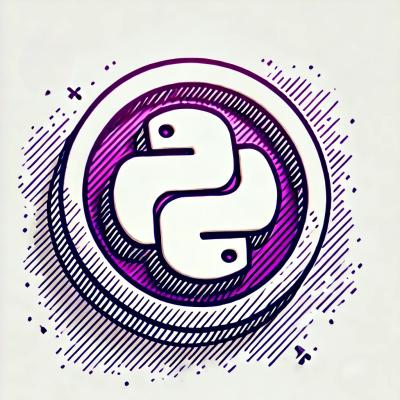
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
airwallex-payment-elements
Advanced tools
This universal component is a lightweight wrapper of airwallex checkout widget, which allow merchant site to integrate with airwallex checkout flow.
This universal component is a lightweight wrapper of airwallex checkout widget, which allow merchant site to integrate with airwallex checkout flow.
By adopt render props pattern, all interaction with the component base on component props, the props interface give merchant fully control on the checkout flow without violation the PCI compliance, also provide maximum flexibility on look and feels customize.
The component provide universal support for all FE framework, no matter Angular / React / VUE. Take advantages of Web Component, more details could be found W3C Web Component.
https://airwallex-checkout.awx.im
yarn add airwallex-payment-elements
OR with NPM:
npm add airwallex-payment-elements
Or using UMD build:
WIP, will provide when CDN is ready
import 'airwallex-payment-elements';
Reference: Airwallex Web APP
Reference: Airwallex API Doc
Reference: Airwallex API Doc
<airwallex-element
gatewayBaseurl="https://airwallex-checkout.awx.im:3000"
intentId={intentId}
merchantId={merchantId}
authentication={authentication}
customerId={customerId}
countryOrRegion={countryOrRegionCode}
currency={currency}
theme={theme}
successUrl="https://www.yourwebsite.com/success"
cancelUrl="https://www.yourwebsite.com/cancel"
errorUrl="https://www.yourwebsite.com/error"
/>
<airwallex-element />
is a web component customer element, which interacting with Airwallex UI FE to render the payment methods
gatewayBaseurl
is the domain url to loading airwallex checkout assets (html, javascript, css, fonts), it's also the server url for user interaction like submit checkout form.
Production: https://checkout.airwallex.com
Demo: https://demo-checkout.airwallex.com
Staging: https://staging-checkout.airwallex.com
Dev: https://airwallex-checkout.awx.im:3000
intentId
is payment intent id create by calling /api/v1/payment_intents/create
merchantId
is unique identification of merchant, create when you sign up as Airwallex merchant, could be found on merchant dashboard(WIP)
authentication
is your secret key get from your dashboard(WIP), Airwallex payment api will use it to verify your true identity
customerId
(Optional) is using for known customer, whom we allow reuse token for fast payment, can be create by calling /api/v1/customer/create
, for anonymous user just ignore this prop.
countryOrRegionCode
is two characters code Country Code
currency
is three characters code Currency Code
theme
is looks and feels config STRING prop which give merchant interface to customize the checkout UI, below are support values:
interface ITheme {
variant: 'outlined' | 'filled' | 'standard' | 'bootstrap';
palette: {
primary: Color; // https://material-ui.com/customization/color/#color
secondary: Color; // https://material-ui.com/customization/color/#color
error: Color; // https://material-ui.com/customization/color/#color
background: {
paper: string;
default: string;
};
text: {
primary: string;
secondary: string;
disabled: string;
hint: string;
};
};
typography: {
htmlFontSize: number;
fontSize: number;
fontFamily: string;
fontWeightLight: number;
fontWeightRegular: number;
fontWeightMedium: number;
fontWeightBold: number;
button: {
textTransform: string;
fontSize: number;
fontWeight: number;
lineHeight: number;
};
};
shape: {
borderRadius: number;
};
zIndex: {
mobileStepper: number;
appBar: number;
drawer: number;
modal: number;
snackbar: number;
tooltip: number;
};
}
Default themes value as below:
{
variant: 'outlined',
palette: {
primary: orange,
secondary: grey,
error: red,
background: {
paper: '#fff',
default: '#fafafa'
},
text: {
primary: '#2A2A2A',
secondary: '#54545E',
disabled: '#A9A9AE',
hint: '#d1d2d5'
}
},
typography: {
htmlFontSize: 16,
fontSize: 14,
fontFamily: 'AxFFDIN'
fontWeightLight: 300,
fontWeightRegular: 400,
fontWeightMedium: 500,
fontWeightBold: 700,
button: {
textTransform: 'unset',
fontSize: 16,
fontWeight: 500,
lineHeight: 2.15
}
},
shape: {
borderRadius: 4
},
zIndex: {
mobileStepper: 1000,
appBar: 1100,
drawer: 1200,
modal: 1300,
snackbar: 1400,
tooltip: 1500
}
}
Before passing into the theme props, you must stringify
(i.e. JSON.stringify()
) it as string.
successUrl / cancelUrl / errorUrl
Optional urls for checkout redirection, any valid url works, the checkout component will append status to the url and do the redirection upon user checkout interaction.
EVENT
IS NOT PASSING BY PROPS, WILL TRIGGER BY CHECKOUT FLOW.
To listen on checkout event, merchant page need to add event listener, below is an example:
const airwallexElement: any = document.querySelector('airwallex-element');
airwallexElement.addEventListener('airwallex-checkout', ({ detail }: any) => {
// Merchant business flow
});
WIP
Checkout UI can also listen on merchant event and take on actions.
FAQs
[](https://www.npmjs.org/package/airwallex-payment-elements) [](https://git
The npm package airwallex-payment-elements receives a total of 4,732 weekly downloads. As such, airwallex-payment-elements popularity was classified as popular.
We found that airwallex-payment-elements demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.