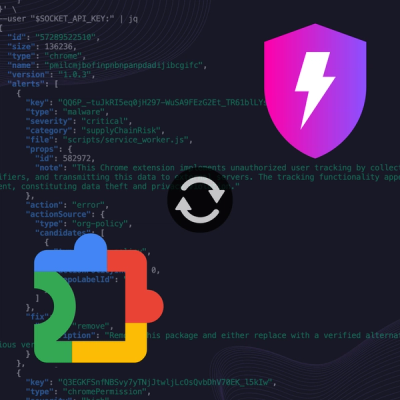
Product
Socket Now Protects the Chrome Extension Ecosystem
Socket is launching experimental protection for Chrome extensions, scanning for malware and risky permissions to prevent silent supply chain attacks.
@yworks/optimizer
Advanced tools
Use this tool to obfuscate and optimize your yFiles for HTML applications.
The optimizer will obfuscate the public API of the yFiles module files, as well as yFiles API usages in application sources.
Note that this tool is meant to be used in production builds. The resulting yFiles module files will be tailor-made to the yFiles API usages in the application sources that were processed along with the yFiles modules.
We highly recommend obfuscating the yFiles for HTML library prior to deploying your application to a public web server to reduce the download size of the library for the end user. Note that, at the time of writing, you are not required to use obfuscation.
The optimizer might wrongly replace non-yFiles API calls if they have the same name as a member of the yFiles API. To avoid this, the optimizer comes with a list of common JavaScript API names that it should not change in any case. If the optimizer erroneously replaces non-yFiles calls in your code, there are two possibilities to prevent this:
Use scope-local @yjs:keep
comments to exclude these members from obfuscation in a particular scope. This is the preferred approach, because the excluded names will still be obfuscated in yFiles modules and all other scopes.
// @yjs:keep = install,apply
function my() {
// "install" and "apply" will not be obfuscated within this scope
someAPI.install()
someAPI.apply()
}
// @yjs:keep
function my() {
// No names will be obfuscated within this scope at all
//...
}
Add the member to the blacklist that can be passed as an option to the optimizer. This will prevent the passed names from being obfuscated in the yFiles modules and all application sources.
safeMode
(default: false
): Enable safeMode
to prevent the optimizer from adjusting any names in non-yFiles code.
The default behavior of the optimizer is to obfuscate the names of all yFiles library members and adjust the corresponding occurrences in your source code. When you use a third-party library that uses member names that also exist in the yFiles API, it can happen that these third-party library calls in your sources are accidentally renamed. In general, we recommend adding these member names to the optimizer's blacklist
, or use Obfuscation Excludes to prevent the optimizer from renaming them. However, if it's hard to find out which
exact member names would have to be excluded from obfuscation in your project, you can enable safeMode
to prevent renaming of any yFiles members that are used in your code. That way, only unused yFiles members will be obfuscated in the yFiles library modules and your sources will be left untouched.
blacklist
(default: []
): An array of names that should never be renamed by the optimizer. See Obfuscation Excludes
logLevel
(default: "info"
): One of "error", "warn", "info", "verbose", "debug", "silly".
Note that with logLevel "debug" or finer, a yworks-optimizer-mappings.json
file will be created in the working directory that contains the mappings of unobfuscated to obfuscated yFiles member names. These mappings can help to debug the obfuscated code.
obfuscateBabelSafePublicFields
(default: true
) Whether the optimizer should try to obfuscate calls of babel's _defineProperty()
helper. With this option enabled, the optimizer will try to detect usages like _defineProperty(item, "propertyName", value)
and rename the "propertyName"
if it matches a yFiles member. Note that this behavior could erroneously rename strings in your application. If you find unwanted obfuscated strings in the generated output, try disabling this option. You can also set the logLevel
to "debug"
and watch for "Renaming possible babel defineProperty call" messages. For details about configuring the babel output of public class fields, please see https://babeljs.io/docs/en/assumptions#setpublicclassfields
To use the optimizer for a Vite production build, add it to the vite config:
import { defineConfig } from 'vite'
import optimizer from '@yworks/optimizer/rollup-plugin'
export default defineConfig(({ mode }) => {
const isProduction = mode === 'production'
return {
plugins: [
isProduction &&
optimizer({
logLevel: 'info'
})
]
}
})
To use it in a pure rollup config:
import optimizer from '@yworks/optimizer/rollup-plugin'
const isProduction = process.env.NODE_ENV === 'production'
export default {
input: 'src/main.js',
output: {
file: 'bundle.js',
format: 'cjs'
},
plugins: [
isProduction &&
optimizer({
logLevel: 'info'
})
]
}
To use the optimizer as a webpack plugin, add the plugin the webpack config:
const OptimizerPlugin = require('@yworks/optimizer/webpack-plugin')
module.exports = {
plugins: [
new OptimizerPlugin({
blacklist: ['install', 'apply'],
logLevel: 'info',
ignore: ['**/lib/external/**/*.js', /exclude[/\\].*\.js/]
})
]
}
Options supported by the webpack and rollup plugins in addition to the optimizer options explained above:
ignore
An array of minimatch-glob patterns or regexps specifying files that should be ignored by the optimizer. Note that the path separators are not normalized for regexp matching, i.e., on windows, /\\ignoreme.js/
would match, but /\/ignoreme.js/
would not.
This option can be used when some source files contain language features that are not supported by the optimizer (e.g. dynamic imports).
Use this option to ignore only files that do not contain any yFiles code. If the ignored files contain yFiles code, this code will not work with the optimized library.
shouldOptimize
An function that determines whether a particular module should be optimized. The default shouldOptimize
function will exclude all node_modules from optimization.
Example:
// webpack.config.js
const WebpackOptimizerPlugin = require('@yworks/optimizer/webpack-plugin')
new WebpackOptimizerPlugin({
shouldOptimize(module) {
return (
/node-module-that-uses-yfiles/.test(module.resource) ||
!/node_modules/.test(module.resource)
)
}
})
// rollup.config.mjs
import RollupOptimizerPlugin from '@yworks/optimizer/rollup-plugin'
new RollupOptimizerPlugin({
shouldOptimize({ id }) {
return id.includes('node-module-that-uses-yfiles') || !id.includes('node_modules')
}
})
const { optimize } = require('@yworks/optimizer')
// libModules: [{source: sourceOfYFilesModuleA}, ...]
// sourceFiles: [{source: appSourceA}, ...]
const optimized = optimize(libModules, sourceFiles, {
blacklist: [],
logLevel: 'warn'
})
// optimized:
// {
// libModules: [{
// source: sourceOfYFilesModuleA
// result: optimizedYFilesModuleA
// }, ...]
// sourceFiles: [{
// source: appSourceA
// result: optimizedAppSourceA
// }, ...]
// }
The optimized sources are attached to the same objects passed to the optimizer, so additional information (e.g. destination paths) on these objects is not lost.
Please see your yFiles for HTML package for example usages of the optimizer.
FAQs
yFiles for HTML obfuscation and optimization tool
The npm package @yworks/optimizer receives a total of 1,010 weekly downloads. As such, @yworks/optimizer popularity was classified as popular.
We found that @yworks/optimizer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket is launching experimental protection for Chrome extensions, scanning for malware and risky permissions to prevent silent supply chain attacks.
Product
Add secure dependency scanning to Claude Desktop with Socket MCP, a one-click extension that keeps your coding conversations safe from malicious packages.
Product
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.