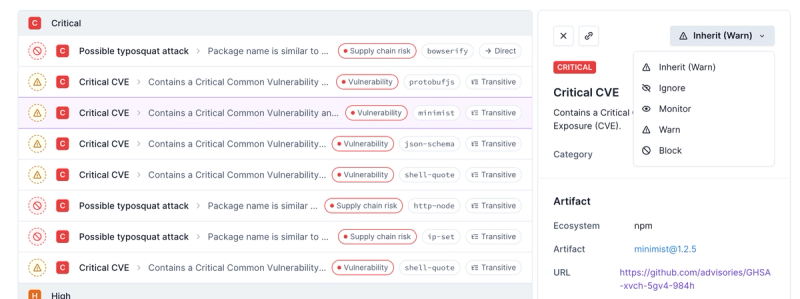
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
agora-rte-extension
Advanced tools
Readme
An extension system for Agora Web SDK NG.
npm install --save agora-rte-extension
when using Yarn
:
yarn add agora-rte-extension
VideoProcessor
or AudioProcessor
Depend on your media type, which is either video or audio, you should extend VideoProcessor
or AudioProcessor
accordingly.
import {VideoProcessor, AudioProcessor} from "agora-rte-extension";
//for video processor
class YourVideoProcessor extends VideoProcessor {
}
//for audio processor
class YourAudioProcessor extends AudioProcessor {
}
For Video Processor, there are 1 property, 4 methods could be implemented:
import {VideoProcessor, AudioProcessor} from "agora-rte-extension";
import type {IProcessorContext} from "agora-rte-extension";
class YourVideoProcessor extends VideoProcessor {
/**
* processor name
* */
public name = "YourVideoProcessor"
/**
* will be called when AgoraRTC.LocalTrack was connected to the pipedline
* */
onPiped(context: IProcessorContext) {
}
/**
* will be called when AgoraRTC.LocalTrack was disconnected from the pipedline,
* or the current/previous processor was disconnected from the pipeline.
* */
onUnpiped() {
}
/**
* will be called when previous processor generated output MediaStreamTrack
* */
onTrack(track: MediaStreamTrack, context: IProcessorContext) {
}
/**
* will be called when enable/disable methods on Processor was called
* */
onEnableChange(enabled: boolean) {
}
}
When the video is being processed and is about to generate output, you can call output
method on Processor
:
import {IProcessorContext} from "agora-rte-extension";
class YourVideoProcessor extends VideoProcessor {
onTrack(track: MediaStreamTrack, context: IProcessorContext) {
//...processing
const processedTrack = this.process(track);
//output processed MediaStreamTrack
this.output(processedTrack, context);
}
}
For Audio Processor, there are 1 property, 5 methods could be implemented:
import {VideoProcessor, AudioProcessor} from "agora-rte-extension";
import type {IAudioProcessorContext} from "agora-rte-extension";
class YourVideoProcessor extends VideoProcessor {
/**
* processor name
* */
public name = "YourVideoProcessor"
/**
* will be called when AgoraRTC.LocalTrack was connected to the pipedline
* */
onPiped(context: IAudioProcessorContext) {
}
/**
* will be called when AgoraRTC.LocalTrack was disconnected from the pipedline,
* or the current/previous processor was disconnected from the pipeline.
* */
onUnpiped() {
}
/**
* will be called when previous processor generated output MediaStreamTrack
* */
onTrack(track: MediaStreamTrack, context: IAudioProcessorContext) {
}
/**
* will be called when previous processor generated output AudioNode
* */
onNode(node: AudioNode, context: IAudioProcessorContext) {
}
/**
* will be called when enable/disable methods on Processor was called
* */
onEnableChange(enabled: boolean) {
}
}
When the audio is being processed and is about to generate output, you can call output
method on Processor
:
import {IProcessorContext} from "./index";
class YourAudioProcessor extends AudioProcessor {
onNode(node: AudioNode, context: IAudioProcessorContext) {
//...processing
const processedAudioNode = this.process(node);
this.output(processedAudioNode, context);
}
}
a.Choose
betweenExtension
orAudioExtension
Depend
on
your
media
type, which
is
either
video
or
audio, you
should
extendExtension
orAudioExtension
accordingly.
import {Extension, AudioExtension} from "agora-rte-extension";
//for video extension
class YourVideoExtension extends Extension<YourVideoProcessor> {
}
//for audio extension
class YourAudioExtension extends AudioExtension<YourAudioProcessor> {
}
_createProcessor
methodfor Extension to create VideoProcessor or AudioProcessor, you should implement _createProcessor
based on Extension
or AudioExtension
:
import {Extension, AudioExtension} from 'agora-rte-extension'
class YourVideoExtension extends Extension<YourVideoProcessor> {
protected _createprocessor(){
return new YourVideoProcessor();
}
}
class YourAudioExtension extends AudioExtension<YourAudioProcessor> {
protected _createprocessor(){
return new YourAudioProcessor();
}
}
FAQs
Agora RTE Extension
The npm package agora-rte-extension receives a total of 24,062 weekly downloads. As such, agora-rte-extension popularity was classified as popular.
We found that agora-rte-extension demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.