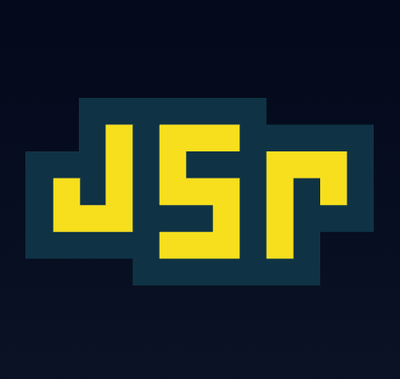
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@workos-inc/node
Advanced tools
@workos-inc/node is an SDK for integrating with WorkOS, a platform that provides enterprise-ready features such as Single Sign-On (SSO), Directory Sync, and Audit Logs. This package allows developers to easily integrate these features into their Node.js applications.
Single Sign-On (SSO)
This feature allows you to generate an SSO authorization URL for a specified provider, such as Google OAuth. The code sample demonstrates how to initialize the WorkOS client and generate an SSO URL.
const { WorkOS } = require('@workos-inc/node');
const workos = new WorkOS('your_api_key');
async function getSSOUrl() {
const ssoUrl = await workos.sso.getAuthorizationURL({
clientID: 'your_client_id',
redirectURI: 'your_redirect_uri',
state: 'optional_state',
provider: 'GoogleOAuth'
});
console.log(ssoUrl);
}
getSSOUrl();
Directory Sync
This feature allows you to list all directories that have been synchronized with WorkOS. The code sample demonstrates how to initialize the WorkOS client and list the directories.
const { WorkOS } = require('@workos-inc/node');
const workos = new WorkOS('your_api_key');
async function listDirectories() {
const directories = await workos.directorySync.listDirectories();
console.log(directories);
}
listDirectories();
Audit Logs
This feature allows you to create an audit log event for tracking user actions. The code sample demonstrates how to initialize the WorkOS client and create an audit log event.
const { WorkOS } = require('@workos-inc/node');
const workos = new WorkOS('your_api_key');
async function createAuditLogEvent() {
const event = await workos.auditLogs.createEvent({
organization: 'your_organization_id',
event: {
action: 'user.login',
occurred_at: new Date().toISOString(),
actor: {
id: 'user_id',
type: 'user'
},
targets: [{
id: 'target_id',
type: 'user'
}],
context: {
location: '127.0.0.1',
user_agent: 'Mozilla/5.0'
}
}
});
console.log(event);
}
createAuditLogEvent();
Passport is a popular authentication middleware for Node.js that supports various authentication strategies, including OAuth and SAML. Unlike @workos-inc/node, Passport focuses solely on authentication and does not provide additional enterprise features like Directory Sync or Audit Logs.
Auth0 is a comprehensive identity management platform that offers features like SSO, user management, and multifactor authentication. It is similar to @workos-inc/node in terms of providing enterprise-ready authentication solutions, but it also includes additional features like user management and multifactor authentication.
Okta is an identity and access management service that provides SSO, multifactor authentication, and user management. It is similar to @workos-inc/node in offering enterprise authentication solutions but also includes extensive user management and security features.
The WorkOS library for Node.js provides convenient access to the WorkOS API from applications written in server-side JavaScript.
See the API Reference for Node.js usage examples.
Node 16 or higher.
Install the package with:
yarn add @workos-inc/node
To use the library you must provide an API key, located in the WorkOS dashboard, as an environment variable WORKOS_API_KEY
:
WORKOS_API_KEY="sk_1234"
Or, you can set it on your own before your application starts:
import { WorkOS } from '@workos-inc/node';
const workos = new WorkOS('sk_1234');
For our SDKs WorkOS follows a Semantic Versioning (SemVer) process where all releases will have a version X.Y.Z (like 1.0.0) pattern wherein Z would be a bug fix (e.g., 1.0.1), Y would be a minor release (1.1.0) and X would be a major release (2.0.0). We permit any breaking changes to only be released in major versions and strongly recommend reading changelogs before making any major version upgrades.
WorkOS has features in Beta that can be accessed via Beta releases. We would love for you to try these and share feedback with us before these features reach general availability (GA). To install a Beta version, please follow the installation steps above using the Beta release version.
Note: there can be breaking changes between Beta versions. Therefore, we recommend pinning the package version to a specific version. This way you can install the same version each time without breaking changes unless you are intentionally looking for the latest Beta version.
We highly recommend keeping an eye on when the Beta feature you are interested in goes from Beta to stable so that you can move to using the stable version.
FAQs
A Node wrapper for the WorkOS API
The npm package @workos-inc/node receives a total of 335,968 weekly downloads. As such, @workos-inc/node popularity was classified as popular.
We found that @workos-inc/node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.