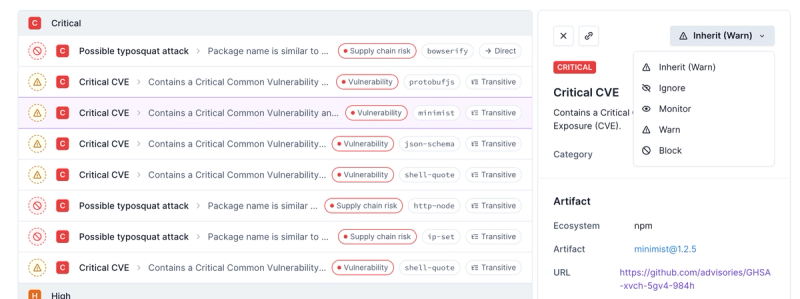
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@webassemblyjs/helper-wasm-bytecode
Advanced tools
Package description
The @webassemblyjs/helper-wasm-bytecode package is part of the WebAssemblyJS project, which provides tools for compiling, decompiling, and manipulating WebAssembly (Wasm) binaries. This specific package offers utilities for dealing with Wasm bytecode, such as parsing and generating bytecode.
Parsing Wasm Bytecode
This feature allows you to parse WebAssembly bytecode into a more readable and manipulable abstract syntax tree (AST) format. The code sample demonstrates how to read a Wasm file from the filesystem, parse it into an AST using the decode function, and then log the AST to the console.
"use strict";\nconst { decode } = require('@webassemblyjs/wasm-parser');\nconst fs = require('fs');\n\nconst wasmBuffer = fs.readFileSync('path/to/your.wasm');\nconst ast = decode(wasmBuffer);\nconsole.log(ast);
Generating Wasm Bytecode
This feature enables the generation of WebAssembly bytecode from an AST. The code sample shows how to read a Wasm file, parse it into an AST, generate new bytecode from the AST using the encode function, and then write the new bytecode to a file.
"use strict";\nconst { encode } = require('@webassemblyjs/wasm-gen');\nconst fs = require('fs');\nconst { decode } = require('@webassemblyjs/wasm-parser');\n\nconst wasmBuffer = fs.readFileSync('path/to/your.wasm');\nconst ast = decode(wasmBuffer);\nconst newWasmBuffer = encode(ast);\nfs.writeFileSync('path/to/output.wasm', newWasmBuffer);
The wabt package is a Node.js binding to the WebAssembly Binary Toolkit. It provides a set of utilities for working with WebAssembly binaries, including parsing, validation, and conversion between Wasm and WAT (WebAssembly Text Format). Compared to @webassemblyjs/helper-wasm-bytecode, wabt offers a broader set of tools directly tied to the official WABT toolkit, making it more comprehensive for certain tasks.
AssemblyScript compiles a strict subset of TypeScript to WebAssembly. While not a direct alternative for manipulating Wasm bytecode, AssemblyScript is relevant for developers looking to write high-level code that compiles down to WebAssembly. In contrast, @webassemblyjs/helper-wasm-bytecode is more focused on the manipulation of existing Wasm binaries.
FAQs
WASM's Bytecode constants
The npm package @webassemblyjs/helper-wasm-bytecode receives a total of 21,956,060 weekly downloads. As such, @webassemblyjs/helper-wasm-bytecode popularity was classified as popular.
We found that @webassemblyjs/helper-wasm-bytecode demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.