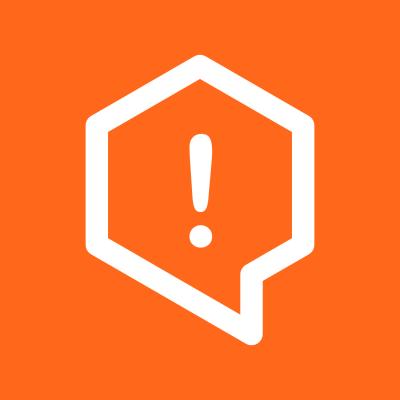
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
A library of async iterator extensions for JavaScript
npm install aix # or yarn install aix
Async iterators are a useful way to handle asynchronous streams. This library adds a number of utility methods similar to those found in lodash, underscore or Ramda.
fromEvents
turns DOM events into an iterable.
import { fromEvents } from "aix/fromEvents";
const clicks = fromEvents(document, 'click');
for await (const click of clicks) {
console.log('a button was clicked');
}
DeferredIterable
makes it easy to turn stream of events into an iterable. The code below
is essentially how fromEvents
was implemented.
import { DeferredIterable } from "aix/deferredIterable";
const deferredIterable = new DeferredIterable();
// set up a callback that calls value on the deferredIterable
const callback = value => deferredIterable.value(value);
// attach the callback to the click event
document.addEventListener('click', callback);
// remove the callback when / if the iterable stops
deferredIterable.finally(() => document.removeEventListener('click', deferredIterable.value));
// go through all the click events
for await (const click of deferredIterable.iterable) {
console.log('a button was clicked');
}
FAQs
A library of async iterator extensions for JavaScript
The npm package aix receives a total of 0 weekly downloads. As such, aix popularity was classified as not popular.
We found that aix demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.