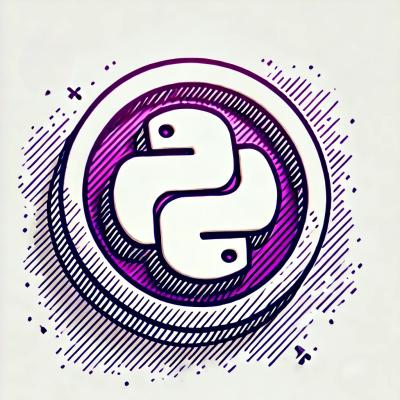
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Functions to create, process and test objects.
npm install adam
component install gamtiq/adam
jam install adam
bower install adam
spm install adam
Use dist/adam.js
or dist/adam.min.js
(minified version).
var adam = require("adam");
require(["adam"], function(adam) {
...
});
define(["path/to/dist/adam.js"], function(adam) {
...
});
<!-- Use bower_components/adam/dist/adam.js if the library was installed by Bower -->
<script type="text/javascript" src="path/to/dist/adam.js"></script>
<script type="text/javascript">
// adam is available via adam field of window object
...
</script>
function inc(data) {
return ++data.value;
}
var s1 = Symbol("s1"),
s2 = Symbol("s2"),
proto = {a: 1},
obj2 = Object.create(proto),
obj;
proto[s1] = "s1";
obj2.b = 2;
obj2[s2] = null;
obj2.c = "str";
obj2.d = 0;
adam.getPropertySymbols(obj2); // [s2, s1]
adam.getFields(obj2); // ["b", "c", "d", s2, "a", s1]
adam.getFields(obj2, {filter: ["string", "false"], filterConnect: "or"}); // ["c", "d", s2, s1]
obj = {a: 1, b: 2, c: 3, d: 4, e: 5};
adam.getClass([8]); // "Array"
adam.getType(null); // "null"
adam.isKindOf(17, "integer"); // true
adam.isKindOf(NaN, "!number"); // true
adam.checkField(obj, "c", ["positive", "odd"]); // true
adam.checkField(obj, "b", ["real", /^7/], {filterConnect: "or"}); // false
adam.getFreeField({a5: 5, a2: 2, a7: 7, a3: 3}, {prefix: "a", startNum: 2}); // "a4"
adam.getSize(obj); // 5
adam.getSize(obj, {filter: "even"}); // 2
adam.isSizeMore(obj, 5); // false
adam.isEmpty({}); // true
adam.getFields(obj); // ["a", "b", "c", "d", "e"]
adam.getFields(obj, {filter: function(value) {return value < 4;}}); // ["a", "b", "c"]
adam.getFields(obj, {filter: {field: /^[d-h]/}}); // ["d", "e"]
adam.getValues(obj); // [1, 2, 3, 4, 5]
adam.getValues(obj, {filter: {field: /a|c/}}); // [1, 3]
adam.getValueKey(obj, 3); // "c"
adam.fromArray([{id: "a", value: 11}, {id: "b", value: 7}, {id: "c", value: 10}], "id"); // {a: {id: "a", value: 11}, b: {id: "b", value: 7}, c: {id: "c", value: 10}}
adam.split(obj, ["a", "d"]); // [{a: 1, d: 4}, {b: 2, c: 3, e: 5}]
adam.split(obj, null, {filter: "odd"}); // [{a: 1, c: 3, e: 5}, {b: 2, d: 4}]
adam.split(obj, null, {filter: ["even", /3/], filterConnect: "or"}); // [{b: 2, c: 3, d: 4}, {a: 1, e: 5}]
adam.remove({a: 1, b: "2", c: 3}, "string"); // {a: 1, c: 3}
adam.remove([1, 2, 3, 4, 5], "even"); // [1, 3, 5]
adam.empty({x: -1, y: 9}); // {}
adam.reverse({a: "x", b: "files"}); // {x: "a", files: "b"}
adam.reverse("eval"); // "lave"
adam.transform("7.381", "string"); // 7
adam.copy(obj, {b: "no", z: "a"}); // {a: 1, b: 2, c: 3, d: 4, e: 5, z: "a"}
adam.copy(obj, {b: "no", z: "a"}, {filter: "odd"}); // {a: 1, b: "no", c: 3, e: 5, z: "a"}
adam.copy(obj, {b: "no", z: "a"}, {filter: "even", transform: inc}); // {b: 3, d: 5, z: "a"}
adam.change({a: 1, b: 2, c: 3}, "reverse"); // {a: -1, b: -2, c: -3}
adam.change({a: 10, b: 28, c: -3, d: null, e: "zero = 0"}, "empty", {filter: /0/}); // {a: 0, b: 28, c: -3, d: null, e: ""}
adam.change([1, 2, 3, 4, 5], "reverse", {filter: "even"}); // [1, -2, 3, -4, 5]
adam.map(obj, "reverse", {filter: "odd"}); // {a: -1, c: -3, e: -5}
adam.map(["1", "2", "3"], "number"); // [1, 2, 3]
See test/adam.js
for additional examples.
Change all or filtered fields of object, applying specified action/transformation.
Check whether the field of given object corresponds to specified condition(s) or filter(s).
Copy all or filtered fields from source object into target object, applying specified transformation if necessary.
Empty the given value.
Create object (map) from list of objects.
Return class of given value (namely value of internal property [[Class]]
).
Return list of all or filtered fields of specified object.
Return name of first free (absent) field of specified object, that conforms to the following pattern: <prefix><number>
Return list of all symbol property keys for given object including keys from prototype chain.
This function is defined only when Object.getOwnPropertySymbols
is available in JS engine.
Otherwise the value of getPropertySymbols
field is undefined
.
Return number of all or filtered fields of specified object.
Return type of given value.
Return the name of field (or list of names) having the specified value in the given object.
Return list of all or filtered field values of specified object.
Check whether given value is an empty value i.e. null
, undefined
, 0
, empty object, empty array or empty string.
Check whether given value has (or does not have) specified kind (type or class).
Check whether number of all or filtered fields of specified object is more than the given value.
Create new object containing all or filtered fields of the source object/array, applying specified action/transformation for field values.
Remove filtered fields/elements from specified object/array.
Reverse or negate the given value.
Divide given object into 2 parts: the first part includes specified fields, the second part includes all other fields.
Transform the given value applying the specified operation.
See doc
folder for details.
In lieu of a formal styleguide, take care to maintain the existing coding style. Add unit tests for any new or changed functionality. Lint and test your code using Grunt.
Copyright (c) 2014-2016 Denis Sikuler
Licensed under the MIT license.
FAQs
Functions to create, process and test objects, maps, arrays, sets
We found that adam demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.