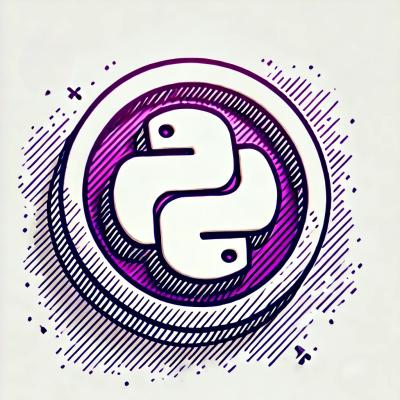
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@web3-onboard/vue
Advanced tools
A collection of Vue Composables for integrating Web3-Onboard in to a Vue or Nuxt project. Web3-Onboard makes it simple to connect Ethereum hardware and software wallets to your dapp. Features standardized spec compliant web3 providers for all supported wa
A collection of composable functions for implementing web3-onboard in to a Vue project; compatible both with Vue 2 + composition-api and Vue 3
NPM
npm i @web3-onboard/vue @web3-onboard/injected-wallets ethers
Yarn
yarn add @web3-onboard/vue @web3-onboard/injected-wallets ethers
import { init } from '@web3-onboard/vue'
import injectedModule from '@web3-onboard/injected-wallets'
const injected = injectedModule()
// Only one RPC endpoint required per chain
const rpcAPIKey = '<INFURA_KEY>' || '<ALCHEMY_KEY>'
const rpcUrl = `https://eth-mainnet.g.alchemy.com/v2/${rpcAPIKey}` || `https://mainnet.infura.io/v3/${rpcAPIKey}`
const web3Onboard = init({
wallets: [injected],
chains: [
{
id: '0x1',
token: 'ETH',
label: 'Ethereum Mainnet',
rpcUrl
}
]
})
const { wallets, connectWallet, disconnectConnectedWallet, connectedWallet } =
useOnboard()
if (connectedWallet) {
// if using ethers v6 this is:
// ethersProvider = new ethers.BrowserProvider(wallet.provider, 'any')
const ethersProvider = new ethers.providers.Web3Provider(
connectedWallet.provider,
'any'
)
// ..... do stuff with the provider
}
init
The init
function initializes web3-onboard
and makes it available to the useOnboard()
composable. For references check out the initialization docs for @web3-onboard/core
import { init } from '@web3-onboard/vue'
import injectedModule from '@web3-onboard/injected-wallets'
const injected = injectedModule()
const infuraKey = '<INFURA_KEY>'
const rpcUrl = `https://mainnet.infura.io/v3/${infuraKey}`
const web3Onboard = init({
wallets: [injected],
chains: [
{
id: '0x1',
token: 'ETH',
label: 'Ethereum Mainnet',
rpcUrl
}
]
})
useOnboard
useOnboard
must be used after the init
function has been called - it will return an object that can be destructured to obtain the following reactive variables and functions:
import { useOnboard } from '@web3-onboard/vue'
// Use the composable
const onboard = useOnboard()
// Or destructure it
const { wallets, connectWallet, disconnectConnectedWallet, connectedWallet } =
useOnboard()
// do stuff
connectWallet
Function to open the onboard modal and connect to a wallet provider. For reference check out the connecting a wallet for @web3-onboard/core
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { connectWallet } = useOnboard()
const connect = async () => connectWallet()
return { connect }
}
}
</script>
<template>
<button type="button" @click="connect">Connect to a Wallet</button>
</template>
connectedChain
Computed property that contains the current chain to which connectedWallet
is connected
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { connectedChain } = useOnboard()
return { connectedChain }
}
}
</script>
<template>
<span>Connected Chain: {{ connectedChain.id }}</span>
</template>
connectedWallet
Computed property that contains the latest connected wallet
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { connectedWallet } = useOnboard()
return { connectedWallet }
}
}
</script>
<template>
<span>Connected Wallet: {{ connectedWallet.label }}</span>
</template>
connectingWallet
Readonly boolean ref that tracks the state of the wallet connection status
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { connectingWallet } = useOnboard()
return { connectingWallet }
}
}
</script>
<template>
<span v-if="connectingWallet">Connecting...</span>
</template>
disconnectWallet
Function to disconnect a specific wallet
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { disconnectWallet } = useOnboard()
const disconnect = async () => disconnectWallet('MetaMask')
return { disconnect }
}
}
</script>
<template>
<button type="button" @click="disconnect">Disconnect MetaMask</button>
</template>
disconnectConnectedWallet
Function to disconnect the connectedWallet
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { disconnectConnectedWallet } = useOnboard()
return { disconnectConnectedWallet }
}
}
</script>
<template>
<button type="button" @click="disconnectConnectedWallet">
Disconnect connectedWallet
</button>
</template>
getChain
Function that returns the current chain a wallet is connected to
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { getChain } = useOnboard()
return { getChain }
}
}
</script>
<template>
<span>MetaMask is connected to: {{ getChain('MetaMask') }}</span>
</template>
setChain
Function to set the chain of a wallet
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { setChain } = useOnboard()
const set = () => setChain({ wallet: 'MetaMask', chainId: '0x1' })
return { set }
}
}
</script>
<template>
<button type="button" @click="set">Set MetaMask chain to mainnet</button>
</template>
settingChain
Readonly boolean ref that tracks the status of setting the chain
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { settingChain } = useOnboard()
return { settingChain }
}
}
</script>
<template>
<span v-if="settingChain">Setting chain...</span>
</template>
wallets
Readonly ref that contains every wallet that has been connected
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { wallets } = useOnboard()
return { wallets }
}
}
alreadyConnectedWallets
Readonly ref that contains every wallet that user connected to in the past; useful to reconnect wallets automatically after a reload
vue
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { alreadyConnectedWallets } = useOnboard()
return { alreadyConnectedWallets }
}
}
</script>
<template>
<div v-for="wallet in wallets">
<span>Label: {{wallet.label}}</span>
</div>
</template>
lastConnectedTimestamp
Readonly ref that contains the last time that the user connected a wallet in milliseconds
vue
<script>
import { useOnboard } from '@web3-onboard/vue'
export default {
setup() {
const { lastConnectedTimestamp } = useOnboard()
return { lastConnectedTimestamp }
}
}
</script>
<template>
<span>Your last connection timestamp was: {{ lastConnectedTimestamp }}</span>
</template>
FAQs
A collection of Vue Composables for integrating Web3-Onboard in to a Vue or Nuxt project. Web3-Onboard makes it simple to connect Ethereum hardware and software wallets to your dapp. Features standardized spec compliant web3 providers for all supported wa
We found that @web3-onboard/vue demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.