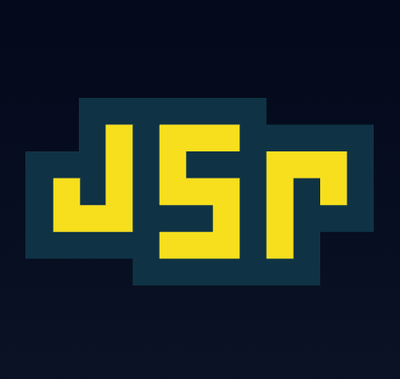
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@zag-js/core
Advanced tools
@zag-js/core is a state management library designed to help developers build complex, interactive user interfaces with ease. It provides a set of tools to manage state machines and statecharts, making it easier to handle UI states and transitions in a predictable and maintainable way.
State Machines
This feature allows you to define state machines with states and transitions. The example demonstrates a simple toggle machine with two states: 'inactive' and 'active'.
const { createMachine } = require('@zag-js/core');
const toggleMachine = createMachine({
id: 'toggle',
initial: 'inactive',
states: {
inactive: {
on: { TOGGLE: 'active' }
},
active: {
on: { TOGGLE: 'inactive' }
}
}
});
console.log(toggleMachine.initialState.value); // 'inactive'
Statecharts
Statecharts extend state machines by adding hierarchical states and parallel states. The example shows a traffic light statechart with three states: 'green', 'yellow', and 'red'.
const { createMachine } = require('@zag-js/core');
const lightMachine = createMachine({
id: 'light',
initial: 'green',
states: {
green: {
on: { TIMER: 'yellow' }
},
yellow: {
on: { TIMER: 'red' }
},
red: {
on: { TIMER: 'green' }
}
}
});
console.log(lightMachine.initialState.value); // 'green'
Contextual State
This feature allows you to manage contextual state within your state machines. The example demonstrates a counter machine with an initial count of 0 and actions to increment and decrement the count.
const { createMachine } = require('@zag-js/core');
const counterMachine = createMachine({
id: 'counter',
initial: 'active',
context: { count: 0 },
states: {
active: {
on: {
INCREMENT: { actions: 'increment' },
DECREMENT: { actions: 'decrement' }
}
}
}
}, {
actions: {
increment: (context) => context.count++,
decrement: (context) => context.count--
}
});
console.log(counterMachine.initialState.context.count); // 0
XState is a popular library for managing state machines and statecharts in JavaScript. It offers a rich set of features for defining and interpreting state machines, and it integrates well with various frameworks like React, Vue, and Angular. Compared to @zag-js/core, XState has a larger community and more extensive documentation.
Robot3 is a lightweight state machine library for JavaScript. It focuses on simplicity and ease of use, making it a good choice for smaller projects or developers who prefer a minimalistic approach. While it may not have as many features as @zag-js/core, it provides a straightforward API for managing state machines.
Redux is a widely-used state management library for JavaScript applications, particularly in the React ecosystem. While it is not specifically designed for state machines, it can be used to manage complex state logic through middleware and reducers. Compared to @zag-js/core, Redux offers a more general-purpose approach to state management.
This package contains a minimal implementation of XState FSM for finite state machines with addition of extra features we need for our components.
setTimeout
)setInterval
)and
, or
, not
)To better understand the state machines, we strongly recommend going though the xstate docs and videos. It'll give you the foundations you need.
Installation
npm i @zag-js/core
# or
yarn add @zag-js/core
Usage (machine):
import { createMachine } from "@zag-js/core"
const toggleMachine = createMachine({
id: "toggle",
initial: "inactive",
states: {
inactive: { on: { TOGGLE: "active" } },
active: { on: { TOGGLE: "inactive" } },
},
})
toggleMachine.start()
console.log(toggleMachine.state.value) // => "inactive"
toggleMachine.send("TOGGLE")
console.log(toggleMachine.state.value) // => "active"
toggleMachine.send("TOGGLE")
console.log(toggleMachine.state.value) // => "inactive"
Usage (service):
import { createMachine } from "@zag-js/core"
const toggleMachine = createMachine({...})
toggleMachine.start()
toggleService.subscribe((state) => {
console.log(state.value)
})
toggleService.send("TOGGLE")
toggleService.send("TOGGLE")
toggleService.stop()
createMachine(config, options)
Creates a new finite state machine from the config.
Argument | Type | Description |
---|---|---|
config | object (see below) | The config object for creating the machine. |
options | object (see below) | The optional options object. |
Returns:
A Machine
, which provides:
machine.initialState
: the machine's resolved initial statemachine.start()
: the function to start the machine in the specified initial state.machine.stop()
: the function to stop the machine completely. It also cleans up all scheduled actions and timeouts.machine.transition(state, event)
: a transition function that returns the next state given the current state
and
event
. It also performs any delayed, entry or exit side-effects.machine.send(event)
: a transition function instructs the machine to execute a transition based on the event.machine.onTransition(fn)
: a function that gets called when the machine transition function instructs the machine to
execute a transition based on the event.machine.onChange(fn)
: a function that gets called when the machine's context value changes.machine.state
: the state object that describes the machine at a specific point in time. It contains the following
properties:
value
: the current state valuepreviousValue
: the previous state valueevent
: the event that triggered the transition to the current statenextEvents
: the list of events the machine can respond to at its current statetags
: the tags associated with this statedone
: whehter the machine that reached its final statecontext
: the current context valuematches(...)
: a function used to test whether the current state matches one or more state valuesThe machine config has this schema:
id
(string) - an identifier for the type of machine this is. Useful for debugging.context
(object) - the extended state data that represents quantitative data (string, number, objects, etc) that can
be modified in the machine.initial
(string) - the key of the initial state.states
(object) - an object mapping state names (keys) to stateson
(object) - an global mapping of event types to transitions. If specified, this event will called if the state
node doesn't handle the emitted event.on
(object) - an object mapping event types (keys) to transitionsString syntax:
{ target: stateName }
Object syntax:
target
(string) - the state name to transition to.actions
(Action | Action[]) - the action(s) to execute when this transition is taken.guard
(Guard) - the condition (predicate function) to test. If it returns true
, the transition will be taken.actions?
(object) - a lookup object for your string actions.guards?
(object) - a lookup object for your string guards specified as guard
in the machine.activities?
(object) - a lookup object for your string activities.delays?
(object) - a lookup object for your string delays used in after
and every
config.The action function to execute while transitioning from one state to another. It takes the following arguments:
context
(any) - the machine's current context
.event
(object) - the event that caused the action to be executed.FAQs
A minimal implementation of xstate fsm for UI machines
The npm package @zag-js/core receives a total of 380,734 weekly downloads. As such, @zag-js/core popularity was classified as popular.
We found that @zag-js/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.