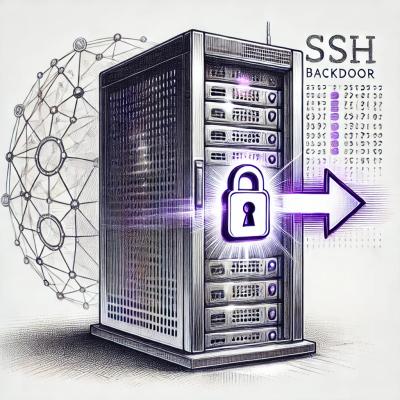
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
abaabil.combobox.acc
Advanced tools
The ComboBox
component is a versatile and customizable dropdown selection component built with React. It supports different variants, sizes, icons, and states, making it suitable for a wide range of use cases.
The ComboBox
component is a customizable dropdown selection input that combines a button with a list of options. This documentation provides instructions on how to use the ComboBox
component in your React projects. The ComboBox
component is a part of the abaabil.combobox
library.
You can import the ComboBox
component from the abaabil
package or directly from the abaabil.combobox
package.
import { ComboBox } from 'abaabil';
// or
import ComboBox from 'abaabil.combobox';
The ComboBox
component can be used in your React projects to create dropdown selection inputs with various properties. You can customize the ComboBox by setting its properties such as options
, onChange
, placeholder
, and more.
import React, { useState } from 'react';
import ComboBox from 'abaabil.combobox';
const options = [
{ key: 'apple', label: 'Apple', icon: 'apple' },
{ key: 'cherry', label: 'Cherry', icon: 'cherry' },
{ key: 'lemon', label: 'Lemon', icon: 'lemon' },
{ key: 'carrot', label: 'Carrot', icon: 'carrot' },
{ key: 'mushroom', label: 'Mushroom', icon: 'mushroom' },
];
const App = () => {
const [selectedOption, setSelectedOption] = useState(null);
const handleChange = (option) => {
setSelectedOption(option);
console.log('Selected option:', option);
};
return (
<div>
<ComboBox
options={options}
placeholder="Select an option"
onChange={handleChange}
variant="primary"
size="df"
/>
</div>
);
};
export default App;
A simple ComboBox with text-only options:
import React, { useState } from 'react';
import ComboBox from 'abaabil.combobox';
const options = [
{ key: 'apple', label: 'Apple' },
{ key: 'banana', label: 'Banana' },
{ key: 'cherry', label: 'Cherry' },
{ key: 'date', label: 'Date' },
{ key: 'elderberry', label: 'Elderberry' },
];
const ComboBoxBasicExample = () => {
const [selectedOption, setSelectedOption] = useState(null);
const handleChange = (option) => {
setSelectedOption(option);
console.log('Selected option:', option);
};
return (
<ComboBox
options={options}
placeholder="Select a fruit"
onChange={handleChange}
/>
);
};
export default ComboBoxBasicExample;
ComboBox that takes up the full width of its container:
import React, { useState } from 'react';
import ComboBox from 'abaabil.combobox';
const options = [
{ key: 'apple', label: 'Apple', icon: 'apple' },
{ key: 'cherry', label: 'Cherry', icon: 'cherry' },
{ key: 'lemon', label: 'Lemon', icon: 'lemon' },
{ key: 'carrot', label: 'Carrot', icon: 'carrot' },
{ key: 'mushroom', label: 'Mushroom', icon: 'mushroom' },
];
const ComboBoxWithFullWidthExample = () => {
const [selectedOption, setSelectedOption] = useState(null);
const handleChange = (option) => {
setSelectedOption(option);
console.log('Selected option:', option);
};
return (
<ComboBox
icon="photo"
actionIcon="chevron-down"
options={options}
onChange={handleChange}
placeholder="Select a fruit or vegetable"
fullWidth
/>
);
};
export default ComboBoxWithFullWidthExample;
ComboBox with icons for options and custom button icons:
import React, { useState } from 'react';
import ComboBox from 'abaabil.combobox';
const options = [
{ key: 'apple', label: 'Apple', icon: 'apple' },
{ key: 'cherry', label: 'Cherry', icon: 'cherry' },
{ key: 'lemon', label: 'Lemon', icon: 'lemon' },
{ key: 'carrot', label: 'Carrot', icon: 'carrot' },
{ key: 'mushroom', label: 'Mushroom', icon: 'mushroom' },
];
const ComboBoxWithIconsExample = () => {
const [selectedOption, setSelectedOption] = useState(null);
const handleChange = (option) => {
setSelectedOption(option);
console.log('Selected option:', option);
};
return (
<ComboBox
icon="photo"
actionIcon="chevron-down"
options={options}
onChange={handleChange}
placeholder="Select a fruit or vegetable"
fullWidth
/>
);
};
export default ComboBoxWithIconsExample;
Different color variants of the ComboBox:
import React, { useState } from 'react';
import ComboBox from 'abaabil.combobox';
const options = [
{ key: 'option1', label: 'Option 1' },
{ key: 'option2', label: 'Option 2' },
{ key: 'option3', label: 'Option 3' },
];
const ComboBoxVariantsExample = () => {
const [selectedOption, setSelectedOption] = useState(null);
const handleChange = (option) => {
setSelectedOption(option);
console.log('Selected option:', option);
};
return (
<div className="space-y-4">
<ComboBox
variant="primary"
options={options}
onChange={handleChange}
placeholder="Primary variant"
/>
<ComboBox
variant="secondary"
options={options}
