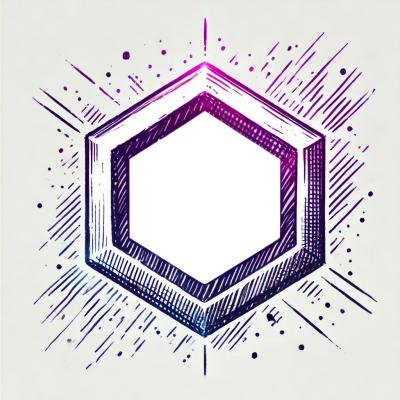
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Comments is an abstraction layer for MongoDB that allows for easily providing some commenting functionality.
new Comments(options)
Creates a new Comments object. This means, a connection to MongoDB is set up.
options
is an object that defines some DB connection parameters.
The default options are as follows:
{
host: 'localhost', // - hostname of the server where mongodb is
// running
port: 27017, // - port that is used by mongodb
name: 'website', // - name of the mongodb database
collection: 'comments' // - name of the collection that contains the
// comments
}
comments.saveComment(res, comment, saved)
Adds a new or updates a comment in the collection depending on if there already
is a comment with the same _id
property. If comment
does not define an
_id
property, a new comment is created.
res
is a string defining the resource the comment belongs to.comment
is an object that defines a comment. It is stored directly into
the collection. Any prior parsing is up to you. comment.res
should be
defined for the later use of comments.getComments
.saved
is a callback function that takes three arguments (error, comment, action)
.
error
is an Error
object, when an error occurred, otherwise it is
null
. comment
is the saved comment object. action
is a string. It can
either be 'create'
, 'update'
or null
, if an error occured.comments.getComments(res, [[properties,] options,] received)
Gives access to the comments of a resource.
res
is a string defining the resource that contains the comments that you
are looking for. If res
is null
, all comments in the collection will be
found.
properties [optional]
is an object that defines, which properties of the
comments shall be returned.
The default properties are as follows:
{
_id: true,
author: true,
website: true,
created: true,
message: true
}
options [optional]
is an object that defines additional options according
to section "Query options" like sorting or
paging.
The default options are as follows:
{
sort: "created" // sort results by date of creation in ascending order
}
received
is a callback function that takes two arguments
(error, results)
. error
is an Error
object, when an error occurred,
otherwise it is null
. results
is a cursor to the result set of the
query. Look at section "Cursors" for more
information on how to use them.
comments.count(res, counted)
Counts the comments of a resource or the complete collection.
res
is a string defining the resource that contains the comments that you
are looking for. If res
is null
, all comments in the collection will be
counted.counted
is a callback function that takes two arguments (error, count)
.
error
is an Error
object, when an error occurred, otherwise it is
null
. count
is the number of comments for the resource or in the
collection.comments.close(done)
Closes the connection to the database.
done
is a callback function.comments.getCommentsJSON(res, resp, received)
Writes a JSON object including the requested comments to a HTTP ServerResponse
object.
res
is a string defining the resource that contains the comments that you
are looking for. If res
is null
, all comments in the collection will be
returned.resp
is a http.ServerResponse
object. (Please refer
to the version of Node.js that you are using.)received
is a callback function that takes one argument (error)
. error
is an Error
object, when an error occurred, otherwise it is null
.comments.parseCommentPOST(res, req, parsed)
Reads a url encoded string from a HTTP ServerRequest
object.
res
is a string defining the resource where the comment should be saved.req
is a http.ServerRequest
object. (Please refer to
the version of Node.js that you are using.)parsed
is a callback function that takes two arguments (error, comment)
.
error
is an Error
object, when an error occurred, otherwise it is
null
. comment
is the parsed comment object.comments.setCommentJSON(res, comment, resp, saved)
Saves or updates a comment for the defined resource and writes the corresponding
HTTP status code to the given ServerResponse
object.
res
is a string defining the resource where the comment should be saved.comment
is an object that defines a comment.resp
is a http.ServerResponse
object. (Please refer
to the version of Node.js that you are using.)saved
is a callback function that takes one argument (error)
. error
is
an Error
object, when an error occurred, otherwise it is null
.You need a running MongoDB before you can use Comments. On Debian
apt-get install mongodb
Then install Comments with npm.
npm install -g Comments
For examples, look at the tests.
If you encounter any bugs or issues, feel free to open an issue at github.
This package is licensed under a MIT license.
FAQs
utilities for building a comment server for your website
We found that Comments demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.