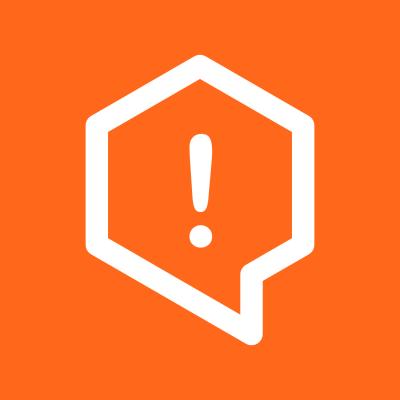
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@wdio/spec-reporter
Advanced tools
@wdio/spec-reporter is a WebdriverIO plugin that provides a detailed and formatted output of test results. It is designed to give a clear and concise summary of test execution, including information about passed, failed, and skipped tests. This reporter is particularly useful for developers and testers who want to quickly understand the outcome of their test suites.
Formatted Test Results
This feature provides a formatted output of test results, including stack traces for failed tests, summaries of failures and pending tests, and durations of test execution. The configuration options allow customization of what information is displayed.
const { SpecReporter } = require('@wdio/spec-reporter');
exports.config = {
reporters: ['spec'],
reporterOptions: {
spec: {
displayStacktrace: 'all',
displayFailuresSummary: true,
displayPendingSummary: true,
displaySuccessfulSpec: true,
displayFailedSpec: true,
displayPendingSpec: true,
displayDuration: true
}
}
};
Customizable Output
This feature allows customization of the symbols used in the output for passed, failed, and pending tests. This can help in making the test results more readable and aligned with the team's preferences.
const { SpecReporter } = require('@wdio/spec-reporter');
exports.config = {
reporters: ['spec'],
reporterOptions: {
spec: {
symbols: {
passed: '[PASS]',
failed: '[FAIL]',
pending: '[PEND]'
}
}
}
};
Integration with WebdriverIO
The @wdio/spec-reporter integrates seamlessly with WebdriverIO, making it easy to add to your existing WebdriverIO configuration. This feature ensures that the reporter works out of the box with WebdriverIO's test runner.
const { SpecReporter } = require('@wdio/spec-reporter');
exports.config = {
reporters: ['spec'],
reporterOptions: {
spec: {
displayStacktrace: 'summary'
}
}
};
Mochawesome is a reporter for the Mocha test framework that generates a visually appealing HTML/CSS report. It provides detailed information about test results, including screenshots and stack traces. Compared to @wdio/spec-reporter, mochawesome offers more visual customization and is specifically designed for Mocha.
Jasmine-spec-reporter is a custom reporter for the Jasmine test framework that provides a detailed and formatted output of test results. It offers similar functionalities to @wdio/spec-reporter, such as displaying stack traces and summaries of test results. However, it is tailored for use with Jasmine rather than WebdriverIO.
Karma-spec-reporter is a reporter for the Karma test runner that provides a concise and readable output of test results. It is similar to @wdio/spec-reporter in terms of providing formatted test results, but it is designed to work with the Karma test runner instead of WebdriverIO.
A WebdriverIO plugin to report in spec style.
The easiest way is to keep @wdio/spec-reporter
as a devDependency in your package.json
, via:
npm install @wdio/spec-reporter --save-dev
Instructions on how to install WebdriverIO
can be found here.
The following code shows the default wdio test runner configuration. Just add 'spec'
as a reporter
to the array.
// wdio.conf.js
module.exports = {
// ...
reporters: ['dot', 'spec'],
// ...
};
Provide custom symbols for passed
, failed
and or skipped
tests
Type: object
Default: {passed: '✓', skipped: '-', failed: '✖'}
[
"spec",
{
symbols: {
passed: '[PASS]',
failed: '[FAIL]',
},
},
]
By default the test results in Sauce Labs can only be viewed by a team member from the same team, not by a team member
from a different team. This options will enable sharable links
by default, which means that all tests that are executed in Sauce Labs can be viewed by everybody.
Just add sauceLabsSharableLinks: false
, as shown below, in the reporter options to disable this feature.
Type: boolean
Default: true
[
"spec",
{
sauceLabsSharableLinks: false,
},
]
Print only failed specs results.
Type: boolean
Default: false
[
"spec",
{
onlyFailures: true,
},
]
Set to true
to show console logs from steps in final report
Type: boolean
Default: false
[
"spec",
{
addConsoleLogs: true,
},
]
Set to true
to display test status realtime than just at the end of the run
Type: boolean
Default: false
[
"spec",
{
realtimeReporting: true,
},
]
Set to false
to disable [ MutliRemoteBrowser ... ]
preface in the reports.
Type: boolean
Default: true
[
"spec",
{
showPreface: false,
},
]
With it set to false
you will see output as:
Running: loremipsum (v50) on Windows 10
Session ID: foobar
» /foo/bar/loo.e2e.js
Foo test
green ✓ foo
green ✓ bar
» /bar/foo/loo.e2e.js
Bar test
green ✓ some test
red ✖ a failed test
red ✖ a failed test with no stack
and with true
(default) each line will be prefixed with the preface:
[loremipsum 50 Windows 10 #0-0] Running: loremipsum (v50) on Windows 10
[loremipsum 50 Windows 10 #0-0] Session ID: foobar
[loremipsum 50 Windows 10 #0-0]
[loremipsum 50 Windows 10 #0-0] » /foo/bar/loo.e2e.js
[loremipsum 50 Windows 10 #0-0] Foo test
[loremipsum 50 Windows 10 #0-0] green ✓ foo
[loremipsum 50 Windows 10 #0-0] green ✓ bar
[loremipsum 50 Windows 10 #0-0]
[loremipsum 50 Windows 10 #0-0] » /bar/foo/loo.e2e.js
[loremipsum 50 Windows 10 #0-0] Bar test
[loremipsum 50 Windows 10 #0-0] green ✓ some test
[loremipsum 50 Windows 10 #0-0] red ✖ a failed test
[loremipsum 50 Windows 10 #0-0] red ✖ a failed test with no stack
[loremipsum 50 Windows 10 #0-0]
Set to true
to display colored output in terminal
Type: boolean
Default: true
[
"spec",
{
color: true,
},
]
There are certain options you can set through environment variables:
FORCE_COLOR
If set to true, e.g. via FORCE_COLOR=0 npx wdio run wdio.conf.js
, all terminal coloring will be disabled.
v9.5.0 (2024-12-30)
webdriverio
webdriverio
wdio-sauce-service
wdio-cli
, wdio-jasmine-framework
, wdio-types
wdio-utils
wdio-types
, wdio-utils
, webdriverio
FAQs
A WebdriverIO plugin to report in spec style
The npm package @wdio/spec-reporter receives a total of 404,514 weekly downloads. As such, @wdio/spec-reporter popularity was classified as popular.
We found that @wdio/spec-reporter demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.