onChange={handleChange}
placeholder="Secondary variant"
/>
<ComboBox
variant="tertiary"
options={options}
onChange={handleChange}
placeholder="Tertiary variant"
/>
<ComboBox
variant="success"
options={options}
onChange={handleChange}
placeholder="Success variant"
/>
<ComboBox
variant="error"
options={options}
onChange={handleChange}
placeholder="Error variant"
/>
</div>
);
};
export default ComboBoxVariantsExample;
ComboBox in different sizes:
import React, { useState } from 'react';
import ComboBox from 'abaabil.combobox';
const options = [
{ key: 'small', label: 'Small' },
{ key: 'medium', label: 'Medium' },
{ key: 'large', label: 'Large' },
];
const ComboBoxSizesExample = () => {
const [selectedOption, setSelectedOption] = useState(null);
const handleChange = (option) => {
setSelectedOption(option);
console.log('Selected option:', option);
};
return (
<div className="space-y-4">
<ComboBox
size="cp"
options={options}
onChange={handleChange}
placeholder="Small size"
/>
<ComboBox
size="df"
options={options}
onChange={handleChange}
placeholder="Base size"
/>
<ComboBox
size="sp"
options={options}
onChange={handleChange}
placeholder="Large size"
/>
</div>
);
};
export default ComboBoxSizesExample;
Different style options for the ComboBox:
import React, { useState } from 'react';
import ComboBox from 'abaabil.combobox';
const options = [
{ key: 'option1', label: 'Option 1' },
{ key: 'option2', label: 'Option 2' },
{ key: 'option3', label: 'Option 3' },
];
const ComboBoxStylesExample = () => {
const [selectedOption, setSelectedOption] = useState(null);
const handleChange = (option) => {
setSelectedOption(option);
console.log('Selected option:', option);
};
return (
<div className="space-y-4">
<div>
<div>Default style</div>
<ComboBox
options={options}
onChange={handleChange}
placeholder="Default style"
/>
</div>
<div>
<div>Rounded style</div>
<ComboBox
icon="dots-vertical"
options={options}
onChange={handleChange}
rounded
/>
</div>
<div>
<div>Outlined style</div>
<ComboBox
options={options}
onChange={handleChange}
placeholder="Outlined style"
outlined
/>
</div>
<div>
<div>Rounded and outlined</div>
<ComboBox
icon="dots-vertical"
options={options}
onChange={handleChange}
rounded
outlined
/>
</div>
</div>
);
};
export default ComboBoxStylesExample;
Prop | Type | Default | Description |
---|---|---|---|
icon | string | null | Icon to display in the button. Uses the Icon component. |
actionIcon | string | null | Icon to display on the right side of the button. Uses the Icon component. |
size | string | df | The size of the button. Can be cp , df , or sp . |
options | array | [] | Array of options to display in the dropdown. Each option should be an object with key , label , and optionally icon . |
variant | string | primary | The button variant. Can be primary , secondary , tertiary , success , or error . |
onChange | function | null | Callback function to handle selection changes. |
placeholder | string | '' | Placeholder text to display in the button when no option is selected. |
className | string | '' | Additional class names to apply to the button. |
fullWidth | boolean | false | Whether the button should take the full width of its container. |
rounded | boolean | false | Whether the button should have rounded corners. |
outlined | boolean | false | Whether the button should be outlined. |
The ComboBox component is designed to be fully accessible, following the W3C WAI-ARIA Authoring Practices 1.1 guidelines for combobox components. It includes the following accessibility features:
By adhering to these accessibility standards, the ComboBox component ensures a consistent and inclusive user experience for all users, regardless of their abilities or the assistive technologies they may be using.
import React, { useState } from 'react';
import ComboBox from 'abaabil.combobox';
const options = [
{ key: '1', label: 'Option 1', icon: 'icon1' },
{ key: '2', label: 'Option 2', icon: 'icon2' },
{ key: '3', label: 'Option 3', icon: 'icon3' },
];
const Example = () => {
const [selectedOption, setSelectedOption] = useState(null);
const handleChange = (option) => {
setSelectedOption(option);
console.log('Selected option:', option);
};
return (
<div>
<ComboBox
options={options}
placeholder="Select an option"
onChange={handleChange}
variant="primary"
size="df"
/>
</div>
);
};
export default Example;
This example demonstrates various ways to use the ComboBox
component, showcasing different variants, sizes, and states.
FAQs
Unknown package
The npm package abaabil.combobox.acc receives a total of 0 weekly downloads. As such, abaabil.combobox.acc popularity was classified as not popular.
We found that abaabil.combobox.acc demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.