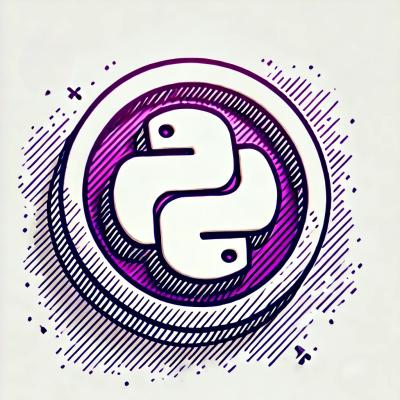
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
airwallex-payment-elements
Advanced tools
[](https://github.com/semantic-release/semantic-release)
This universal library is a lightweight javascript SDK, which allow merchant site to convenient integrate with airwallex checkout flow
From the merchant perspective, The Hosted Payment Page(HPP) / Drop-in / Elements integration happen after shopper decide to checkout an order on merchant's website, Airwallex javascript SDK help merchant accept payments on merchant website, the whole user experience include create an PaymentIntent entity to tacking the payment request, which link to merchant’s order entity, Airwallex using a paymentAttempt entity to collect payment methods details from a shopper, and link back to the PaymentIntent entity, which tracking the state change all the way through user interactions, you can checkout the PaymentIntent section for more details
With the API integration from merchant server, after PaymentIntent is successfully created, the response of the API call will return a unique ID for the PaymentIntent, and a client_secret which act as a single source of truth cross over the shopping experience, each time user's interaction trigger a service call Airwallex client API will validate against the client_secret to secure the whole payment flow
So long story short, the merchant website checkout start with PaymentIntent and client_secret
Below are the step by step guide to use this library
yarn add airwallex-payment-elements
OR with NPM:
npm install airwallex-payment-elements
Or using UMD build from your client HTML:
<script src="https://checkout.airwallex.com/assets/bundle.min.js"></script>
Airwallex.init(client_secret: string, gateway_url: string, options?: InitOptions);
client_secret
- [REQUIRED], can find it from response when create PaymentIntent
gateway_url
- [REQUIRED], is the domain url to loading airwallex checkout assets (html, javascript, css, fonts), it's also the server url for user interaction like submit checkout form.
Production: https://checkout.airwallex.com
Demo: https://checkout-demo.airwallex.com
Staging: https://checkout-staging.airwallex.com
options
is useful to customize looks and feel of the checkout form when you are adopt Drop-in / Elements integration
interface FontOptions {
family?: string;
src?: string;
weight?: string;
}
export interface InitOptions {
locale?: string;
font?: FontOptions;
}
The HPP provide the most convenient way to integrate
export interface HostPaymentPage {
host: string; // checkout-{env}.airwallex.com | checkout.airwallex.com
client_secret: string;
theme?: Style;
customerId?: string;
component?: 'default' | 'cards' | 'wechatpay';
successUrl?: string;
failUrl?: string;
cancelUrl?: string;
encodeLogoUrl?: string;
}
i.e Using default method and looks & feel
airwallex.redirectToCheckout({
host: 'checkout.airwallex.com',
client_secret: 'client_secret',
}
The primary integration path through this library is with Elements, which enables merchant to collect sensitive payment information(PCI) using customizable UI elements. this library also provides a single interface for Payment Request API
With this library, you can also tokenize sensitive information, and handle 3D Secure authentication
Airwallex element is a set of prebuilt UI components, like inputs and buttons, for building your checkout flow. It’s available as a feature of this library. this library handle checkout confirm within an Element without ever having it touch your server
Elements includes features like:
Formatting card information automatically as it’s entered
Customize placeholders to fit your UX design
Using responsive design to fit the width of your customer’s screen or mobile device
Customizing the styling to match the look and feel of your checkout flow
When you select dropIn element type you are actually adopt Drop-in integration
With Drop-in integration, those prebuilt UI components has been composed into a payment form with all available payment methods, you can put it directly into you html page, and integrate with the rest of your own components to render your checkout page.
With cardNumber | expiry | cvc | paymentRequestButton | wechat elements, they are just building blocks:
You can arrange with any order you want
Show and hide anyone of them at any moment you want
Customize the look and feels as you want
Listen on the events and interact with shoppers when they click or typing in those elements
export declare const createElement: (type: 'cardNumber' | 'expiry' | 'cvc' | 'paymentRequestButton' | 'card' | 'wechat' | 'dropIn', options?: ElementOptions | undefined) => ElementBase | null;
export interface Intent {
id: string;
latest_payment_attempt: {
id: string;
};
}
export interface Style {
variant: 'outlined' | 'filled' | 'standard' | 'bootstrap';
hidden?: boolean;
backgroundColor?: string;
color?: string;
fontSize?: string;
fontSmoothing?: string;
fontStyle?: string;
fontVariant?: string;
fontWeight?: string;
lineHeight?: string;
letterSpacing?: string;
textAlign?: string;
padding?: number;
textDecoration?: string;
textShadow?: string;
textTransform?: string;
}
export interface ElementOptions {
style?: Style;
placeholder?: string;
cvcLength?: number;
origin?: string;
intent?: Intent;
}
Airwallex.createElement(type, options);
i.e Mount element to div, you can add card html div element in your html template, and call below method will mount them:
<div id='card'></div>
const cardElement = Airwallex.createElement('card');
cardElement.mount('card');
export declare const confirmPaymentIntent: (data: PaymentMethod) => Promise<boolean | Intent>;
i.e Confirm using card element
const payload = {
element: Airwallex.getElement('card'),
intent,
payment_method: {
billing
}
};
try {
const res = await Airwallex.confirmPaymentIntent(payload);
const { confirmResult } = res || {};
const { id, status } = confirmResult || {};
/*
Handle success with below parameters
...({
id,
email,
status
})
*/
} catch (err) {
const { reason } = err;
const { error: { code, message } } = reason || {};
/*
... Handle error with checkout
*/
}
export interface ElementEvent {
type: 'cardNumber' | 'expiry' | 'cvc' | 'paymentRequestButton' | 'card' | 'wechat' | 'dropIn';
code: 'onReady' | 'onSubmit' | 'onSuccess' | 'onError' | 'onCancel' | 'onFocus' | 'onBlur' | 'onChange' | 'onClick';
complete: boolean;
empty: boolean;
brand: string;
error?: {
msg: string;
code: string;
value?: string;
};
}
i.e Listen to event when user typing on the input
window.addEventListener('onChange', (event: ElementEvent) => {
/*
... Handle event
*/
}
FAQs
[](https://www.npmjs.org/package/airwallex-payment-elements) [](https://git
The npm package airwallex-payment-elements receives a total of 4,732 weekly downloads. As such, airwallex-payment-elements popularity was classified as popular.
We found that airwallex-payment-elements demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.