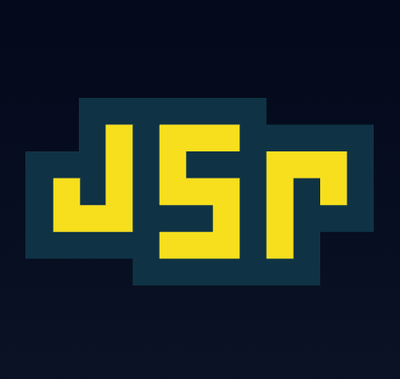
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@web3-onboard/react
Advanced tools
A collection of React hooks for implementing web3-onboard in to a React project
npm i @web3-onboard/react
import React from 'react'
import {
init,
useConnectWallet,
useSetChain,
useWallets
} from '@web3-onboard/react'
import injectedModule from '@web3-onboard/injected-wallets'
import coinbaseModule from '@web3-onboard/coinbase'
import trezorModule from '@web3-onboard/trezor'
import ledgerModule from '@web3-onboard/ledger'
import walletConnectModule from '@web3-onboard/walletconnect'
import portisModule from '@web3-onboard/portis'
import fortmaticModule from '@web3-onboard/fortmatic'
import torusModule from '@web3-onboard/torus'
import keepkeyModule from '@web3-onboard/keepkey'
const injected = injectedModule()
const coinbase = coinbaseModule()
const walletConnect = walletConnectModule()
const portis = portisModule({
apiKey: 'b2b7586f-2b1e-4c30-a7fb-c2d1533b153b'
})
const fortmatic = fortmaticModule({
apiKey: 'pk_test_886ADCAB855632AA'
})
const torus = torusModule()
const ledger = ledgerModule()
const keepkey = keepkeyModule()
const trezorOptions = {
email: 'test@test.com',
appUrl: 'https://www.blocknative.com'
}
const trezor = trezorModule(trezorOptions)
const web3Onboard = init({
wallets: [
injected,
coinbase,
ledger,
trezor,
walletConnect,
keepkey,
fortmatic,
portis,
torus
],
chains: [
{
id: '0x1',
token: 'ETH',
label: 'Ethereum Mainnet',
rpcUrl: 'https://mainnet.infura.io/v3/ababf9851fd845d0a167825f97eeb12b'
},
{
id: '0x3',
token: 'tROP',
label: 'Ethereum Ropsten Testnet',
rpcUrl: 'https://ropsten.infura.io/v3/ababf9851fd845d0a167825f97eeb12b'
},
{
id: '0x4',
token: 'rETH',
label: 'Ethereum Rinkeby Testnet',
rpcUrl: 'https://rinkeby.infura.io/v3/ababf9851fd845d0a167825f97eeb12b'
},
{
id: '0x89',
token: 'MATIC',
label: 'Matic Mainnet',
rpcUrl: 'https://matic-mainnet.chainstacklabs.com'
}
],
appMetadata: {
name: 'Blocknative',
icon: '<svg><svg/>',
description: 'Demo app for Onboard V2',
recommendedInjectedWallets: [
{ name: 'MetaMask', url: 'https://metamask.io' },
{ name: 'Coinbase', url: 'https://wallet.coinbase.com/' }
]
},
accountCenter: {
desktop: {
position: 'topRight',
enabled: true,
minimal: true
},
mobile: {
position: 'topRight',
enabled: true,
minimal: true
}
}
})
function App() {
const [{ wallet, connecting }, connect, disconnect] = useConnectWallet()
const [{ chains, connectedChain, settingChain }, setChain] = useSetChain()
const connectedWallets = useWallets()
return (
<div>
<button onClick={() => connect()}>
{connecting ? 'connecting' : 'connect'}
</button>
{wallet && (
<div>
<label>Switch Chain</label>
{settingChain ? (
<span>Switching chain...</span>
) : (
<select
onChange={({ target: { value } }) =>
console.log('onChange called') || setChain({ chainId: value })
}
value={connectedChain.id}
>
{chains.map(({ id, label }) => {
return <option value={id}>{label}</option>
})}
</select>
)}
<button onClick={() => disconnect(wallet)}>Disconnect Wallet</button>
</div>
)}
{connectedWallets.map(({ label, accounts }) => {
return (
<div>
<div>{label}</div>
<div>Accounts: {JSON.stringify(accounts, null, 2)}</div>
</div>
)
})}
</div>
)
}
export default App
init
The init
function must be called before any hooks can be used. The init
function just initializes web3-onboard
and makes it available for all hooks to use. For reference check out the initialization docs for @web3-onboard/core
useConnectWallet
This hook allows you to connect the user's wallet and track the state of the connection status and the wallet that is connected.
import { useConnectWallet } from '@web3-onboard/react'
type UseConnectWallet = (): [
{ wallet: WalletState | null; connecting: boolean },
(options: ConnectOptions) => Promise<void>,
(wallet: DisconnectOptions) => Promise<void>
]
type ConnectOptions = {
autoSelect?: string // wallet name to auto-select for user
}
type DisconnectOptions = {
label: string // wallet label
}
type WalletState = {
label: string
icon: string
provider: EIP1193Provider
accounts: Account[]
chains: ConnectedChain[]
instance?: unknown
}
const [
{
wallet, // the wallet that has been connected or null if not yet connected
connecting // boolean indicating if connection is in progress
},
connect, // function to call to initiate user to connect wallet
disconnect // function to call to with wallet<DisconnectOptions> to disconnect wallet
] = useConnectWallet()
useSetChain
This hook allows you to set the chain of a user's connected wallet, keep track of the current chain the user is connected to and the status of setting the chain. Passing in a wallet label will operate on that connected wallet, otherwise it will default to the last connected wallet.
import { useSetChain } from '@web3-onboard/react'
type UseSetChain = (
walletLabel?: string
): [
{
chains: Chain[]
connectedChain: ConnectedChain | null
settingChain: boolean
},
(options: SetChainOptions) => Promise<void>
]
type SetChainOptions = {
chainId: string
chainNamespace?: string
wallet?: WalletState['label']
}
const [
{
chains, // the list of chains that web3-onboard was initialized with
connectedChain, // the current chain the user's wallet is connected to
settingChain // boolean indicating if the chain is in the process of being set
},
setChain // function to call to initiate user to switch chains in their wallet
] = useSetChain()
useWallets
This hook allows you to track the state of all the currently connected wallets.
import { useWallets } from '@web3-onboard/react'
type UseWallets = (): WalletState[]
const connectedWallets = useWallets()
FAQs
A collection of React hooks for integrating Web3-Onboard in to React and Next.js projects. Web3-Onboard makes it simple to connect Ethereum hardware and software wallets to your dapp. Features standardised spec compliant web3 providers for all supported w
We found that @web3-onboard/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.