers a more user-friendly interface for wallet selection but may not provide as deep integration with WalletConnect-specific features.
Ethers.js is a library for interacting with the Ethereum blockchain and its ecosystem. It provides utilities for connecting to Ethereum nodes, managing wallets, and interacting with smart contracts. While it does not specifically focus on WalletConnect, it can be used in conjunction with WalletConnect to manage wallet connections and transactions. Ethers.js is more general-purpose and offers a wide range of Ethereum-related functionalities.
Universal Provider for WalletConnect Protocol
import { ethers } from "ethers";
import UniversalProvider from "@walletconnect/universal-provider";
// Initialize the provider
const provider = await UniversalProvider.init({
logger: "info",
relayUrl: "ws://<relay-url>",
projectId: "12345678",
metadata: {
name: "React App",
description: "React App for WalletConnect",
url: "https://walletconnect.com/",
icons: ["https://avatars.githubusercontent.com/u/37784886"],
},
client: undefined, // optional instance of @walletconnect/sign-client
});
// create sub providers for each namespace/chain
await provider.connect({
namespaces: {
eip155: {
methods: [
"eth_sendTransaction",
"eth_signTransaction",
"eth_sign",
"personal_sign",
"eth_signTypedData",
],
chains: ["eip155:80001"],
events: ["chainChanged", "accountsChanged"],
rpcMap: {
80001: "https://rpc.walletconnect.com?chainId=eip155:80001&projectId=<your walletconnect project id>",
},
},
pairingTopic: "<123...topic>", // optional topic to connect to
skipPairing: false, // optional to skip pairing ( later it can be resumed by invoking .pair())
},
});
// Create Web3 Provider
const web3Provider = new ethers.providers.Web3Provider(provider);
// Subscribe for pairing URI
provider.on("display_uri", (uri) => {
console.log(uri);
});
// Subscribe to session ping
provider.on("session_ping", ({ id, topic }) => {
console.log(id, topic);
});
// Subscribe to session event
provider.on("session_event", ({ event, chainId }) => {
console.log(event, chainId);
});
// Subscribe to session update
provider.on("session_update", ({ topic, params }) => {
console.log(topic, params);
});
// Subscribe to session delete
provider.on("session_delete", ({ id, topic }) => {
console.log(id, topic);
});
interface RequestArguments {
method: string;
params?: any[] | undefined;
}
// Send JSON RPC requests
/**
* @param payload
* @param chain - optionally specify which chain should handle this request
* in the format `<namespace>:<chainId>` e.g. `eip155:1`
*/
const result = await provider.request(payload: RequestArguments, chain: string | undefined);
const web3 = new Web3(provider);
// default chainId is the FIRST chain during setup
const chainId = await web3.eth.getChainId();
// set the default chain to 56
provider.setDefaultChain(`eip155:56`, rpcUrl?: string | undefined);
// get the updated default chainId
const updatedDefaultChainId = await web3.eth.getChainId();
FAQs
Universal Provider for WalletConnect Protocol
The npm package @walletconnect/universal-provider receives a total of 282,622 weekly downloads. As such, @walletconnect/universal-provider popularity was classified as popular.
We found that @walletconnect/universal-provider demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 12 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.